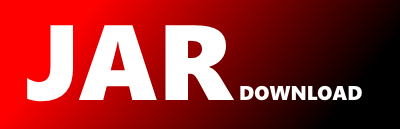
io.ebeaninternal.dbmigration.model.build.ModelBuildContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebean Show documentation
Show all versions of ebean Show documentation
composite of common runtime dependencies for all platforms
package io.ebeaninternal.dbmigration.model.build;
import io.ebean.config.DbConstraintNaming;
import io.ebean.config.dbplatform.DbPlatformType;
import io.ebean.config.dbplatform.DbPlatformTypeMapping;
import io.ebeaninternal.dbmigration.model.MColumn;
import io.ebeaninternal.dbmigration.model.MCompoundForeignKey;
import io.ebeaninternal.dbmigration.model.MTable;
import io.ebeaninternal.dbmigration.model.ModelContainer;
import io.ebeaninternal.server.deploy.BeanDescriptor;
import io.ebeaninternal.server.deploy.BeanProperty;
import io.ebeaninternal.server.deploy.TableJoin;
import io.ebeaninternal.server.deploy.TableJoinColumn;
import io.ebeaninternal.server.type.ScalarType;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
/**
* The context used during DDL generation.
*/
public class ModelBuildContext {
/**
* Use platform agnostic logical types. These types are converted to
* platform specific types in the DDL generation.
*/
private final DbPlatformTypeMapping dbTypeMap = DbPlatformTypeMapping.logicalTypes();
private final ModelContainer model;
private final DbConstraintNaming constraintNaming;
private final DbConstraintNaming.MaxLength maxLength;
private final boolean platformTypes;
public ModelBuildContext(ModelContainer model, DbConstraintNaming naming, DbConstraintNaming.MaxLength maxLength, boolean platformTypes) {
this.model = model;
this.constraintNaming = naming;
this.maxLength = maxLength;
this.platformTypes = platformTypes;
}
/**
* Adjust the foreign key references on any draft tables (that reference other draft tables).
* This is called as a 'second pass' after all the draft tables have been identified.
*/
public void adjustDraftReferences() {
model.adjustDraftReferences();
}
public String normaliseTable(String baseTable) {
return constraintNaming.normaliseTable(baseTable);
}
public String primaryKeyName(String tableName) {
return maxLength(constraintNaming.primaryKeyName(tableName), 0);
}
public String foreignKeyConstraintName(String tableName, String columnName, int foreignKeyCount) {
return maxLength(constraintNaming.foreignKeyConstraintName(tableName, columnName), foreignKeyCount);
}
public String foreignKeyIndexName(String tableName, String[] columns, int indexCount) {
return maxLength(constraintNaming.foreignKeyIndexName(tableName, columns), indexCount);
}
public String foreignKeyIndexName(String tableName, String column, int indexCount) {
return maxLength(constraintNaming.foreignKeyIndexName(tableName, column), indexCount);
}
public String indexName(String tableName, String column, int indexCount) {
return maxLength(constraintNaming.indexName(tableName, column), indexCount);
}
public String indexName(String tableName, String[] columns, int indexCount) {
return maxLength(constraintNaming.indexName(tableName, columns), indexCount);
}
public String uniqueConstraintName(String tableName, String columnName, int indexCount) {
return maxLength(constraintNaming.uniqueConstraintName(tableName, columnName), indexCount);
}
public String uniqueConstraintName(String tableName, String[] columnNames, int indexCount) {
return maxLength(constraintNaming.uniqueConstraintName(tableName, columnNames), indexCount);
}
public String checkConstraintName(String tableName, String columnName, int checkCount) {
return maxLength(constraintNaming.checkConstraintName(tableName, columnName), checkCount);
}
public MTable addTable(MTable table) {
return model.addTable(table);
}
public void addIndex(String indexName, String tableName, String columnName) {
model.addIndex(indexName, tableName, columnName);
}
public void addIndex(String indexName, String tableName, String[] columnNames) {
model.addIndex(indexName, tableName, columnNames);
}
private String maxLength(String constraintName, int indexCount) {
return maxLength.maxLength(constraintName, indexCount);
}
/**
* Return the map used to determine the DB specific type
* for a given bean property.
*/
public DbPlatformTypeMapping getDbTypeMap() {
return dbTypeMap;
}
/**
* Render the DB type for this property given the strict mode.
*/
public String getColumnDefn(BeanProperty p, boolean strict) {
DbPlatformType dbType = getDbType(p);
if (dbType == null) {
throw new IllegalStateException("Unknown DbType mapping for " + p.getFullBeanName());
}
return p.renderDbType(dbType, strict);
}
private DbPlatformType getDbType(BeanProperty p) {
if (p.isDbEncrypted()) {
return dbTypeMap.get(p.getDbEncryptedType());
}
if (p.isLocalEncrypted()) {
// scalar type potentially wrapping varbinary db type
ScalarType
© 2015 - 2025 Weber Informatics LLC | Privacy Policy