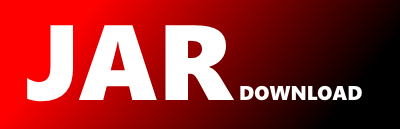
io.ebeaninternal.server.expression.JunctionExpression Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebean Show documentation
Show all versions of ebean Show documentation
composite of common runtime dependencies for all platforms
package io.ebeaninternal.server.expression;
import io.ebean.CacheMode;
import io.ebean.CountDistinctOrder;
import io.ebean.DtoQuery;
import io.ebean.Expression;
import io.ebean.ExpressionList;
import io.ebean.FetchGroup;
import io.ebean.FetchPath;
import io.ebean.FutureIds;
import io.ebean.FutureList;
import io.ebean.FutureRowCount;
import io.ebean.Junction;
import io.ebean.OrderBy;
import io.ebean.PagedList;
import io.ebean.Pairs;
import io.ebean.Query;
import io.ebean.QueryIterator;
import io.ebean.Transaction;
import io.ebean.UpdateQuery;
import io.ebean.Version;
import io.ebean.event.BeanQueryRequest;
import io.ebean.search.Match;
import io.ebean.search.MultiMatch;
import io.ebean.search.TextCommonTerms;
import io.ebean.search.TextQueryString;
import io.ebean.search.TextSimple;
import io.ebeaninternal.api.ManyWhereJoins;
import io.ebeaninternal.api.NaturalKeyQueryData;
import io.ebeaninternal.api.SpiExpression;
import io.ebeaninternal.api.SpiExpressionRequest;
import io.ebeaninternal.api.SpiExpressionValidation;
import io.ebeaninternal.api.SpiJunction;
import io.ebeaninternal.server.deploy.BeanDescriptor;
import java.io.IOException;
import java.sql.Connection;
import java.sql.Timestamp;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
import java.util.function.Predicate;
/**
* Junction implementation.
*/
class JunctionExpression implements SpiJunction, SpiExpression, ExpressionList {
protected DefaultExpressionList exprList;
protected Junction.Type type;
JunctionExpression(Junction.Type type, Query query, ExpressionList parent) {
this.type = type;
this.exprList = new DefaultExpressionList<>(query, parent);
}
/**
* Construct for copyForPlanKey.
*/
JunctionExpression(Junction.Type type, DefaultExpressionList exprList) {
this.type = type;
this.exprList = exprList;
}
@Override
public boolean naturalKey(NaturalKeyQueryData> data) {
// can't use naturalKey cache
return false;
}
/**
* Simplify nested expressions where possible.
*
* This is expected to only used after expressions are built via query language parsing.
*
*/
@SuppressWarnings("unchecked")
@Override
public void simplify() {
exprList.simplifyEntries();
List list = exprList.list;
if (list.size() == 1 && list.get(0) instanceof JunctionExpression) {
@SuppressWarnings("rawtypes")
JunctionExpression nested = (JunctionExpression) list.get(0);
if (type == Type.AND && !nested.type.isText()) {
// and (and (a, b, c)) -> and (a, b, c)
// and (not (a, b, c)) -> not (a, b, c)
// and (or (a, b, c)) -> or (a, b, c)
this.exprList = nested.exprList;
this.type = nested.type;
} else if (type == Type.NOT && nested.type == Type.AND) {
// not (and (a, b, c)) -> not (a, b, c)
this.exprList = nested.exprList;
}
}
}
@Override
public SpiExpression copyForPlanKey() {
return new JunctionExpression<>(type, exprList.copyForPlanKey());
}
@Override
public void writeDocQuery(DocQueryContext context) throws IOException {
context.startBool(type);
List list = exprList.internalList();
for (SpiExpression aList : list) {
aList.writeDocQuery(context);
}
context.endBool();
}
@Override
public void writeDocQueryJunction(DocQueryContext context) throws IOException {
context.startBoolGroupList(type);
List list = exprList.internalList();
for (SpiExpression aList : list) {
aList.writeDocQuery(context);
}
context.endBoolGroupList();
}
@Override
public Object getIdEqualTo(String idName) {
// always null for this expression
return null;
}
@Override
public void containsMany(BeanDescriptor> desc, ManyWhereJoins manyWhereJoin) {
List list = exprList.internalList();
// get the current state for 'require outer joins'
boolean parentOuterJoins = manyWhereJoin.isRequireOuterJoins();
if (type == Type.OR) {
// turn on outer joins required for disjunction expressions
manyWhereJoin.setRequireOuterJoins(true);
}
for (SpiExpression aList : list) {
aList.containsMany(desc, manyWhereJoin);
}
if (type == Type.OR && !parentOuterJoins) {
// restore state to not forcing outer joins
manyWhereJoin.setRequireOuterJoins(false);
}
}
@Override
public void validate(SpiExpressionValidation validation) {
exprList.validate(validation);
}
@Override
public Junction add(Expression item) {
exprList.add(item);
return this;
}
@Override
public Junction addAll(ExpressionList addList) {
exprList.addAll(addList);
return this;
}
@Override
public void addBindValues(SpiExpressionRequest request) {
List list = exprList.internalList();
for (SpiExpression aList : list) {
aList.addBindValues(request);
}
}
@Override
public void addSql(SpiExpressionRequest request) {
List list = exprList.internalList();
if (!list.isEmpty()) {
request.append(type.prefix());
request.append("(");
for (int i = 0; i < list.size(); i++) {
SpiExpression item = list.get(i);
if (i > 0) {
request.append(type.literal());
}
item.addSql(request);
}
request.append(")");
}
}
@Override
public void prepareExpression(BeanQueryRequest> request) {
List list = exprList.internalList();
for (SpiExpression aList : list) {
aList.prepareExpression(request);
}
}
/**
* Based on Junction type and all the expression contained.
*/
@Override
public void queryPlanHash(StringBuilder builder) {
builder.append(type).append("[");
List list = exprList.internalList();
for (SpiExpression aList : list) {
aList.queryPlanHash(builder);
builder.append(",");
}
builder.append("]");
}
@Override
public int queryBindHash() {
int hc = JunctionExpression.class.getName().hashCode();
List list = exprList.internalList();
for (SpiExpression aList : list) {
hc = hc * 92821 + aList.queryBindHash();
}
return hc;
}
@Override
public boolean isSameByBind(SpiExpression other) {
JunctionExpression> that = (JunctionExpression>) other;
return type == that.type && exprList.isSameByBind(that.exprList);
}
@Override
public ExpressionList match(String propertyName, String search) {
return match(propertyName, search, null);
}
@Override
public ExpressionList match(String propertyName, String search, Match options) {
return exprList.match(propertyName, search, options);
}
@Override
public ExpressionList multiMatch(String query, String... properties) {
return exprList.multiMatch(query, properties);
}
@Override
public ExpressionList multiMatch(String query, MultiMatch options) {
return exprList.multiMatch(query, options);
}
@Override
public ExpressionList textSimple(String search, TextSimple options) {
return exprList.textSimple(search, options);
}
@Override
public ExpressionList textQueryString(String search, TextQueryString options) {
return exprList.textQueryString(search, options);
}
@Override
public ExpressionList textCommonTerms(String search, TextCommonTerms options) {
return exprList.textCommonTerms(search, options);
}
@Override
public ExpressionList allEq(Map propertyMap) {
return exprList.allEq(propertyMap);
}
@Override
public ExpressionList and(Expression expOne, Expression expTwo) {
return exprList.and(expOne, expTwo);
}
@Override
public ExpressionList inRangeWith(String lowProperty, String highProperty, Object value) {
return exprList.inRangeWith(lowProperty, highProperty, value);
}
@Override
public ExpressionList inRange(String propertyName, Object value1, Object value2) {
return exprList.inRange(propertyName, value1, value2);
}
@Override
public ExpressionList between(String propertyName, Object value1, Object value2) {
return exprList.between(propertyName, value1, value2);
}
@Override
public ExpressionList betweenProperties(String lowProperty, String highProperty, Object value) {
return exprList.betweenProperties(lowProperty, highProperty, value);
}
@Override
public ExpressionList contains(String propertyName, String value) {
return exprList.contains(propertyName, value);
}
@Override
public ExpressionList endsWith(String propertyName, String value) {
return exprList.endsWith(propertyName, value);
}
@Override
public ExpressionList eq(String propertyName, Object value) {
return exprList.eq(propertyName, value);
}
@Override
public ExpressionList eqOrNull(String propertyName, Object value) {
return exprList.eqOrNull(propertyName, value);
}
@Override
public ExpressionList exampleLike(Object example) {
return exprList.exampleLike(example);
}
@Override
public ExpressionList where(String expressions, Object... params) {
throw new IllegalStateException("where not allowed on Junction expression list");
}
@Override
public ExpressionList filterMany(String prop) {
throw new IllegalStateException("filterMany not allowed on Junction expression list");
}
@Override
public ExpressionList filterMany(String manyProperty, String expressions, Object... params) {
throw new IllegalStateException("filterMany not allowed on Junction expression list");
}
@Override
public Query usingTransaction(Transaction transaction) {
return exprList.usingTransaction(transaction);
}
@Override
public Query usingConnection(Connection connection) {
return exprList.usingConnection(connection);
}
@Override
public int delete() {
return exprList.delete();
}
@Override
public int delete(Transaction transaction) {
return exprList.delete(transaction);
}
@Override
public int update() {
return exprList.update();
}
@Override
public int update(Transaction transaction) {
return exprList.update(transaction);
}
@Override
public Query asOf(Timestamp asOf) {
return exprList.asOf(asOf);
}
@Override
public Query asDraft() {
return exprList.asDraft();
}
@Override
public DtoQuery asDto(Class dtoClass) {
return exprList.asDto(dtoClass);
}
@Override
public UpdateQuery asUpdate() {
return exprList.asUpdate();
}
@Override
public Query setIncludeSoftDeletes() {
return exprList.setIncludeSoftDeletes();
}
@Override
public List> findVersions() {
return exprList.findVersions();
}
@Override
public List> findVersionsBetween(Timestamp start, Timestamp end) {
return exprList.findVersionsBetween(start, end);
}
@Override
public Query apply(FetchPath fetchPath) {
return exprList.apply(fetchPath);
}
@Override
public boolean exists() {
return exprList.exists();
}
@Override
public FutureIds findFutureIds() {
return exprList.findFutureIds();
}
@Override
public FutureList findFutureList() {
return exprList.findFutureList();
}
@Override
public FutureRowCount findFutureCount() {
return exprList.findFutureCount();
}
@Override
public List findIds() {
return exprList.findIds();
}
@Override
public QueryIterator findIterate() {
return exprList.findIterate();
}
@Override
public void findEach(Consumer consumer) {
exprList.findEach(consumer);
}
@Override
public void findEachWhile(Predicate consumer) {
exprList.findEachWhile(consumer);
}
@Override
public List findList() {
return exprList.findList();
}
@Override
public Map findMap() {
return exprList.findMap();
}
@Override
public List findSingleAttributeList() {
return exprList.findSingleAttributeList();
}
@Override
public PagedList findPagedList() {
return exprList.findPagedList();
}
@Override
public int findCount() {
return exprList.findCount();
}
@Override
public Set findSet() {
return exprList.findSet();
}
@Override
public T findOne() {
return exprList.findOne();
}
@Override
public Optional findOneOrEmpty() {
return exprList.findOneOrEmpty();
}
@Override
public Query forUpdate() {
return exprList.forUpdate();
}
@Override
public Query forUpdateNoWait() {
return exprList.forUpdateNoWait();
}
@Override
public Query forUpdateSkipLocked() {
return exprList.forUpdateSkipLocked();
}
/**
* Path exists - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonExists(String propertyName, String path) {
return exprList.jsonExists(propertyName, path);
}
/**
* Path does not exist - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonNotExists(String propertyName, String path) {
return exprList.jsonNotExists(propertyName, path);
}
/**
* Equal to - for the value at the given path in the JSON document.
*/
@Override
public ExpressionList jsonEqualTo(String propertyName, String path, Object value) {
return exprList.jsonEqualTo(propertyName, path, value);
}
/**
* Not Equal to - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonNotEqualTo(String propertyName, String path, Object val) {
return exprList.jsonNotEqualTo(propertyName, path, val);
}
/**
* Greater than - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonGreaterThan(String propertyName, String path, Object val) {
return exprList.jsonGreaterThan(propertyName, path, val);
}
/**
* Greater than or equal to - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonGreaterOrEqual(String propertyName, String path, Object val) {
return exprList.jsonGreaterOrEqual(propertyName, path, val);
}
/**
* Less than - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonLessThan(String propertyName, String path, Object val) {
return exprList.jsonLessThan(propertyName, path, val);
}
/**
* Less than or equal to - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonLessOrEqualTo(String propertyName, String path, Object val) {
return exprList.jsonLessOrEqualTo(propertyName, path, val);
}
/**
* Between - for the given path in a JSON document.
*/
@Override
public ExpressionList jsonBetween(String propertyName, String path, Object lowerValue, Object upperValue) {
return exprList.jsonBetween(propertyName, path, lowerValue, upperValue);
}
@Override
public ExpressionList arrayContains(String propertyName, Object... values) {
return exprList.arrayContains(propertyName, values);
}
@Override
public ExpressionList arrayNotContains(String propertyName, Object... values) {
return exprList.arrayNotContains(propertyName, values);
}
@Override
public ExpressionList arrayIsEmpty(String propertyName) {
return exprList.arrayIsEmpty(propertyName);
}
@Override
public ExpressionList arrayIsNotEmpty(String propertyName) {
return exprList.arrayIsNotEmpty(propertyName);
}
@Override
public ExpressionList bitwiseAny(String propertyName, long flags) {
return exprList.bitwiseAny(propertyName, flags);
}
@Override
public ExpressionList bitwiseAll(String propertyName, long flags) {
return exprList.bitwiseAll(propertyName, flags);
}
@Override
public ExpressionList bitwiseAnd(String propertyName, long flags, long match) {
return exprList.bitwiseAnd(propertyName, flags, match);
}
@Override
public ExpressionList bitwiseNot(String propertyName, long flags) {
return exprList.bitwiseNot(propertyName, flags);
}
@Override
public ExpressionList ge(String propertyName, Object value) {
return exprList.ge(propertyName, value);
}
@Override
public ExpressionList gt(String propertyName, Object value) {
return exprList.gt(propertyName, value);
}
@Override
public ExpressionList gtOrNull(String propertyName, Object value) {
return exprList.gtOrNull(propertyName, value);
}
@Override
public ExpressionList having() {
throw new IllegalStateException("having() not allowed on Junction expression list");
}
@Override
public ExpressionList icontains(String propertyName, String value) {
return exprList.icontains(propertyName, value);
}
@Override
public ExpressionList idEq(Object value) {
return exprList.idEq(value);
}
@Override
public ExpressionList idIn(Object... idValues) {
return exprList.idIn(idValues);
}
@Override
public ExpressionList idIn(Collection> idValues) {
return exprList.idIn(idValues);
}
@Override
public ExpressionList iendsWith(String propertyName, String value) {
return exprList.iendsWith(propertyName, value);
}
@Override
public ExpressionList ieq(String propertyName, String value) {
return exprList.ieq(propertyName, value);
}
@Override
public ExpressionList ine(String propertyName, String value) {
return exprList.ine(propertyName, value);
}
@Override
public ExpressionList iexampleLike(Object example) {
return exprList.iexampleLike(example);
}
@Override
public ExpressionList ilike(String propertyName, String value) {
return exprList.ilike(propertyName, value);
}
@Override
public ExpressionList inPairs(Pairs pairs) {
return exprList.inPairs(pairs);
}
@Override
public ExpressionList in(String propertyName, Collection> values) {
return exprList.in(propertyName, values);
}
@Override
public ExpressionList inOrEmpty(String propertyName, Collection> values) {
return exprList.inOrEmpty(propertyName, values);
}
@Override
public ExpressionList in(String propertyName, Object... values) {
return exprList.in(propertyName, values);
}
@Override
public ExpressionList in(String propertyName, Query> subQuery) {
return exprList.in(propertyName, subQuery);
}
@Override
public ExpressionList notIn(String propertyName, Collection> values) {
return exprList.notIn(propertyName, values);
}
@Override
public ExpressionList notIn(String propertyName, Object... values) {
return exprList.notIn(propertyName, values);
}
@Override
public ExpressionList notIn(String propertyName, Query> subQuery) {
return exprList.notIn(propertyName, subQuery);
}
@Override
public ExpressionList isEmpty(String propertyName) {
return exprList.isEmpty(propertyName);
}
@Override
public ExpressionList isNotEmpty(String propertyName) {
return exprList.isNotEmpty(propertyName);
}
@Override
public ExpressionList exists(Query> subQuery) {
return exprList.exists(subQuery);
}
@Override
public ExpressionList notExists(Query> subQuery) {
return exprList.notExists(subQuery);
}
@Override
public ExpressionList isNotNull(String propertyName) {
return exprList.isNotNull(propertyName);
}
@Override
public ExpressionList isNull(String propertyName) {
return exprList.isNull(propertyName);
}
@Override
public ExpressionList istartsWith(String propertyName, String value) {
return exprList.istartsWith(propertyName, value);
}
@Override
public ExpressionList le(String propertyName, Object value) {
return exprList.le(propertyName, value);
}
@Override
public ExpressionList like(String propertyName, String value) {
return exprList.like(propertyName, value);
}
@Override
public ExpressionList lt(String propertyName, Object value) {
return exprList.lt(propertyName, value);
}
@Override
public ExpressionList ltOrNull(String propertyName, Object value) {
return exprList.ltOrNull(propertyName, value);
}
@Override
public ExpressionList ne(String propertyName, Object value) {
return exprList.ne(propertyName, value);
}
@Override
public ExpressionList not(Expression exp) {
return exprList.not(exp);
}
@Override
public ExpressionList or(Expression expOne, Expression expTwo) {
return exprList.or(expOne, expTwo);
}
@Override
public OrderBy order() {
return exprList.order();
}
@Override
public ExpressionList order(String orderByClause) {
return exprList.order(orderByClause);
}
@Override
public OrderBy orderBy() {
return exprList.orderBy();
}
@Override
public ExpressionList orderBy(String orderBy) {
return exprList.orderBy(orderBy);
}
@Override
public Query orderById(boolean orderById) {
return exprList.orderById(orderById);
}
@Override
public Query query() {
return exprList.query();
}
@Override
public ExpressionList raw(String raw, Object value) {
return exprList.raw(raw, value);
}
@Override
public ExpressionList raw(String raw, Object... values) {
return exprList.raw(raw, values);
}
@Override
public ExpressionList rawOrEmpty(String raw, Collection> values) {
return exprList.rawOrEmpty(raw, values);
}
@Override
public ExpressionList raw(String raw) {
return exprList.raw(raw);
}
@Override
public Query select(String properties) {
return exprList.select(properties);
}
@Override
public Query select(FetchGroup fetchGroup) {
return exprList.select(fetchGroup);
}
@Override
public Query setDistinct(boolean distinct) {
return exprList.setDistinct(distinct);
}
@Override
public Query setDocIndexName(String indexName) {
return exprList.setDocIndexName(indexName);
}
@Override
public ExpressionList setFirstRow(int firstRow) {
return exprList.setFirstRow(firstRow);
}
@Override
public Query setMapKey(String mapKey) {
return exprList.setMapKey(mapKey);
}
@Override
public ExpressionList setMaxRows(int maxRows) {
return exprList.setMaxRows(maxRows);
}
@Override
public Query setOrderBy(String orderBy) {
return exprList.setOrderBy(orderBy);
}
@Override
public Query setUseCache(boolean useCache) {
return exprList.setUseCache(useCache);
}
@Override
public Query setBeanCacheMode(CacheMode useCache) {
return exprList.setBeanCacheMode(useCache);
}
@Override
public Query setUseQueryCache(CacheMode useCache) {
return exprList.setUseQueryCache(useCache);
}
@Override
public Query setUseDocStore(boolean useDocsStore) {
return exprList.setUseDocStore(useDocsStore);
}
@Override
public Query setDisableLazyLoading(boolean disableLazyLoading) {
return exprList.setDisableLazyLoading(disableLazyLoading);
}
@Override
public Query setDisableReadAuditing() {
return exprList.setDisableReadAuditing();
}
@Override
public Query setCountDistinct(CountDistinctOrder orderBy) {
return exprList.setCountDistinct(orderBy);
}
@Override
public Query setLabel(String label) {
return exprList.setLabel(label);
}
@Override
public ExpressionList startsWith(String propertyName, String value) {
return exprList.startsWith(propertyName, value);
}
@Override
public ExpressionList where() {
return exprList.where();
}
@Override
public Junction and() {
return conjunction();
}
@Override
public Junction or() {
return disjunction();
}
@Override
public Junction not() {
return exprList.not();
}
@Override
public Junction conjunction() {
return exprList.conjunction();
}
@Override
public Junction disjunction() {
return exprList.disjunction();
}
@Override
public Junction must() {
return exprList.must();
}
@Override
public Junction should() {
return exprList.should();
}
@Override
public Junction mustNot() {
return exprList.mustNot();
}
@Override
public ExpressionList endJunction() {
return exprList.endJunction();
}
@Override
public ExpressionList endAnd() {
return endJunction();
}
@Override
public ExpressionList endOr() {
return endJunction();
}
@Override
public ExpressionList endNot() {
return endJunction();
}
@Override
public String nestedPath(BeanDescriptor> desc) {
PrepareDocNested.prepare(exprList, desc, type);
String nestedPath = exprList.allDocNestedPath;
if (nestedPath != null) {
// push the nestedPath up to parent
exprList.setAllDocNested(null);
return nestedPath;
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy