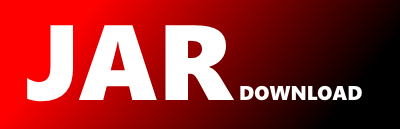
io.dropwizard.logging.json.layout.MapBuilder Maven / Gradle / Ivy
package io.dropwizard.logging.json.layout;
import org.checkerframework.checker.nullness.qual.Nullable;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Supplier;
import static java.util.Objects.requireNonNull;
/**
* Builds a Java map based on the provided configuration and customization.
*/
public class MapBuilder {
private final TimestampFormatter timestampFormatter;
/**
* Custom field name replacements in the format (oldName:newName).
*/
private final Map customFieldNames;
/**
* Additional fields which should be included in the message.
*/
private final Map additionalFields;
private final Map map;
public MapBuilder(TimestampFormatter timestampFormatter, Map customFieldNames,
Map additionalFields, int expectedSize) {
this.timestampFormatter = timestampFormatter;
this.customFieldNames = requireNonNull(customFieldNames);
this.additionalFields = requireNonNull(additionalFields);
this.map = new LinkedHashMap<>(expectedSize);
this.map.put(getFieldName("timestamp"), null); // Insert a null timestamp at first and fix it at build time
}
/**
* Adds the string value to the provided map under the provided field name,
* if it should be included.
*/
public MapBuilder add(String fieldName, boolean include, @Nullable String value) {
if (include && value != null) {
map.put(getFieldName(fieldName), value);
}
return this;
}
/**
* Adds the string value to the provided map under the provided field name,
* if it should be included. The supplier is only invoked if the field is to be included.
*
* @since 2.0
*/
public MapBuilder add(String fieldName, boolean include, Supplier supplier) {
if (include) {
String value = supplier.get();
if (value != null) {
map.put(getFieldName(fieldName), value);
}
}
return this;
}
/**
* Adds the number to the provided map under the provided field name if it's should be included.
*/
public MapBuilder addNumber(String fieldName, boolean include, @Nullable Number number) {
if (include && number != null) {
map.put(getFieldName(fieldName), number);
}
return this;
}
/**
* Adds the number value to the provided map under the provided field name,
* if it should be included. The supplier is only invoked if the field is to be included.
*
* @since 2.0
*/
public MapBuilder addNumber(String fieldName, boolean include, Supplier supplier) {
if (include) {
Number value = supplier.get();
if (value != null) {
map.put(getFieldName(fieldName), value);
}
}
return this;
}
/**
* Adds the map to the provided map under the provided field name if it's should be included.
*/
public MapBuilder add(String fieldName, boolean include, @Nullable Map mapValue) {
if (include && mapValue != null && !mapValue.isEmpty()) {
map.put(getFieldName(fieldName), mapValue);
}
return this;
}
/**
* Adds the map value to the provided map under the provided field name, if it should be
* included. The supplier is only invoked if the field is to be included.
*
* @since 2.0
*/
public MapBuilder addMap(String fieldName, boolean include, Supplier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy