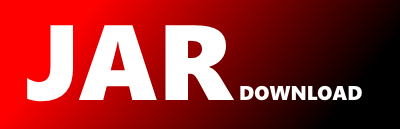
io.ebean.meta.BasicMetricVisitor Maven / Gradle / Ivy
package io.ebean.meta;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
/**
* A simple MetricVisitor that can collect the desired metrics into lists.
*/
public class BasicMetricVisitor extends AbstractMetricVisitor implements ServerMetrics {
private final String name;
private final Function naming;
private final List timed = new ArrayList<>();
private final List query = new ArrayList<>();
private final List count = new ArrayList<>();
public BasicMetricVisitor() {
this("db", MetricNamingMatch.INSTANCE);
}
/**
* Construct to reset and collect everything.
*/
public BasicMetricVisitor(String name, Function naming) {
this(name, naming, true, true, true, true);
}
/**
* Construct specifying reset and what to collect.
*/
public BasicMetricVisitor(String name, Function naming, boolean reset, boolean collectTransactionMetrics, boolean collectQueryMetrics, boolean collectL2Metrics) {
super(reset, collectTransactionMetrics, collectQueryMetrics, collectL2Metrics);
this.name = name;
this.naming = naming;
}
@Override
public String name() {
return name;
}
@Override
public Function namingConvention() {
return naming;
}
@Override
public ServerMetricsAsJson asJson() {
return new MetricsAsJson(this);
}
@Override
public List asData() {
return new MetricsAsData(this).data();
}
@Override
public List timedMetrics() {
return timed;
}
@Override
public List queryMetrics() {
return query;
}
@Override
public List countMetrics() {
return count;
}
@Override
public void visitTimed(MetaTimedMetric metric) {
timed.add(metric);
}
@Override
public void visitQuery(MetaQueryMetric metric) {
query.add(metric);
}
@Override
public void visitCount(MetaCountMetric metric) {
count.add(metric);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy