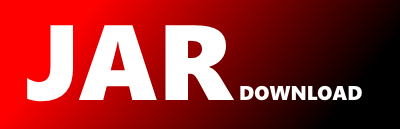
io.ebeaninternal.server.querydefn.DefaultDtoQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebean Show documentation
Show all versions of ebean Show documentation
composite of common runtime dependencies for all platforms
package io.ebeaninternal.server.querydefn;
import io.ebean.DtoQuery;
import io.ebean.ProfileLocation;
import io.ebean.Transaction;
import io.ebeaninternal.api.BindParams;
import io.ebeaninternal.api.SpiDtoQuery;
import io.ebeaninternal.api.SpiEbeanServer;
import io.ebeaninternal.api.SpiQuery;
import io.ebeaninternal.server.dto.DtoBeanDescriptor;
import io.ebeaninternal.server.dto.DtoMappingRequest;
import io.ebeaninternal.server.dto.DtoQueryPlan;
import java.util.List;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Predicate;
/**
* Default implementation of DtoQuery.
*/
public class DefaultDtoQuery implements SpiDtoQuery {
private final SpiEbeanServer server;
private final DtoBeanDescriptor descriptor;
private final SpiQuery> ormQuery;
private String sql;
private int firstRow;
private int maxRows;
private int timeout;
private int bufferFetchSizeHint;
private boolean relaxedMode;
private String label;
private ProfileLocation profileLocation;
/**
* Bind parameters when using the query language.
*/
private final BindParams bindParams = new BindParams();
private Transaction transaction;
/**
* Create given an underlying ORM query.
*/
public DefaultDtoQuery(SpiEbeanServer server, DtoBeanDescriptor descriptor, SpiQuery> ormQuery) {
this.server = server;
this.descriptor = descriptor;
this.ormQuery = ormQuery;
this.label = ormQuery.getLabel();
this.profileLocation = ormQuery.getProfileLocation();
}
/**
* Create given a native SQL query.
*/
public DefaultDtoQuery(SpiEbeanServer server, DtoBeanDescriptor descriptor, String sql) {
this.server = server;
this.descriptor = descriptor;
this.ormQuery = null;
this.sql = sql;
}
@Override
public String planKey() {
return sql + ":first" + firstRow + ":max" + maxRows;
}
@Override
public DtoQueryPlan getQueryPlan(Object planKey) {
return descriptor.getQueryPlan(planKey);
}
@Override
public DtoQueryPlan buildPlan(DtoMappingRequest request) {
return descriptor.buildPlan(request);
}
@Override
public void putQueryPlan(Object planKey, DtoQueryPlan plan) {
descriptor.putQueryPlan(planKey, plan);
}
@Override
public DtoQuery usingTransaction(Transaction transaction) {
this.transaction = transaction;
return this;
}
@Override
public void findEach(Consumer consumer) {
server.findDtoEach(this, consumer);
}
@Override
public void findEachWhile(Predicate consumer) {
server.findDtoEachWhile(this, consumer);
}
@Override
public List findList() {
return server.findDtoList(this);
}
@Override
public T findOne() {
return server.findDtoOne(this);
}
@Override
public Optional findOneOrEmpty() {
return Optional.ofNullable(findOne());
}
@Override
public DtoQuery setParameter(int position, Object value) {
if (ormQuery != null) {
ormQuery.setParameter(position, value);
} else {
bindParams.setParameter(position, value);
}
return this;
}
@Override
public DtoQuery setParameter(String paramName, Object value) {
if (ormQuery != null) {
ormQuery.setParameter(paramName, value);
} else {
bindParams.setParameter(paramName, value);
}
return this;
}
@Override
public DtoQuery setParameters(Object... values) {
if (ormQuery != null) {
ormQuery.setParameters(values);
} else {
bindParams.setNextParameters(values);
}
return this;
}
@Override
public DtoQuery setParameter(Object value) {
if (ormQuery != null) {
ormQuery.setParameter(value);
} else {
bindParams.setNextParameter(value);
}
return this;
}
@Override
public String toString() {
return "DtoQuery [" + sql + "]";
}
@Override
public Class getType() {
return descriptor.getType();
}
@Override
public SpiQuery> getOrmQuery() {
return ormQuery;
}
@Override
public Transaction getTransaction() {
return transaction;
}
@Override
public DtoQuery setRelaxedMode() {
this.relaxedMode = true;
return this;
}
@Override
public boolean isRelaxedMode() {
return relaxedMode;
}
@Override
public DtoQuery setLabel(String label) {
this.label = label;
return this;
}
@Override
public String getLabel() {
return label;
}
@Override
public String getPlanLabel() {
if (label != null) {
return label;
}
if (profileLocation != null) {
return profileLocation.label();
}
return null;
}
@Override
public void obtainLocation() {
if (profileLocation != null) {
profileLocation.obtain();
}
}
@Override
public DtoQuery setProfileLocation(ProfileLocation profileLocation) {
this.profileLocation = profileLocation;
return this;
}
@Override
public ProfileLocation getProfileLocation() {
return profileLocation;
}
@Override
public int getFirstRow() {
return firstRow;
}
@Override
public DtoQuery setFirstRow(int firstRow) {
this.firstRow = firstRow;
if (ormQuery != null) {
ormQuery.setFirstRow(firstRow);
}
return this;
}
@Override
public int getMaxRows() {
return maxRows;
}
@Override
public DtoQuery setMaxRows(int maxRows) {
this.maxRows = maxRows;
if (ormQuery != null) {
ormQuery.setMaxRows(maxRows);
}
return this;
}
@Override
public int getTimeout() {
return timeout;
}
@Override
public DtoQuery setTimeout(int secs) {
this.timeout = secs;
return this;
}
@Override
public BindParams getBindParams() {
return bindParams;
}
@Override
public DtoQuery setBufferFetchSizeHint(int bufferFetchSizeHint) {
this.bufferFetchSizeHint = bufferFetchSizeHint;
return this;
}
@Override
public int getBufferFetchSizeHint() {
return bufferFetchSizeHint;
}
@Override
public String getQuery() {
return sql;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy