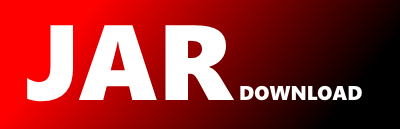
io.divide.client.data.ObjectManager Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2014 Divide.io
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package io.divide.client.data;
import com.google.inject.Inject;
import io.divide.client.BackendObject;
import io.divide.client.BackendUser;
import io.divide.shared.server.DAO;
import io.divide.shared.transitory.query.Query;
import io.divide.shared.transitory.query.SelectOperation;
import rx.Observable;
import java.util.Arrays;
import java.util.Collection;
public class ObjectManager {
@Inject DataManager dataManager;
@Inject DAO localStorage;
public ObjectManager(){ }
public DAO local(){
return localStorage;
}
public RemoteStorage remote(){
return remote;
}
private RemoteStorage remote = new RemoteStorage();
public class RemoteStorage{
private RemoteStorage(){};
private Collection assignUser(BackendUser user, B... objects) throws NotLoggedInException {
return assignUser(user, Arrays.asList(objects));
}
private Collection assignUser(BackendUser user,Collection objects) throws NotLoggedInException{
for(BackendObject o : objects){
if(o.getOwnerId() == null){
o.setOwnerId(user.getOwnerId());
}
}
return objects;
}
public Observable save(B... objects){
try{
BackendUser user;
if((user = BackendUser.getUser()) == null) throw new NotLoggedInException();
return dataManager.send(assignUser(user, objects));
}catch (Exception e){
return Observable.error(e);
}
}
public Observable> load(Class type, String... keys){
return dataManager.get(type,Arrays.asList(keys));
}
public Observable> query(Class type, Query query){
if(query.getSelect() != null){
SelectOperation so = query.getSelect();
if(!so.getType().equals(type))
throw new IllegalStateException(so.getErrorMessage());
}
if (!query.getFrom().equals(Query.safeTable(type))){
throw new IllegalStateException("Can not return a different type then what is queried!\n" +
"Expected: " + query.getFrom() + "\n" +
"Actual: " + Query.safeTable(type));
}
return dataManager.query(type,query);
}
public Observable count(Class type){
return dataManager.count(type);
}
}
public static class NotLoggedInException extends Exception {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy