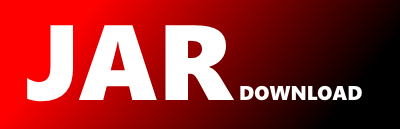
commonMain.socket.preset.PresetUpdates.kt Maven / Gradle / Ivy
// Code generated by Wire protocol buffer compiler, do not edit.
// Source: socket.preset.PresetUpdates in socket/preset.proto
package socket.preset
import com.squareup.wire.FieldEncoding
import com.squareup.wire.Message
import com.squareup.wire.ProtoAdapter
import com.squareup.wire.ProtoReader
import com.squareup.wire.ProtoWriter
import com.squareup.wire.ReverseProtoWriter
import com.squareup.wire.Syntax.PROTO_3
import com.squareup.wire.WireField
import kotlin.Any
import kotlin.AssertionError
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.DeprecationLevel
import kotlin.Int
import kotlin.Long
import kotlin.Nothing
import kotlin.String
import kotlin.Unit
import okio.ByteString
import socket.room.RecorderType
public class PresetUpdates(
@field:WireField(
tag = 1,
adapter = "socket.preset.PollsPermissionUpdate#ADAPTER",
)
public val polls: PollsPermissionUpdate? = null,
@field:WireField(
tag = 2,
adapter = "socket.preset.PluginsPermissionsUpdate#ADAPTER",
)
public val plugins: PluginsPermissionsUpdate? = null,
@field:WireField(
tag = 3,
adapter = "socket.preset.ChatPermissionUpdate#ADAPTER",
)
public val chat: ChatPermissionUpdate? = null,
@field:WireField(
tag = 4,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "acceptWaitingRequests",
)
public val accept_waiting_requests: Boolean? = null,
@field:WireField(
tag = 5,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "canAcceptProductionRequests",
)
public val can_accept_production_requests: Boolean? = null,
@field:WireField(
tag = 6,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "canEditDisplayName",
)
public val can_edit_display_name: Boolean? = null,
@field:WireField(
tag = 7,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "canRecord",
)
public val can_record: Boolean? = null,
@field:WireField(
tag = 8,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "canLivestream",
)
public val can_livestream: Boolean? = null,
@field:WireField(
tag = 9,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "canSpotlight",
)
public val can_spotlight: Boolean? = null,
@field:WireField(
tag = 10,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "disableParticipantAudio",
)
public val disable_participant_audio: Boolean? = null,
@field:WireField(
tag = 11,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "disableParticipantScreensharing",
)
public val disable_participant_screensharing: Boolean? = null,
@field:WireField(
tag = 12,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "disableParticipantVideo",
)
public val disable_participant_video: Boolean? = null,
@field:WireField(
tag = 13,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "kickParticipant",
)
public val kick_participant: Boolean? = null,
@field:WireField(
tag = 14,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "pinParticipant",
)
public val pin_participant: Boolean? = null,
@field:WireField(
tag = 15,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "transcriptionEnabled",
)
public val transcription_enabled: Boolean? = null,
@field:WireField(
tag = 16,
adapter = "socket.preset.WaitingRoomType#ADAPTER",
jsonName = "waitingRoomType",
)
public val waiting_room_type: WaitingRoomType? = null,
@field:WireField(
tag = 17,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "isRecorder",
)
public val is_recorder: Boolean? = null,
@field:WireField(
tag = 18,
adapter = "socket.room.RecorderType#ADAPTER",
jsonName = "recorderType",
)
public val recorder_type: RecorderType? = null,
@field:WireField(
tag = 19,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "hiddenParticipant",
)
public val hidden_participant: Boolean? = null,
@field:WireField(
tag = 20,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "showParticipantList",
)
public val show_participant_list: Boolean? = null,
@field:WireField(
tag = 21,
adapter = "com.squareup.wire.ProtoAdapter#BOOL",
jsonName = "canChangeParticipantPermissions",
)
public val can_change_participant_permissions: Boolean? = null,
@field:WireField(
tag = 22,
adapter = "socket.preset.ConnectedMeetingPermissionUpdate#ADAPTER",
jsonName = "connectedMeetings",
)
public val connected_meetings: ConnectedMeetingPermissionUpdate? = null,
@field:WireField(
tag = 23,
adapter = "socket.preset.MediaPermissionUpdate#ADAPTER",
)
public val media: MediaPermissionUpdate? = null,
unknownFields: ByteString = ByteString.EMPTY,
) : Message(ADAPTER, unknownFields) {
@Deprecated(
message = "Shouldn't be used in Kotlin",
level = DeprecationLevel.HIDDEN,
)
public override fun newBuilder(): Nothing = throw
AssertionError("Builders are deprecated and only available in a javaInterop build; see https://square.github.io/wire/wire_compiler/#kotlin")
public override fun equals(other: Any?): Boolean {
if (other === this) return true
if (other !is PresetUpdates) return false
if (unknownFields != other.unknownFields) return false
if (polls != other.polls) return false
if (plugins != other.plugins) return false
if (chat != other.chat) return false
if (accept_waiting_requests != other.accept_waiting_requests) return false
if (can_accept_production_requests != other.can_accept_production_requests) return false
if (can_edit_display_name != other.can_edit_display_name) return false
if (can_record != other.can_record) return false
if (can_livestream != other.can_livestream) return false
if (can_spotlight != other.can_spotlight) return false
if (disable_participant_audio != other.disable_participant_audio) return false
if (disable_participant_screensharing != other.disable_participant_screensharing) return false
if (disable_participant_video != other.disable_participant_video) return false
if (kick_participant != other.kick_participant) return false
if (pin_participant != other.pin_participant) return false
if (transcription_enabled != other.transcription_enabled) return false
if (waiting_room_type != other.waiting_room_type) return false
if (is_recorder != other.is_recorder) return false
if (recorder_type != other.recorder_type) return false
if (hidden_participant != other.hidden_participant) return false
if (show_participant_list != other.show_participant_list) return false
if (can_change_participant_permissions != other.can_change_participant_permissions) return false
if (connected_meetings != other.connected_meetings) return false
if (media != other.media) return false
return true
}
public override fun hashCode(): Int {
var result = super.hashCode
if (result == 0) {
result = unknownFields.hashCode()
result = result * 37 + (polls?.hashCode() ?: 0)
result = result * 37 + (plugins?.hashCode() ?: 0)
result = result * 37 + (chat?.hashCode() ?: 0)
result = result * 37 + (accept_waiting_requests?.hashCode() ?: 0)
result = result * 37 + (can_accept_production_requests?.hashCode() ?: 0)
result = result * 37 + (can_edit_display_name?.hashCode() ?: 0)
result = result * 37 + (can_record?.hashCode() ?: 0)
result = result * 37 + (can_livestream?.hashCode() ?: 0)
result = result * 37 + (can_spotlight?.hashCode() ?: 0)
result = result * 37 + (disable_participant_audio?.hashCode() ?: 0)
result = result * 37 + (disable_participant_screensharing?.hashCode() ?: 0)
result = result * 37 + (disable_participant_video?.hashCode() ?: 0)
result = result * 37 + (kick_participant?.hashCode() ?: 0)
result = result * 37 + (pin_participant?.hashCode() ?: 0)
result = result * 37 + (transcription_enabled?.hashCode() ?: 0)
result = result * 37 + (waiting_room_type?.hashCode() ?: 0)
result = result * 37 + (is_recorder?.hashCode() ?: 0)
result = result * 37 + (recorder_type?.hashCode() ?: 0)
result = result * 37 + (hidden_participant?.hashCode() ?: 0)
result = result * 37 + (show_participant_list?.hashCode() ?: 0)
result = result * 37 + (can_change_participant_permissions?.hashCode() ?: 0)
result = result * 37 + (connected_meetings?.hashCode() ?: 0)
result = result * 37 + (media?.hashCode() ?: 0)
super.hashCode = result
}
return result
}
public override fun toString(): String {
val result = mutableListOf()
if (polls != null) result += """polls=$polls"""
if (plugins != null) result += """plugins=$plugins"""
if (chat != null) result += """chat=$chat"""
if (accept_waiting_requests != null) result +=
"""accept_waiting_requests=$accept_waiting_requests"""
if (can_accept_production_requests != null) result +=
"""can_accept_production_requests=$can_accept_production_requests"""
if (can_edit_display_name != null) result += """can_edit_display_name=$can_edit_display_name"""
if (can_record != null) result += """can_record=$can_record"""
if (can_livestream != null) result += """can_livestream=$can_livestream"""
if (can_spotlight != null) result += """can_spotlight=$can_spotlight"""
if (disable_participant_audio != null) result +=
"""disable_participant_audio=$disable_participant_audio"""
if (disable_participant_screensharing != null) result +=
"""disable_participant_screensharing=$disable_participant_screensharing"""
if (disable_participant_video != null) result +=
"""disable_participant_video=$disable_participant_video"""
if (kick_participant != null) result += """kick_participant=$kick_participant"""
if (pin_participant != null) result += """pin_participant=$pin_participant"""
if (transcription_enabled != null) result += """transcription_enabled=$transcription_enabled"""
if (waiting_room_type != null) result += """waiting_room_type=$waiting_room_type"""
if (is_recorder != null) result += """is_recorder=$is_recorder"""
if (recorder_type != null) result += """recorder_type=$recorder_type"""
if (hidden_participant != null) result += """hidden_participant=$hidden_participant"""
if (show_participant_list != null) result += """show_participant_list=$show_participant_list"""
if (can_change_participant_permissions != null) result +=
"""can_change_participant_permissions=$can_change_participant_permissions"""
if (connected_meetings != null) result += """connected_meetings=$connected_meetings"""
if (media != null) result += """media=$media"""
return result.joinToString(prefix = "PresetUpdates{", separator = ", ", postfix = "}")
}
public fun copy(
polls: PollsPermissionUpdate? = this.polls,
plugins: PluginsPermissionsUpdate? = this.plugins,
chat: ChatPermissionUpdate? = this.chat,
accept_waiting_requests: Boolean? = this.accept_waiting_requests,
can_accept_production_requests: Boolean? = this.can_accept_production_requests,
can_edit_display_name: Boolean? = this.can_edit_display_name,
can_record: Boolean? = this.can_record,
can_livestream: Boolean? = this.can_livestream,
can_spotlight: Boolean? = this.can_spotlight,
disable_participant_audio: Boolean? = this.disable_participant_audio,
disable_participant_screensharing: Boolean? = this.disable_participant_screensharing,
disable_participant_video: Boolean? = this.disable_participant_video,
kick_participant: Boolean? = this.kick_participant,
pin_participant: Boolean? = this.pin_participant,
transcription_enabled: Boolean? = this.transcription_enabled,
waiting_room_type: WaitingRoomType? = this.waiting_room_type,
is_recorder: Boolean? = this.is_recorder,
recorder_type: RecorderType? = this.recorder_type,
hidden_participant: Boolean? = this.hidden_participant,
show_participant_list: Boolean? = this.show_participant_list,
can_change_participant_permissions: Boolean? = this.can_change_participant_permissions,
connected_meetings: ConnectedMeetingPermissionUpdate? = this.connected_meetings,
media: MediaPermissionUpdate? = this.media,
unknownFields: ByteString = this.unknownFields,
): PresetUpdates = PresetUpdates(polls, plugins, chat, accept_waiting_requests,
can_accept_production_requests, can_edit_display_name, can_record, can_livestream,
can_spotlight, disable_participant_audio, disable_participant_screensharing,
disable_participant_video, kick_participant, pin_participant, transcription_enabled,
waiting_room_type, is_recorder, recorder_type, hidden_participant, show_participant_list,
can_change_participant_permissions, connected_meetings, media, unknownFields)
public companion object {
public val ADAPTER: ProtoAdapter = object : ProtoAdapter(
FieldEncoding.LENGTH_DELIMITED,
PresetUpdates::class,
"type.googleapis.com/socket.preset.PresetUpdates",
PROTO_3,
null,
"socket/preset.proto"
) {
public override fun encodedSize(`value`: PresetUpdates): Int {
var size = value.unknownFields.size
size += PollsPermissionUpdate.ADAPTER.encodedSizeWithTag(1, value.polls)
size += PluginsPermissionsUpdate.ADAPTER.encodedSizeWithTag(2, value.plugins)
size += ChatPermissionUpdate.ADAPTER.encodedSizeWithTag(3, value.chat)
size += ProtoAdapter.BOOL.encodedSizeWithTag(4, value.accept_waiting_requests)
size += ProtoAdapter.BOOL.encodedSizeWithTag(5, value.can_accept_production_requests)
size += ProtoAdapter.BOOL.encodedSizeWithTag(6, value.can_edit_display_name)
size += ProtoAdapter.BOOL.encodedSizeWithTag(7, value.can_record)
size += ProtoAdapter.BOOL.encodedSizeWithTag(8, value.can_livestream)
size += ProtoAdapter.BOOL.encodedSizeWithTag(9, value.can_spotlight)
size += ProtoAdapter.BOOL.encodedSizeWithTag(10, value.disable_participant_audio)
size += ProtoAdapter.BOOL.encodedSizeWithTag(11, value.disable_participant_screensharing)
size += ProtoAdapter.BOOL.encodedSizeWithTag(12, value.disable_participant_video)
size += ProtoAdapter.BOOL.encodedSizeWithTag(13, value.kick_participant)
size += ProtoAdapter.BOOL.encodedSizeWithTag(14, value.pin_participant)
size += ProtoAdapter.BOOL.encodedSizeWithTag(15, value.transcription_enabled)
size += WaitingRoomType.ADAPTER.encodedSizeWithTag(16, value.waiting_room_type)
size += ProtoAdapter.BOOL.encodedSizeWithTag(17, value.is_recorder)
size += RecorderType.ADAPTER.encodedSizeWithTag(18, value.recorder_type)
size += ProtoAdapter.BOOL.encodedSizeWithTag(19, value.hidden_participant)
size += ProtoAdapter.BOOL.encodedSizeWithTag(20, value.show_participant_list)
size += ProtoAdapter.BOOL.encodedSizeWithTag(21, value.can_change_participant_permissions)
size += ConnectedMeetingPermissionUpdate.ADAPTER.encodedSizeWithTag(22,
value.connected_meetings)
size += MediaPermissionUpdate.ADAPTER.encodedSizeWithTag(23, value.media)
return size
}
public override fun encode(writer: ProtoWriter, `value`: PresetUpdates): Unit {
PollsPermissionUpdate.ADAPTER.encodeWithTag(writer, 1, value.polls)
PluginsPermissionsUpdate.ADAPTER.encodeWithTag(writer, 2, value.plugins)
ChatPermissionUpdate.ADAPTER.encodeWithTag(writer, 3, value.chat)
ProtoAdapter.BOOL.encodeWithTag(writer, 4, value.accept_waiting_requests)
ProtoAdapter.BOOL.encodeWithTag(writer, 5, value.can_accept_production_requests)
ProtoAdapter.BOOL.encodeWithTag(writer, 6, value.can_edit_display_name)
ProtoAdapter.BOOL.encodeWithTag(writer, 7, value.can_record)
ProtoAdapter.BOOL.encodeWithTag(writer, 8, value.can_livestream)
ProtoAdapter.BOOL.encodeWithTag(writer, 9, value.can_spotlight)
ProtoAdapter.BOOL.encodeWithTag(writer, 10, value.disable_participant_audio)
ProtoAdapter.BOOL.encodeWithTag(writer, 11, value.disable_participant_screensharing)
ProtoAdapter.BOOL.encodeWithTag(writer, 12, value.disable_participant_video)
ProtoAdapter.BOOL.encodeWithTag(writer, 13, value.kick_participant)
ProtoAdapter.BOOL.encodeWithTag(writer, 14, value.pin_participant)
ProtoAdapter.BOOL.encodeWithTag(writer, 15, value.transcription_enabled)
WaitingRoomType.ADAPTER.encodeWithTag(writer, 16, value.waiting_room_type)
ProtoAdapter.BOOL.encodeWithTag(writer, 17, value.is_recorder)
RecorderType.ADAPTER.encodeWithTag(writer, 18, value.recorder_type)
ProtoAdapter.BOOL.encodeWithTag(writer, 19, value.hidden_participant)
ProtoAdapter.BOOL.encodeWithTag(writer, 20, value.show_participant_list)
ProtoAdapter.BOOL.encodeWithTag(writer, 21, value.can_change_participant_permissions)
ConnectedMeetingPermissionUpdate.ADAPTER.encodeWithTag(writer, 22, value.connected_meetings)
MediaPermissionUpdate.ADAPTER.encodeWithTag(writer, 23, value.media)
writer.writeBytes(value.unknownFields)
}
public override fun encode(writer: ReverseProtoWriter, `value`: PresetUpdates): Unit {
writer.writeBytes(value.unknownFields)
MediaPermissionUpdate.ADAPTER.encodeWithTag(writer, 23, value.media)
ConnectedMeetingPermissionUpdate.ADAPTER.encodeWithTag(writer, 22, value.connected_meetings)
ProtoAdapter.BOOL.encodeWithTag(writer, 21, value.can_change_participant_permissions)
ProtoAdapter.BOOL.encodeWithTag(writer, 20, value.show_participant_list)
ProtoAdapter.BOOL.encodeWithTag(writer, 19, value.hidden_participant)
RecorderType.ADAPTER.encodeWithTag(writer, 18, value.recorder_type)
ProtoAdapter.BOOL.encodeWithTag(writer, 17, value.is_recorder)
WaitingRoomType.ADAPTER.encodeWithTag(writer, 16, value.waiting_room_type)
ProtoAdapter.BOOL.encodeWithTag(writer, 15, value.transcription_enabled)
ProtoAdapter.BOOL.encodeWithTag(writer, 14, value.pin_participant)
ProtoAdapter.BOOL.encodeWithTag(writer, 13, value.kick_participant)
ProtoAdapter.BOOL.encodeWithTag(writer, 12, value.disable_participant_video)
ProtoAdapter.BOOL.encodeWithTag(writer, 11, value.disable_participant_screensharing)
ProtoAdapter.BOOL.encodeWithTag(writer, 10, value.disable_participant_audio)
ProtoAdapter.BOOL.encodeWithTag(writer, 9, value.can_spotlight)
ProtoAdapter.BOOL.encodeWithTag(writer, 8, value.can_livestream)
ProtoAdapter.BOOL.encodeWithTag(writer, 7, value.can_record)
ProtoAdapter.BOOL.encodeWithTag(writer, 6, value.can_edit_display_name)
ProtoAdapter.BOOL.encodeWithTag(writer, 5, value.can_accept_production_requests)
ProtoAdapter.BOOL.encodeWithTag(writer, 4, value.accept_waiting_requests)
ChatPermissionUpdate.ADAPTER.encodeWithTag(writer, 3, value.chat)
PluginsPermissionsUpdate.ADAPTER.encodeWithTag(writer, 2, value.plugins)
PollsPermissionUpdate.ADAPTER.encodeWithTag(writer, 1, value.polls)
}
public override fun decode(reader: ProtoReader): PresetUpdates {
var polls: PollsPermissionUpdate? = null
var plugins: PluginsPermissionsUpdate? = null
var chat: ChatPermissionUpdate? = null
var accept_waiting_requests: Boolean? = null
var can_accept_production_requests: Boolean? = null
var can_edit_display_name: Boolean? = null
var can_record: Boolean? = null
var can_livestream: Boolean? = null
var can_spotlight: Boolean? = null
var disable_participant_audio: Boolean? = null
var disable_participant_screensharing: Boolean? = null
var disable_participant_video: Boolean? = null
var kick_participant: Boolean? = null
var pin_participant: Boolean? = null
var transcription_enabled: Boolean? = null
var waiting_room_type: WaitingRoomType? = null
var is_recorder: Boolean? = null
var recorder_type: RecorderType? = null
var hidden_participant: Boolean? = null
var show_participant_list: Boolean? = null
var can_change_participant_permissions: Boolean? = null
var connected_meetings: ConnectedMeetingPermissionUpdate? = null
var media: MediaPermissionUpdate? = null
val unknownFields = reader.forEachTag { tag ->
when (tag) {
1 -> polls = PollsPermissionUpdate.ADAPTER.decode(reader)
2 -> plugins = PluginsPermissionsUpdate.ADAPTER.decode(reader)
3 -> chat = ChatPermissionUpdate.ADAPTER.decode(reader)
4 -> accept_waiting_requests = ProtoAdapter.BOOL.decode(reader)
5 -> can_accept_production_requests = ProtoAdapter.BOOL.decode(reader)
6 -> can_edit_display_name = ProtoAdapter.BOOL.decode(reader)
7 -> can_record = ProtoAdapter.BOOL.decode(reader)
8 -> can_livestream = ProtoAdapter.BOOL.decode(reader)
9 -> can_spotlight = ProtoAdapter.BOOL.decode(reader)
10 -> disable_participant_audio = ProtoAdapter.BOOL.decode(reader)
11 -> disable_participant_screensharing = ProtoAdapter.BOOL.decode(reader)
12 -> disable_participant_video = ProtoAdapter.BOOL.decode(reader)
13 -> kick_participant = ProtoAdapter.BOOL.decode(reader)
14 -> pin_participant = ProtoAdapter.BOOL.decode(reader)
15 -> transcription_enabled = ProtoAdapter.BOOL.decode(reader)
16 -> try {
waiting_room_type = WaitingRoomType.ADAPTER.decode(reader)
} catch (e: ProtoAdapter.EnumConstantNotFoundException) {
reader.addUnknownField(tag, FieldEncoding.VARINT, e.value.toLong())
}
17 -> is_recorder = ProtoAdapter.BOOL.decode(reader)
18 -> try {
recorder_type = RecorderType.ADAPTER.decode(reader)
} catch (e: ProtoAdapter.EnumConstantNotFoundException) {
reader.addUnknownField(tag, FieldEncoding.VARINT, e.value.toLong())
}
19 -> hidden_participant = ProtoAdapter.BOOL.decode(reader)
20 -> show_participant_list = ProtoAdapter.BOOL.decode(reader)
21 -> can_change_participant_permissions = ProtoAdapter.BOOL.decode(reader)
22 -> connected_meetings = ConnectedMeetingPermissionUpdate.ADAPTER.decode(reader)
23 -> media = MediaPermissionUpdate.ADAPTER.decode(reader)
else -> reader.readUnknownField(tag)
}
}
return PresetUpdates(
polls = polls,
plugins = plugins,
chat = chat,
accept_waiting_requests = accept_waiting_requests,
can_accept_production_requests = can_accept_production_requests,
can_edit_display_name = can_edit_display_name,
can_record = can_record,
can_livestream = can_livestream,
can_spotlight = can_spotlight,
disable_participant_audio = disable_participant_audio,
disable_participant_screensharing = disable_participant_screensharing,
disable_participant_video = disable_participant_video,
kick_participant = kick_participant,
pin_participant = pin_participant,
transcription_enabled = transcription_enabled,
waiting_room_type = waiting_room_type,
is_recorder = is_recorder,
recorder_type = recorder_type,
hidden_participant = hidden_participant,
show_participant_list = show_participant_list,
can_change_participant_permissions = can_change_participant_permissions,
connected_meetings = connected_meetings,
media = media,
unknownFields = unknownFields
)
}
public override fun redact(`value`: PresetUpdates): PresetUpdates = value.copy(
polls = value.polls?.let(PollsPermissionUpdate.ADAPTER::redact),
plugins = value.plugins?.let(PluginsPermissionsUpdate.ADAPTER::redact),
chat = value.chat?.let(ChatPermissionUpdate.ADAPTER::redact),
connected_meetings =
value.connected_meetings?.let(ConnectedMeetingPermissionUpdate.ADAPTER::redact),
media = value.media?.let(MediaPermissionUpdate.ADAPTER::redact),
unknownFields = ByteString.EMPTY
)
}
private const val serialVersionUID: Long = 0L
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy