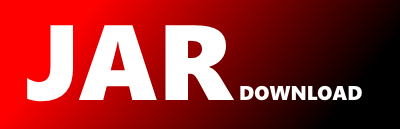
io.ebeaninternal.api.SpiEbeanServer Maven / Gradle / Ivy
package io.ebeaninternal.api;
import io.avaje.lang.Nullable;
import io.ebean.*;
import io.ebean.bean.BeanCollectionLoader;
import io.ebean.bean.CallOrigin;
import io.ebean.event.readaudit.ReadAuditLogger;
import io.ebean.event.readaudit.ReadAuditPrepare;
import io.ebean.meta.MetricVisitor;
import io.ebean.plugin.SpiServer;
import io.ebeaninternal.api.SpiQuery.Type;
import io.ebeaninternal.server.core.SpiResultSet;
import io.ebeaninternal.server.core.timezone.DataTimeZone;
import io.ebeaninternal.server.deploy.BeanDescriptor;
import io.ebeaninternal.server.query.CQuery;
import io.ebeaninternal.server.transaction.RemoteTransactionEvent;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
import java.util.function.Predicate;
import java.util.stream.Stream;
/**
* Service Provider extension to EbeanServer.
*/
public interface SpiEbeanServer extends SpiServer, ExtendedServer, BeanCollectionLoader {
/**
* Return the NOW time from the Clock.
*/
long clockNow();
/**
* Return true if the L2 cache has been disabled.
*/
boolean isDisableL2Cache();
/**
* Return the log manager.
*/
SpiLogManager log();
/**
* Return the server extended Json context.
*/
SpiJsonContext jsonExtended();
/**
* Return true if updates in JDBC batch should include all columns if unspecified on the transaction.
*/
boolean isUpdateAllPropertiesInBatch();
/**
* Return the current Tenant Id.
*/
Object currentTenantId();
/**
* Create an object to represent the current CallStack.
*
* Typically used to identify the origin of queries for AutoTune and object
* graph costing.
*/
CallOrigin createCallOrigin();
/**
* Override in order to return SpiQuery
*/
SpiQuery createQuery(Class beanType);
/**
* Return the PersistenceContextScope to use defined at query or server level.
*/
PersistenceContextScope persistenceContextScope(SpiQuery> query);
/**
* Clear the query execution statistics.
*/
void clearQueryStatistics();
/**
* Return the transaction manager.
*/
SpiTransactionManager transactionManager();
/**
* End the current transaction if it is active.
*/
void endTransaction();
/**
* Return all the descriptors.
*/
List> descriptors();
/**
* Return the BeanDescriptor for a given type of bean.
*/
BeanDescriptor descriptor(Class type);
/**
* Return BeanDescriptor using it's unique id.
*/
BeanDescriptor> descriptorById(String className);
/**
* Return BeanDescriptor using it's unique doc store queueId.
*/
BeanDescriptor> descriptorByQueueId(String queueId);
/**
* Return BeanDescriptors mapped to this table.
*/
List> descriptors(String tableName);
/**
* Process committed changes from another framework.
*
* This notifies this instance of the framework that beans have been committed
* externally to it. Either by another framework or clustered server. It uses
* this to maintain its cache and text indexes appropriately.
*
*/
void externalModification(TransactionEventTable event);
/**
* Clear an implicit transaction from the scope.
*/
void clearServerTransaction();
/**
* Begin a managed transaction (Uses scope manager / ThreadLocal).
*/
SpiTransaction beginServerTransaction();
/**
* Return the current transaction or null if there is no current transaction.
*/
SpiTransaction currentServerTransaction();
/**
* Create a ServerTransaction for query purposes.
*
* @param tenantId For multi-tenant lazy loading provide the tenantId to use.
* @param useMaster Set to true when the query should use the master data source.
*/
SpiTransaction createReadOnlyTransaction(Object tenantId, boolean useMaster);
/**
* An event from another server in the cluster used to notify local
* BeanListeners of remote inserts updates and deletes.
*/
void remoteTransactionEvent(RemoteTransactionEvent event);
/**
* Compile a query.
*/
CQuery compileQuery(Type type, SpiQuery query, Transaction transaction);
/**
* Execute the findId's query but without copying the query.
*
* Used so that the list of Id's can be made accessible to client code before
* the query has finished (if executing in a background thread).
*
*/
List findIdsWithCopy(SpiQuery query);
/**
* Execute the findCount query but without copying the query.
*/
int findCountWithCopy(SpiQuery query);
/**
* Load a batch of Associated One Beans.
*/
void loadBean(LoadBeanRequest loadRequest);
/**
* Lazy load a batch of Many's.
*/
void loadMany(LoadManyRequest loadRequest);
/**
* Return the default batch size for lazy loading.
*/
int lazyLoadBatchSize();
/**
* Return true if the type is known as an Entity or Xml type or a List Set or
* Map of known bean types.
*/
boolean isSupportedType(java.lang.reflect.Type genericType);
/**
* Return the ReadAuditLogger to use for logging all read audit events.
*/
ReadAuditLogger readAuditLogger();
/**
* Return the ReadAuditPrepare used to populate the read audit events with
* user context information (user id, user ip address etc).
*/
ReadAuditPrepare readAuditPrepare();
/**
* Return the DataTimeZone to use when reading/writing timestamps via JDBC.
*/
DataTimeZone dataTimeZone();
/**
* Check for slow query event.
*/
void slowQueryCheck(long executionTimeMicros, int rowCount, SpiQuery> query);
/**
* Start an enhanced transactional method.
*/
void scopedTransactionEnter(TxScope txScope);
/**
* Handle the end of an enhanced Transactional method.
*/
void scopedTransactionExit(Object returnOrThrowable, int opCode);
/**
* SqlQuery find single attribute.
*/
T findSingleAttribute(SpiSqlQuery query, Class cls);
/**
* SqlQuery find single attribute list.
*/
List findSingleAttributeList(SpiSqlQuery query, Class cls);
/**
* SqlQuery find single attribute streaming the result to a consumer.
*/
void findSingleAttributeEach(SpiSqlQuery query, Class cls, Consumer consumer);
/**
* SqlQuery find one with mapper.
*/
T findOneMapper(SpiSqlQuery query, RowMapper mapper);
/**
* SqlQuery find list with mapper.
*/
List findListMapper(SpiSqlQuery query, RowMapper mapper);
/**
* SqlQuery find each with consumer.
*/
void findEachRow(SpiSqlQuery query, RowConsumer consumer);
/**
* DTO findIterate query.
*/
QueryIterator findDtoIterate(SpiDtoQuery query);
/**
* DTO findStream query.
*/
Stream findDtoStream(SpiDtoQuery query);
/**
* DTO findList query.
*/
List findDtoList(SpiDtoQuery query);
/**
* DTO findOne query.
*/
@Nullable
T findDtoOne(SpiDtoQuery query);
/**
* DTO findEach query.
*/
void findDtoEach(SpiDtoQuery query, Consumer consumer);
/**
* DTO findEach batch query.
*/
void findDtoEach(SpiDtoQuery query, int batch, Consumer> consumer);
/**
* DTO findEachWhile query.
*/
void findDtoEachWhile(SpiDtoQuery query, Predicate consumer);
/**
* Return / wrap the ORM query as a DTO query.
*/
DtoQuery findDto(Class dtoType, SpiQuery> ormQuery);
/**
* Execute the underlying ORM query returning as a JDBC ResultSet to map to DTO beans.
*/
SpiResultSet findResultSet(SpiQuery> ormQuery);
/**
* Visit all the metrics (typically reporting them).
*/
void visitMetrics(MetricVisitor visitor);
/**
* Return true if a row for the bean type and id exists.
*/
boolean exists(Class> beanType, Object beanId, Transaction transaction);
/**
* Add to JDBC batch for later execution.
*/
void addBatch(SpiSqlUpdate defaultSqlUpdate, SpiTransaction transaction);
/**
* Execute the batched statement.
*/
int[] executeBatch(SpiSqlUpdate defaultSqlUpdate, SpiTransaction transaction);
/**
* Execute the sql update regardless of transaction batch mode.
*/
int executeNow(SpiSqlUpdate sqlUpdate);
/**
* Create a query bind capture for the given query plan.
*/
SpiQueryBindCapture createQueryBindCapture(SpiQueryPlan queryPlan);
boolean exists(SpiQuery ormQuery);
int findCount(SpiQuery query);
List findIds(SpiQuery query);
QueryIterator findIterate(SpiQuery query);
Stream findStream(SpiQuery query);
void findEach(SpiQuery query, Consumer consumer);
void findEach(SpiQuery query, int batch, Consumer> consumer);
void findEachWhile(SpiQuery query, Predicate consumer);
List> findVersions(SpiQuery query);
List findList(SpiQuery query);
FutureRowCount findFutureCount(SpiQuery query);
FutureIds findFutureIds(SpiQuery query);
FutureList findFutureList(SpiQuery query);
PagedList findPagedList(SpiQuery query);
Set findSet(SpiQuery query);
Map findMap(SpiQuery query);
List findSingleAttributeList(SpiQuery query);
Set findSingleAttributeSet(SpiQuery query);
@Nullable
T findOne(SpiQuery query);
Optional findOneOrEmpty(SpiQuery query);
int delete(SpiQuery query);
int update(SpiQuery query);
List findList(SpiSqlQuery query);
void findEach(SpiSqlQuery query, Consumer consumer);
void findEachWhile(SpiSqlQuery query, Predicate consumer);
@Nullable
SqlRow findOne(SpiSqlQuery query);
}