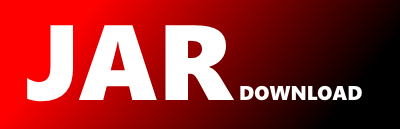
io.ebean.cache.ServerCache Maven / Gradle / Ivy
package io.ebean.cache;
import org.jspecify.annotations.Nullable;
import io.ebean.meta.MetricVisitor;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
/**
* Represents part of the "L2" server side cache.
*
* This is used to cache beans or query results (bean collections).
*
* There are 2 ServerCache's for each bean type. One is used as the 'bean cache'
* which holds beans of a given type. The other is the 'query cache' holding
* query results for a given type.
*/
public interface ServerCache {
/**
* Get values for many keys.
*/
default Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy