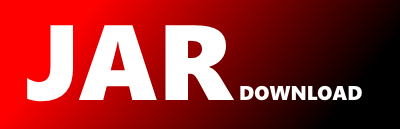
io.ebean.FetchGroupBuilder Maven / Gradle / Ivy
package io.ebean;
import org.jspecify.annotations.NullMarked;
/**
* Builds a FetchGroup by adding fetch clauses.
*
* We add select() and fetch() clauses to define the object graph we want to load.
*
*
* {@code
*
* FetchGroup fetchGroup = FetchGroup
* .select("name, status")
* .fetch("contacts", "firstName, lastName, email")
* .build();
*
* Customer.query()
* .select(fetchGroup)
* .where()
* ...
* .findList();
*
* }
*/
@NullMarked
public interface FetchGroupBuilder {
/**
* Specify specific properties to select (top level properties).
*/
FetchGroupBuilder select(String select);
/**
* Fetch all the properties at the given path.
*/
FetchGroupBuilder fetch(String path);
/**
* Fetch the path with the nested fetch group.
*/
FetchGroupBuilder fetch(String path, FetchGroup> nestedGroup);
/**
* Fetch the path using a query join with the nested fetch group.
*/
FetchGroupBuilder fetchQuery(String path, FetchGroup> nestedGroup);
/**
* Fetch the path lazily with the nested fetch group.
*/
FetchGroupBuilder fetchLazy(String path, FetchGroup> nestedGroup);
/**
* Fetch the path including specified properties.
*/
FetchGroupBuilder fetch(String path, String properties);
/**
* Fetch the path including all its properties using a query join.
*/
FetchGroupBuilder fetchQuery(String path);
/**
* Fetch the path including all its properties using L2 cache.
* Cache misses fallback to fetchQuery().
*/
FetchGroupBuilder fetchCache(String path);
/**
* Fetch the path including specified properties using a query join.
*/
FetchGroupBuilder fetchQuery(String path, String properties);
/**
* Fetch the path including specified properties using L2 cache.
* Cache misses fallback to fetchQuery().
*/
FetchGroupBuilder fetchCache(String path, String properties);
/**
* Fetch the path including all its properties lazily.
*/
FetchGroupBuilder fetchLazy(String path);
/**
* Fetch the path including specified properties lazily.
*/
FetchGroupBuilder fetchLazy(String path, String properties);
/**
* Build and return the FetchGroup.
*/
FetchGroup build();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy