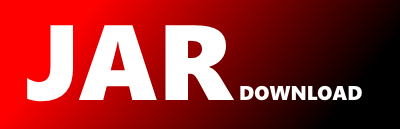
io.dropwizard.bundles.version.VersionServlet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dropwizard-version-bundle Show documentation
Show all versions of dropwizard-version-bundle Show documentation
Dropwizard bundle that easily exposes the version of your application.
package io.dropwizard.bundles.version;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.collect.Maps;
import com.google.common.io.Closeables;
import io.dropwizard.jackson.Jackson;
import java.io.IOException;
import java.util.Map;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import static com.google.common.base.Preconditions.checkNotNull;
/**
* A simple servlet that will return the application version along with the versions of its
* dependencies as a JSON object. For example:
*
* {
* application: "1.0.0-SNAPSHOT",
* dependencies: {
* "com.google.guava:guava": "18.0",
* "io.dropwizard:dropwizard-core": "0.8.1"
* }
* }
*
*
* NOTE: This class intentionally delays asking the provided {@code VersionSupplier} for
* versions until the servlet is called. Because of this, the supplier will be invoked for each
* request to the servlet. The provided implementation of {@code VersionSupplier} should memoize
* its results so that a recalculation doesn't happen on every request.
*/
class VersionServlet extends HttpServlet {
private final VersionSupplier supplier;
private final ObjectMapper objectMapper;
VersionServlet(VersionSupplier supplier, ObjectMapper objectMapper) {
this.supplier = checkNotNull(supplier);
this.objectMapper = checkNotNull(objectMapper);
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException {
String applicationVersion;
try {
applicationVersion = supplier.getApplicationVersion();
} catch (Throwable t) {
resp.sendError(500, "Unable to determine application version.");
return;
}
Map dependencyVersions;
try {
dependencyVersions = supplier.getDependencyVersions();
} catch (Throwable t) {
resp.sendError(500, "Unable to determine dependency versions.");
return;
}
Map response = Maps.newHashMap();
response.put("application", applicationVersion);
response.put("dependencies", dependencyVersions);
// At this point we've collected all of the data we need, we know the result will be a success.
resp.setStatus(200);
resp.setContentType("application/json");
ServletOutputStream out = resp.getOutputStream();
try {
objectMapper.writeValue(out, response);
} finally {
Closeables.close(out, false);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy