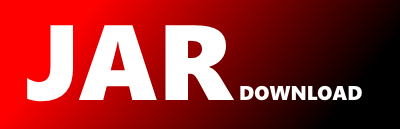
io.edurt.datacap.parser.node.Node Maven / Gradle / Ivy
package io.edurt.datacap.parser.node;
import io.edurt.datacap.parser.ast.AstVisitor;
import java.util.List;
import java.util.Optional;
import static java.util.Objects.requireNonNull;
public abstract class Node
{
private final Optional location;
protected Node(Optional location)
{
this.location = requireNonNull(location, "location is null");
}
protected static boolean sameClass(Node left, Node right)
{
if (left == right) {
return true;
}
return left.getClass() == right.getClass();
}
/**
* Accessible for {@link AstVisitor}, use {@link AstVisitor#process(Node, Object)} instead.
*/
public R accept(AstVisitor visitor, C context)
{
return visitor.visitNode(this, context);
}
public Optional getLocation()
{
return location;
}
public abstract List extends Node> getChildren();
// Force subclasses to have a proper equals and hashcode implementation
@Override
public abstract int hashCode();
@Override
public abstract boolean equals(Object obj);
@Override
public abstract String toString();
/**
* Compare with another node by considering internal state excluding any Node returned by getChildren()
*/
public boolean shallowEquals(Node other)
{
throw new UnsupportedOperationException("not yet implemented: " + getClass().getName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy