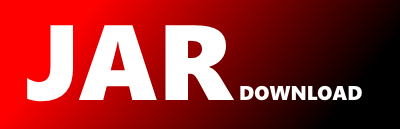
io.dropwizard.bundles.webjars.VersionLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dropwizard-webjars-bundle Show documentation
Show all versions of dropwizard-webjars-bundle Show documentation
Dropwizard bundle to make working with Webjars (http://webjars.org) easier.
package io.dropwizard.bundles.webjars;
import com.google.common.cache.CacheLoader;
import com.google.common.io.Closer;
import com.google.common.io.Resources;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Properties;
/**
* Determines the version of the webjar that's in the classpath for a particular library.
*
* The version of a webjar can be determined by looking at the pom.properties
file
* located at {@code META-INF/maven///pom.properties}.
*
* Where {@code } is the name of the maven group the webjar artifact is part of, and
* {@code } is the name of the library.
*/
class VersionLoader extends CacheLoader {
public static final String NOT_FOUND = "VERSION-NOT-FOUND";
private final Iterable groups;
VersionLoader(Iterable groups) {
this.groups = groups;
}
@Override
public String load(String library) throws Exception {
for (String group : groups) {
String found = tryToLoadFrom("META-INF/maven/%s/%s/pom.properties", group, library);
if (found != null) {
return found;
}
}
return NOT_FOUND;
}
private String tryToLoadFrom(String format, String group, String library) {
String path = String.format(format, group, library);
URL url;
try {
url = Resources.getResource(path);
} catch (IllegalArgumentException e) {
return null;
}
try {
Closer closer = Closer.create();
InputStream in = closer.register(url.openStream());
try {
Properties props = new Properties();
props.load(in);
return props.getProperty("version");
} finally {
closer.close();
}
} catch (IOException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy