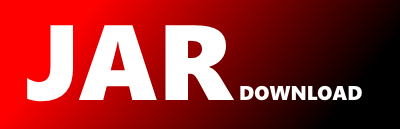
io.ebeaninternal.server.query.DFetchGroupBuilder Maven / Gradle / Ivy
package io.ebeaninternal.server.query;
import io.avaje.lang.NonNullApi;
import io.ebean.FetchConfig;
import io.ebean.FetchGroup;
import io.ebean.FetchGroupBuilder;
import io.ebeaninternal.server.querydefn.OrmQueryDetail;
import io.ebeaninternal.server.querydefn.SpiFetchGroup;
/**
* Default implementation of the FetchGroupBuilder.
*/
@NonNullApi
final class DFetchGroupBuilder implements FetchGroupBuilder {
private static final FetchConfig DEFAULT_FETCH = FetchConfig.ofDefault();
private static final FetchConfig FETCH_CACHE = FetchConfig.ofCache();
private static final FetchConfig FETCH_QUERY = FetchConfig.ofQuery();
private static final FetchConfig FETCH_LAZY = FetchConfig.ofLazy();
private final OrmQueryDetail detail;
DFetchGroupBuilder() {
this.detail = new OrmQueryDetail();
}
@Override
public FetchGroupBuilder select(String select) {
detail.select(select);
return this;
}
@Override
public FetchGroupBuilder fetch(String path) {
detail.fetchProperties(path, null, DEFAULT_FETCH);
return this;
}
@Override
public FetchGroupBuilder fetch(String path, FetchGroup> nestedGroup) {
return fetchNested(path, nestedGroup, DEFAULT_FETCH);
}
@Override
public FetchGroupBuilder fetchQuery(String path, FetchGroup> nestedGroup) {
return fetchNested(path, nestedGroup, FETCH_QUERY);
}
@Override
public FetchGroupBuilder fetchLazy(String path, FetchGroup> nestedGroup) {
return fetchNested(path, nestedGroup, FETCH_LAZY);
}
private FetchGroupBuilder fetchNested(String path, FetchGroup> nestedGroup, FetchConfig fetchConfig) {
OrmQueryDetail nestedDetail = ((SpiFetchGroup>) nestedGroup).underlying();
detail.addNested(path, nestedDetail, fetchConfig);
return this;
}
@Override
public FetchGroupBuilder fetchQuery(String path) {
detail.fetchProperties(path, null, FETCH_QUERY);
return this;
}
@Override
public FetchGroupBuilder fetchCache(String path) {
detail.fetchProperties(path, null, FETCH_CACHE);
return this;
}
@Override
public FetchGroupBuilder fetchLazy(String path) {
detail.fetchProperties(path, null, FETCH_LAZY);
return this;
}
@Override
public FetchGroupBuilder fetch(String path, String properties) {
detail.fetch(path, properties, DEFAULT_FETCH);
return this;
}
@Override
public FetchGroupBuilder fetchQuery(String path, String properties) {
detail.fetch(path, properties, FETCH_QUERY);
return this;
}
@Override
public FetchGroupBuilder fetchCache(String path, String properties) {
detail.fetch(path, properties, FETCH_CACHE);
return this;
}
@Override
public FetchGroupBuilder fetchLazy(String path, String properties) {
detail.fetch(path, properties, FETCH_LAZY);
return this;
}
@Override
public FetchGroup build() {
return new DFetchGroup<>(detail);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy