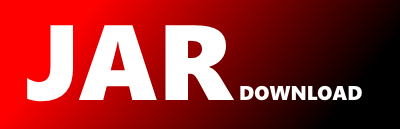
io.ebeaninternal.server.deploy.parse.AnnotationBase Maven / Gradle / Ivy
package io.ebeaninternal.server.deploy.parse;
import io.ebean.annotation.DbMigration;
import io.ebean.annotation.Index;
import io.ebean.annotation.Indices;
import io.ebean.annotation.Platform;
import io.ebean.config.NamingConvention;
import io.ebean.config.dbplatform.DatabasePlatform;
import io.ebean.util.AnnotationUtil;
import io.ebeaninternal.server.deploy.meta.DeployBeanProperty;
import jakarta.persistence.AttributeOverride;
import jakarta.persistence.JoinColumn;
import jakarta.persistence.JoinColumns;
import jakarta.persistence.NamedQueries;
import jakarta.persistence.NamedQuery;
import java.lang.annotation.Annotation;
import java.util.Collections;
import java.util.Set;
/**
* Provides some base methods for processing deployment annotations. All findAnnotation* methods
* are capable to search for meta-annotations (annotation that has an other annotation)
*
*
search algorithm for ONE annotation:
*
* - Check if annotation is direct on the property
* - if not found: Check all annotations at the annotateElement
* if they have the needed annotation as meta annotation
* - if not found: go up to super class and try again
* (only findAnnotationRecursive)
*
* DFS (Depth-First-Search) is used. The algorithm is the same as it is used in Spring-Framework,
* as the code is taken from there.
*
*
search algoritm for a Set<Annotation> works a litte bit different, as it does not stop
* on the first match, but continues searching down to the last corner to find all annotations.
*
*
To prevent endless recursion, the search algoritm tracks all visited annotations
*
*
Supports also "java 1.6 repeatable containers" like{@link JoinColumn} / {@link JoinColumns}.
*
*
This means, searching for JoinColumn
will find them also if they are inside a
* JoinColumns
annotation
*/
abstract class AnnotationBase {
final DatabasePlatform databasePlatform;
protected final Platform platform;
final NamingConvention namingConvention;
final DeployUtil util;
AnnotationBase(DeployUtil util) {
this.util = util;
this.databasePlatform = util.dbPlatform();
this.platform = databasePlatform.platform();
this.namingConvention = util.namingConvention();
}
/**
* read the deployment annotations.
*/
public abstract void parse();
/**
* Checks string is null or empty .
*/
boolean isEmpty(String s) {
return s == null || s.trim().isEmpty();
}
T get(DeployBeanProperty prop, Class annClass) {
return AnnotationUtil.get(prop.getField(), annClass);
}
boolean has(DeployBeanProperty prop, Class annClass) {
return AnnotationUtil.has(prop.getField(), annClass);
}
Set annotationJoinColumns(DeployBeanProperty prop) {
return AnnotationFind.joinColumns(prop.getField());
}
Set annotationAttributeOverrides(DeployBeanProperty prop) {
return AnnotationFind.attributeOverrides(prop.getField());
}
Set annotationIndexes(DeployBeanProperty prop) {
return AnnotationFind.indexes(prop.getField());
}
Set annotationDbMigrations(DeployBeanProperty prop) {
return AnnotationFind.dbMigrations(prop.getField());
}
Set annotationClassIndexes(Class> cls) {
Set result = AnnotationUtil.typeGetAll(cls, Index.class);
for (Indices index : AnnotationUtil.typeGetAll(cls, Indices.class)) {
Collections.addAll(result, index.value());
}
return result;
}
Set annotationClassNamedQuery(Class> cls) {
Set result = AnnotationUtil.typeGetAll(cls, NamedQuery.class);
for (NamedQueries queries : AnnotationUtil.typeGetAll(cls, NamedQueries.class)) {
Collections.addAll(result, queries.value());
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy