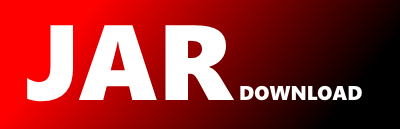
io.ebeaninternal.server.transaction.DefaultPersistenceContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebean Show documentation
Show all versions of ebean Show documentation
composite of common runtime dependencies for all platforms
package io.ebeaninternal.server.transaction;
import io.ebean.bean.PersistenceContext;
import io.ebeaninternal.api.Monitor;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
/**
* Default implementation of PersistenceContext.
*
* Ensures only one instance of a bean is used according to its type and unique
* id.
*
*
* PersistenceContext lives on a Transaction and as such is expected to only
* have a single thread accessing it at a time. This is not expected to be used
* concurrently.
*
*
* Duplicate beans are ones having the same type and unique id value. These are
* considered duplicates and replaced by the bean instance that was already
* loaded into the PersistenceContext.
*
*/
public final class DefaultPersistenceContext implements PersistenceContext {
/**
* Map used hold caches. One cache per bean type.
*/
private final HashMap, ClassContext> typeCache = new HashMap<>();
private final Monitor monitor = new Monitor();
/**
* Create a new PersistenceContext.
*/
public DefaultPersistenceContext() {
}
/**
* Set an object into the PersistenceContext.
*/
public void put(Class> rootType, Object id, Object bean) {
synchronized (monitor) {
getClassContext(rootType).put(id, bean);
}
}
public Object putIfAbsent(Class> rootType, Object id, Object bean) {
synchronized (monitor) {
return getClassContext(rootType).putIfAbsent(id, bean);
}
}
/**
* Return an object given its type and unique id.
*/
public Object get(Class> rootType, Object id) {
synchronized (monitor) {
return getClassContext(rootType).get(id);
}
}
public WithOption getWithOption(Class> rootType, Object id) {
synchronized (monitor) {
return getClassContext(rootType).getWithOption(id);
}
}
/**
* Return the number of beans of the given type in the persistence context.
*/
public int size(Class> rootType) {
synchronized (monitor) {
ClassContext classMap = typeCache.get(rootType);
return classMap == null ? 0 : classMap.size();
}
}
/**
* Clear the PersistenceContext.
*/
public void clear() {
synchronized (monitor) {
typeCache.clear();
}
}
public void clear(Class> rootType) {
synchronized (monitor) {
ClassContext classMap = typeCache.get(rootType);
if (classMap != null) {
classMap.clear();
}
}
}
public void deleted(Class> rootType, Object id) {
synchronized (monitor) {
ClassContext classMap = typeCache.get(rootType);
if (classMap != null && id != null) {
classMap.deleted(id);
}
}
}
public void clear(Class> rootType, Object id) {
synchronized (monitor) {
ClassContext classMap = typeCache.get(rootType);
if (classMap != null && id != null) {
classMap.remove(id);
}
}
}
public String toString() {
synchronized (monitor) {
return typeCache.toString();
}
}
private ClassContext getClassContext(Class> rootType) {
ClassContext classMap = typeCache.get(rootType);
if (classMap == null) {
classMap = new ClassContext();
typeCache.put(rootType, classMap);
}
return classMap;
}
private static class ClassContext {
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy