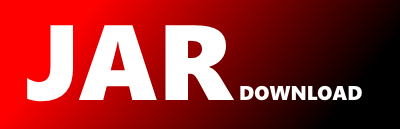
io.druid.server.lookup.cache.polling.OffHeapPollingCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of druid-lookups-cached-single Show documentation
Show all versions of druid-lookups-cached-single Show documentation
Extension to rename Druid dimension values using lookups
/*
*
* Licensed to Metamarkets Group Inc. (Metamarkets) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. Metamarkets licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
* /
*/
package io.druid.server.lookup.cache.polling;
import com.google.common.base.Function;
import com.google.common.base.Predicate;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import org.mapdb.DB;
import org.mapdb.DBMaker;
import org.mapdb.HTreeMap;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import java.util.concurrent.atomic.AtomicBoolean;
public class OffHeapPollingCache implements PollingCache
{
private static final DB DB = DBMaker.newMemoryDirectDB().transactionDisable().closeOnJvmShutdown().make();
private final HTreeMap mapCache;
private final HTreeMap> reverseCache;
private final AtomicBoolean started = new AtomicBoolean(false);
private final String cacheName;
private final String reverseCacheName;
public OffHeapPollingCache(final Iterable> entries)
{
synchronized (started) {
this.cacheName = String.format("cache-%s", UUID.randomUUID());
this.reverseCacheName = String.format("reverseCache-%s", UUID.randomUUID());
mapCache = DB.createHashMap(cacheName).make();
reverseCache = DB.createHashMap(reverseCacheName).make();
ImmutableSet.Builder setOfValuesBuilder = ImmutableSet.builder();
for (Map.Entry entry : entries) {
mapCache.put(entry.getKey(), entry.getValue());
setOfValuesBuilder.add(entry.getValue());
}
final Set setOfValues = setOfValuesBuilder.build();
reverseCache.putAll(Maps.asMap(
setOfValues, new Function>()
{
@Override
public List apply(final V input)
{
return Lists.newArrayList(Maps.filterKeys(mapCache, new Predicate()
{
@Override
public boolean apply(K key)
{
V retVal = mapCache.get(key);
if (retVal == null) {
return false;
}
return retVal.equals(input);
}
}).keySet());
}
}));
started.getAndSet(true);
}
}
@Override
public V get(K key)
{
return mapCache.get(key);
}
@Override
public List getKeys(V value)
{
final List listOfKey = reverseCache.get(value);
if (listOfKey == null) {
return Collections.emptyList();
}
return listOfKey;
}
@Override
public void close()
{
synchronized (started) {
if (started.getAndSet(false)) {
DB.delete(cacheName);
DB.delete(reverseCacheName);
}
}
}
public static class OffHeapPollingCacheProvider implements PollingCacheFactory
{
@Override
public PollingCache makeOf(Iterable> entries)
{
return new OffHeapPollingCache<>(entries);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy