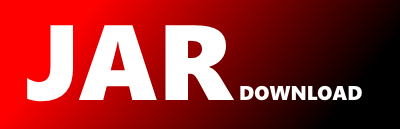
io.ebeaninternal.server.deploy.ChainedBeanPostLoad Maven / Gradle / Ivy
package io.ebeaninternal.server.deploy;
import io.ebean.event.BeanPostLoad;
import java.util.ArrayList;
import java.util.List;
/**
* Handles multiple BeanPostLoad's for a given entity type.
*/
public final class ChainedBeanPostLoad implements BeanPostLoad {
private final List list;
private final BeanPostLoad[] chain;
/**
* Construct given the list of BeanPostLoad's.
*/
public ChainedBeanPostLoad(List list) {
this.list = list;
this.chain = list.toArray(new BeanPostLoad[0]);
}
/**
* Register a new BeanPostLoad and return the resulting chain.
*/
public ChainedBeanPostLoad register(BeanPostLoad c) {
if (list.contains(c)) {
return this;
} else {
List newList = new ArrayList<>(list);
newList.add(c);
return new ChainedBeanPostLoad(newList);
}
}
/**
* De-register a BeanPostLoad and return the resulting chain.
*/
public ChainedBeanPostLoad deregister(BeanPostLoad c) {
if (!list.contains(c)) {
return this;
} else {
List newList = new ArrayList<>(list);
newList.remove(c);
return new ChainedBeanPostLoad(newList);
}
}
@Override
public boolean isRegisterFor(Class> cls) {
// never called
return false;
}
/**
* Fire postLoad on all registered BeanPostLoad implementations.
*/
@Override
public void postLoad(Object bean) {
for (BeanPostLoad postLoad : chain) {
postLoad.postLoad(bean);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy