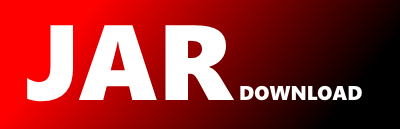
io.ebeaninternal.server.querydefn.DefaultDtoQuery Maven / Gradle / Ivy
package io.ebeaninternal.server.querydefn;
import io.avaje.lang.NonNullApi;
import io.ebean.DtoQuery;
import io.ebean.ProfileLocation;
import io.ebean.QueryIterator;
import io.ebean.Transaction;
import io.ebeaninternal.api.*;
import io.ebeaninternal.server.dto.DtoBeanDescriptor;
import io.ebeaninternal.server.dto.DtoMappingRequest;
import io.ebeaninternal.server.dto.DtoQueryPlan;
import io.ebeaninternal.server.transaction.ExternalJdbcTransaction;
import javax.annotation.Nullable;
import java.sql.Connection;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Predicate;
import java.util.stream.Stream;
/**
* Default implementation of DtoQuery.
*/
@NonNullApi
public final class DefaultDtoQuery extends AbstractQuery implements SpiDtoQuery {
private final SpiEbeanServer server;
private final DtoBeanDescriptor descriptor;
private final SpiQuery> ormQuery;
private String sql;
private int firstRow;
private int maxRows;
private int timeout;
private int bufferFetchSizeHint;
private boolean relaxedMode;
private String label;
private ProfileLocation profileLocation;
private final BindParams bindParams = new BindParams();
private SpiTransaction transaction;
/**
* Create given an underlying ORM query.
*/
public DefaultDtoQuery(SpiEbeanServer server, DtoBeanDescriptor descriptor, SpiQuery> ormQuery) {
this.server = server;
this.descriptor = descriptor;
this.ormQuery = ormQuery;
this.useMaster = ormQuery.isUseMaster();
this.label = ormQuery.label();
this.profileLocation = ormQuery.profileLocation();
}
/**
* Create given a native SQL query.
*/
public DefaultDtoQuery(SpiEbeanServer server, DtoBeanDescriptor descriptor, String sql) {
this.server = server;
this.descriptor = descriptor;
this.ormQuery = null;
this.sql = sql;
}
@Override
public String planKey() {
return sql + ":first" + firstRow + ":max" + maxRows;
}
@Override
public DtoQueryPlan queryPlan(Object planKey) {
return descriptor.queryPlan(planKey);
}
@Override
public DtoQueryPlan buildPlan(DtoMappingRequest request) {
return descriptor.buildPlan(request);
}
@Override
public void putQueryPlan(Object planKey, DtoQueryPlan plan) {
descriptor.putQueryPlan(planKey, plan);
}
@Override
public DtoQuery usingTransaction(Transaction transaction) {
this.transaction = (SpiTransaction) transaction;
return this;
}
@Override
public DtoQuery usingConnection(Connection connection) {
this.transaction = new ExternalJdbcTransaction(connection);
return this;
}
@Override
public DtoQuery usingMaster() {
this.useMaster = true;
return this;
}
@Override
public boolean isUseMaster() {
return useMaster;
}
@Override
public void findEach(Consumer consumer) {
server.findDtoEach(this, consumer);
}
@Override
public void findEach(int batch, Consumer> consumer) {
server.findDtoEach(this, batch, consumer);
}
@Override
public void findEachWhile(Predicate consumer) {
server.findDtoEachWhile(this, consumer);
}
@Override
public QueryIterator findIterate() {
return server.findDtoIterate(this);
}
@Override
public Stream findStream() {
return server.findDtoStream(this);
}
@Override
public List findList() {
return server.findDtoList(this);
}
@Nullable
@Override
public T findOne() {
return server.findDtoOne(this);
}
@Override
public Optional findOneOrEmpty() {
return Optional.ofNullable(findOne());
}
@Override
public DtoQuery setNullParameter(String name, int jdbcType) {
if (ormQuery != null) {
ormQuery.initBindParams().setNullParameter(name, jdbcType);
} else {
bindParams.setNullParameter(name, jdbcType);
}
return this;
}
@Override
public DtoQuery setNullParameter(int position, int jdbcType) {
if (ormQuery != null) {
ormQuery.initBindParams().setNullParameter(position, jdbcType);
} else {
bindParams.setNullParameter(position, jdbcType);
}
return this;
}
@Override
public DtoQuery setParameter(int position, Object value) {
if (ormQuery != null) {
ormQuery.setParameter(position, value);
} else {
bindParams.setParameter(position, value);
}
return this;
}
@Override
public DtoQuery setParameter(String paramName, Object value) {
if (ormQuery != null) {
ormQuery.setParameter(paramName, value);
} else {
bindParams.setParameter(paramName, value);
}
return this;
}
@Override
public DtoQuery setArrayParameter(String paramName, Collection> values) {
if (ormQuery != null) {
ormQuery.setArrayParameter(paramName, values);
} else {
bindParams.setArrayParameter(paramName, values);
}
return this;
}
@Override
public DtoQuery setParameters(Object... values) {
if (ormQuery != null) {
ormQuery.setParameters(values);
} else {
bindParams.setNextParameters(values);
}
return this;
}
@Override
public DtoQuery setParameter(Object value) {
if (ormQuery != null) {
ormQuery.setParameter(value);
} else {
bindParams.setNextParameter(value);
}
return this;
}
@Override
public String toString() {
return "DtoQuery " + sql;
}
@Override
public Class type() {
return descriptor.type();
}
@Override
public SpiQuery> ormQuery() {
return ormQuery;
}
@Override
public SpiTransaction transaction() {
return transaction;
}
@Override
public DtoQuery setRelaxedMode() {
this.relaxedMode = true;
return this;
}
@Override
public boolean isRelaxedMode() {
return relaxedMode;
}
@Override
public DtoQuery setLabel(String label) {
this.label = label;
if (ormQuery != null) {
ormQuery.setLabel(label);
}
return this;
}
@Override
public String getLabel() {
return label;
}
@Nullable
@Override
public String planLabel() {
if (label != null) {
return label;
}
if (profileLocation != null) {
return profileLocation.label();
}
return null;
}
@Override
public void obtainLocation() {
if (profileLocation != null) {
profileLocation.obtain();
}
}
@Override
public DtoQuery setProfileLocation(ProfileLocation profileLocation) {
this.profileLocation = profileLocation;
return this;
}
@Override
public ProfileLocation profileLocation() {
return profileLocation;
}
@Override
public int getFirstRow() {
return firstRow;
}
@Override
public DtoQuery setFirstRow(int firstRow) {
this.firstRow = firstRow;
if (ormQuery != null) {
ormQuery.setFirstRow(firstRow);
}
return this;
}
@Override
public int getMaxRows() {
return maxRows;
}
@Override
public DtoQuery setMaxRows(int maxRows) {
this.maxRows = maxRows;
if (ormQuery != null) {
ormQuery.setMaxRows(maxRows);
}
return this;
}
@Override
public int getTimeout() {
return timeout;
}
@Override
public DtoQuery setTimeout(int secs) {
this.timeout = secs;
return this;
}
@Override
public BindParams getBindParams() {
return bindParams;
}
@Override
public DtoQuery setBufferFetchSizeHint(int bufferFetchSizeHint) {
this.bufferFetchSizeHint = bufferFetchSizeHint;
return this;
}
@Override
public int getBufferFetchSizeHint() {
return bufferFetchSizeHint;
}
@Override
public String getQuery() {
return sql;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy