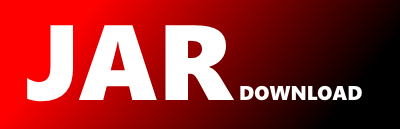
io.ebeaninternal.server.core.bootup.ManifestReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebean Show documentation
Show all versions of ebean Show documentation
composite of common runtime dependencies for all platforms
package io.ebeaninternal.server.core.bootup;
import io.ebean.util.StringHelper;
import io.ebeaninternal.util.UrlHelper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Collections;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Set;
import java.util.jar.Attributes;
import java.util.jar.Manifest;
/**
* Reads all the META-INF/ebean.mf resources with the package locations of entity beans.
*/
class ManifestReader {
private static final Logger logger = LoggerFactory.getLogger(ManifestReader.class);
private final Set packageSet = new HashSet<>();
private final ClassLoader classLoader;
/**
* Create with a classLoader to use to read the ebean.mf resources.
*/
static ManifestReader create(ClassLoader classLoader) {
return new ManifestReader(classLoader);
}
private ManifestReader(ClassLoader classLoader) {
this.classLoader = classLoader;
}
/**
* Read the packages from ebean.mf manifest files found as resources.
*/
ManifestReader read(String resourcePath) {
read(classLoader, resourcePath);
return this;
}
/**
* Return all the entityPackages read.
*/
Set entityPackages() {
return packageSet;
}
/**
* Read all the specific manifest files and return the set of packages containing type query beans.
*/
private Set read(ClassLoader classLoader, String resourcePath) {
try {
Enumeration resources = classLoader.getResources(resourcePath);
while (resources.hasMoreElements()) {
try (InputStream is = UrlHelper.openNoCache(resources.nextElement())) {
read(new Manifest(is));
}
}
} catch (IOException e) {
logger.warn("Error reading " + resourcePath + " manifest resources", e);
}
return packageSet;
}
/**
* Read the entity packages from the manifest.
*/
private void read(Manifest manifest) throws IOException {
Attributes attributes = manifest.getMainAttributes();
String agentOnlyUse = attributes.getValue("agent-use-only");
if (agentOnlyUse == null || !"true".equalsIgnoreCase(agentOnlyUse.trim())) {
add(attributes.getValue("packages"));
add(attributes.getValue("entity-packages"));
}
}
/**
* Collect each individual package splitting by delimiters.
*/
private void add(String packages) {
if (packages != null) {
Collections.addAll(packageSet, StringHelper.splitNames(packages));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy