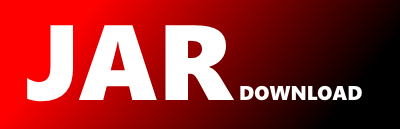
io.ebean.Filter Maven / Gradle / Ivy
package io.ebean;
import io.avaje.lang.NonNullApi;
import java.util.List;
import java.util.Set;
/**
* Provides support for filtering and sorting lists of entities without going
* back to the database.
*
* That is, it uses local in-memory sorting and filtering of a list of entity
* beans. It is not used in a Database query or invoke a Database query.
*
*
* You can optionally specify a sortByClause and if so, the sort will always
* execute prior to the filter expressions. You can specify any number of filter
* expressions and they are effectively joined by logical "AND".
*
*
* The result of the filter method will leave the original list unmodified and
* return a new List instance.
*
*
*
{@code
*
* // get a list of entities (query execution statistics in this case)
*
* List list =
* DB.find(MetaQueryStatistic.class).findList();
*
* long nowMinus24Hrs = System.currentTimeMillis() - 24 * (1000 * 60 * 60);
*
* // sort and filter the list returning a filtered list...
*
* List filteredList =
* DB.filter(MetaQueryStatistic.class)
* .sort("avgTimeMicros desc")
* .gt("executionCount", 0)
* .gt("lastQueryTime", nowMinus24Hrs)
* .eq("autoTuned", true)
* .maxRows(10)
* .filter(list);
*
* }
*
* The propertyNames can traverse the object graph (e.g. customer.name) by using
* dot notation. If any point during the object graph traversal to get a
* property value is null then null is returned.
*
*
*
{@code
*
* // examples of property names that
* // ... will traverse the object graph
* // ... where customer is a property of our bean
*
* customer.name
* customer.shippingAddress.city
*
* }
*
*
{@code
*
* // get a list of entities (query execution statistics)
*
* List orders =
* DB.find(Order.class).findList();
*
* // Apply a filter...
*
* List filteredOrders =
* DB.filter(Order.class)
* .startsWith("customer.name", "Rob")
* .eq("customer.shippingAddress.city", "Auckland")
* .filter(orders);
*
* }
*
* @param the entity bean type
*/
@NonNullApi
public interface Filter {
/**
* Specify a sortByClause.
*
* The sort (if specified) will always execute first followed by the filter
* expressions.
*
*
* Refer to {@link DB#sort(List, String)} for more detail.
*
*/
Filter sort(String sortByClause);
/**
* Specify the maximum number of rows/elements to return.
*/
Filter maxRows(int maxRows);
/**
* Equal To - property equal to the given value.
*/
Filter eq(String prop, Object value);
/**
* Not Equal To - property not equal to the given value.
*/
Filter ne(String propertyName, Object value);
/**
* Case Insensitive Equal To.
*/
Filter ieq(String propertyName, String value);
/**
* Between - property between the two given values.
*/
Filter between(String propertyName, Object value1, Object value2);
/**
* Greater Than - property greater than the given value.
*/
Filter gt(String propertyName, Object value);
/**
* Greater Than or Equal to - property greater than or equal to the given
* value.
*/
Filter ge(String propertyName, Object value);
/**
* Less Than - property less than the given value.
*/
Filter lt(String propertyName, Object value);
/**
* Less Than or Equal to - property less than or equal to the given value.
*/
Filter le(String propertyName, Object value);
/**
* Is Null - property is null.
*/
Filter isNull(String propertyName);
/**
* Is Not Null - property is not null.
*/
Filter isNotNull(String propertyName);
/**
* Starts With.
*/
Filter startsWith(String propertyName, String value);
/**
* Case insensitive Starts With.
*/
Filter istartsWith(String propertyName, String value);
/**
* Ends With.
*/
Filter endsWith(String propertyName, String value);
/**
* Case insensitive Ends With.
*/
Filter iendsWith(String propertyName, String value);
/**
* Contains - property contains the string "value".
*/
Filter contains(String propertyName, String value);
/**
* Case insensitive Contains.
*/
Filter icontains(String propertyName, String value);
/**
* In - property has a value contained in the set of values.
*/
Filter in(String propertyName, Set> values);
/**
* Apply the filter to the list returning a new list of the matching elements
* in the sorted order.
*
* The sourceList will remain unmodified.
*
*
* @return Returns a new list with the sorting and filters applied.
*/
List filter(List sourceList);
}