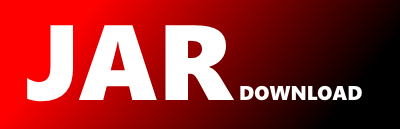
io.dropwizard.logging.TlsSocketAppenderFactory Maven / Gradle / Ivy
package io.dropwizard.logging;
import ch.qos.logback.core.spi.DeferredProcessingAware;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeName;
import org.eclipse.jetty.util.ssl.SslContextFactory;
import javax.annotation.Nullable;
import javax.net.SocketFactory;
import javax.validation.constraints.NotEmpty;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.List;
/**
* An {@link AppenderFactory} implementation which provides an appender that writes events to a TCP socket
* secured by the TLS/SSL protocol on the presentation layer.
*
* Configuration Parameters:
*
*
* Name
* Default
* Description
*
*
* {@code keyStorePath}
* (none)
*
* The path to the Java key store which contains the host certificate and private key.
*
*
*
* {@code keyStorePassword}
* (none)
*
* The password used to access the key store.
*
*
*
* {@code keyStoreType}
* {@code JKS}
*
* The type of key store (usually {@code JKS}, {@code PKCS12}, {@code JCEKS},
* {@code Windows-MY}, or {@code Windows-ROOT}).
*
*
*
* {@code keyStoreProvider}
* (none)
*
* The JCE provider to use to access the key store.
*
*
*
* {@code trustStorePath}
* (none)
*
* The path to the Java key store which contains the CA certificates used to establish
* trust.
*
*
*
* {@code trustStorePassword}
* (none)
* The password used to access the trust store.
*
*
* {@code trustStoreType}
* {@code JKS}
*
* The type of trust store (usually {@code JKS}, {@code PKCS12}, {@code JCEKS},
* {@code Windows-MY}, or {@code Windows-ROOT}).
*
*
*
* {@code trustStoreProvider}
* (none)
*
* The JCE provider to use to access the trust store.
*
*
*
* {@code jceProvider}
* (none)
* The name of the JCE provider to use for cryptographic support.
*
*
* {@code validateCerts}
* false
*
* Whether or not to validate TLS certificates before starting. If enabled, Dropwizard
* will refuse to start with expired or otherwise invalid certificates.
*
*
*
* {@code validatePeers}
* false
*
* Whether or not to validate TLS peer certificates.
*
*
*
* {@code supportedProtocols}
* JVM default
*
* A list of protocols (e.g., {@code SSLv3}, {@code TLSv1}) which are supported. All
* other protocols will be refused.
*
*
*
* {@code excludedProtocols}
* [SSL, SSLv2, SSLv2Hello, SSLv3]
*
* A list of protocols (e.g., {@code SSLv3}, {@code TLSv1}) which are excluded. These
* protocols will be refused.
*
*
*
* {@code supportedCipherSuites}
* JVM default
*
* A list of cipher suites (e.g., {@code TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256}) which
* are supported. All other cipher suites will be refused
*
*
*
* {@code excludedCipherSuites}
* [.*_(MD5|SHA|SHA1)$]
*
* A list of cipher suites (e.g., {@code TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256}) which
* are excluded. These cipher suites will be refused.
*
*
*
*
* For more configuration parameters, see {@link TcpSocketAppenderFactory}.
*
* @see TcpSocketAppenderFactory
*/
@JsonTypeName("tls")
public class TlsSocketAppenderFactory extends TcpSocketAppenderFactory {
@Nullable
private String keyStorePath;
@Nullable
private String keyStorePassword;
@NotEmpty
private String keyStoreType = "JKS";
@Nullable
private String keyStoreProvider;
@Nullable
private String trustStorePath;
@Nullable
private String trustStorePassword;
@NotEmpty
private String trustStoreType = "JKS";
@Nullable
private String trustStoreProvider;
@Nullable
private String jceProvider;
@Nullable
private List supportedProtocols;
@Nullable
private List excludedProtocols;
@Nullable
private List supportedCipherSuites;
@Nullable
private List excludedCipherSuites;
private boolean validateCerts;
private boolean validatePeers;
@JsonProperty
public boolean isValidatePeers() {
return validatePeers;
}
@JsonProperty
public void setValidatePeers(boolean validatePeers) {
this.validatePeers = validatePeers;
}
@JsonProperty
public boolean isValidateCerts() {
return validateCerts;
}
@JsonProperty
public void setValidateCerts(boolean validateCerts) {
this.validateCerts = validateCerts;
}
@JsonProperty
@Nullable
public List getExcludedCipherSuites() {
return excludedCipherSuites;
}
@JsonProperty
public void setExcludedCipherSuites(List excludedCipherSuites) {
this.excludedCipherSuites = excludedCipherSuites;
}
@JsonProperty
@Nullable
public List getSupportedCipherSuites() {
return supportedCipherSuites;
}
@JsonProperty
public void setSupportedCipherSuites(List supportedCipherSuites) {
this.supportedCipherSuites = supportedCipherSuites;
}
@JsonProperty
@Nullable
public List getExcludedProtocols() {
return excludedProtocols;
}
@JsonProperty
public void setExcludedProtocols(List excludedProtocols) {
this.excludedProtocols = excludedProtocols;
}
@JsonProperty
@Nullable
public List getSupportedProtocols() {
return supportedProtocols;
}
@JsonProperty
public void setSupportedProtocols(List supportedProtocols) {
this.supportedProtocols = supportedProtocols;
}
@JsonProperty
@Nullable
public String getTrustStoreProvider() {
return trustStoreProvider;
}
@JsonProperty
public void setTrustStoreProvider(String trustStoreProvider) {
this.trustStoreProvider = trustStoreProvider;
}
@JsonProperty
@Nullable
public String getTrustStoreType() {
return trustStoreType;
}
@JsonProperty
public void setTrustStoreType(String trustStoreType) {
this.trustStoreType = trustStoreType;
}
@JsonProperty
@Nullable
public String getTrustStorePassword() {
return trustStorePassword;
}
@JsonProperty
public void setTrustStorePassword(String trustStorePassword) {
this.trustStorePassword = trustStorePassword;
}
@JsonProperty
@Nullable
public String getTrustStorePath() {
return trustStorePath;
}
@JsonProperty
public void setTrustStorePath(String trustStorePath) {
this.trustStorePath = trustStorePath;
}
@JsonProperty
@Nullable
public String getKeyStoreProvider() {
return keyStoreProvider;
}
@JsonProperty
public void setKeyStoreProvider(String keyStoreProvider) {
this.keyStoreProvider = keyStoreProvider;
}
@JsonProperty
@Nullable
public String getKeyStoreType() {
return keyStoreType;
}
@JsonProperty
public void setKeyStoreType(String keyStoreType) {
this.keyStoreType = keyStoreType;
}
@JsonProperty
@Nullable
public String getKeyStorePassword() {
return keyStorePassword;
}
@JsonProperty
public void setKeyStorePassword(String keyStorePassword) {
this.keyStorePassword = keyStorePassword;
}
@JsonProperty
@Nullable
public String getKeyStorePath() {
return keyStorePath;
}
@JsonProperty
public void setKeyStorePath(String keyStorePath) {
this.keyStorePath = keyStorePath;
}
@JsonProperty
@Nullable
public String getJceProvider() {
return jceProvider;
}
@JsonProperty
public void setJceProvider(String jceProvider) {
this.jceProvider = jceProvider;
}
private SslContextFactory createSslContextFactory() {
SslContextFactory factory = new SslContextFactory.Server();
if (keyStorePath != null) {
factory.setKeyStorePath(keyStorePath);
}
factory.setKeyStoreType(keyStoreType);
if (keyStorePassword != null) {
factory.setKeyStorePassword(keyStorePassword);
}
if (keyStoreProvider != null) {
factory.setKeyStoreProvider(keyStoreProvider);
}
if (trustStorePath != null) {
factory.setTrustStorePath(trustStorePath);
}
if (trustStorePassword != null) {
factory.setTrustStorePassword(trustStorePassword);
}
factory.setTrustStoreType(trustStoreType);
if (trustStoreProvider != null) {
factory.setTrustStoreProvider(trustStoreProvider);
}
factory.setValidateCerts(validateCerts);
factory.setValidatePeerCerts(validatePeers);
if (supportedProtocols != null) {
factory.setIncludeProtocols(supportedProtocols.toArray(new String[0]));
}
if (excludedProtocols != null) {
factory.setExcludeProtocols(excludedProtocols.toArray(new String[0]));
}
if (supportedCipherSuites != null) {
factory.setIncludeCipherSuites(supportedCipherSuites.toArray(new String[0]));
}
if (excludedCipherSuites != null) {
factory.setExcludeCipherSuites(excludedCipherSuites.toArray(new String[0]));
}
if (jceProvider != null) {
factory.setProvider(jceProvider);
}
return factory;
}
@Override
protected SocketFactory socketFactory() {
final SslContextFactory sslContextFactory = createSslContextFactory();
try {
sslContextFactory.start();
} catch (Exception e) {
throw new IllegalStateException("Unable to configure SSLContext", e);
}
// We use an adapter over the `newSslSocket` call of Jetty's `SslContextFactory`, because it provides more
// advanced socket configuration than Java's `SSLSocketFactory`.
return new SocketFactory() {
@Override
public Socket createSocket() throws IOException {
return sslContextFactory.newSslSocket();
}
@Override
public Socket createSocket(String host, int port) throws IOException, UnknownHostException {
return unsupported();
}
@Override
public Socket createSocket(String host, int port, InetAddress localHost, int localPort) throws IOException, UnknownHostException {
return unsupported();
}
@Override
public Socket createSocket(InetAddress host, int port) throws IOException {
return unsupported();
}
@Override
public Socket createSocket(InetAddress address, int port, InetAddress localAddress, int localPort) throws IOException {
return unsupported();
}
private Socket unsupported() {
throw new UnsupportedOperationException("Only createSocket is supported");
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy