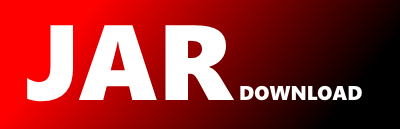
io.ebeaninternal.server.expression.NamedParamHelp Maven / Gradle / Ivy
package io.ebeaninternal.server.expression;
import io.ebeaninternal.api.SpiNamedParam;
import java.util.Collection;
import java.util.List;
/**
* Helper for evaluating named parameters.
*/
final class NamedParamHelp {
/**
* Return the bind value taking into account named parameters.
*/
static Object value(Object val) {
if (val instanceof SpiNamedParam) {
return ((SpiNamedParam) val).getValue();
}
return val;
}
/**
* Return the value as a string.
*/
static String valueAsString(Object val) {
Object value = value(val);
return (value == null) ? null : value.toString();
}
/**
* Add the potentially named parameter(s) to the values.
*/
public static void valueAdd(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy