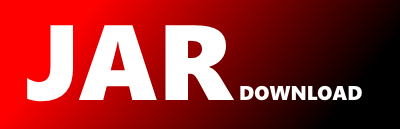
io.elastic.sailor.ExecutionContext Maven / Gradle / Ivy
package io.elastic.sailor;
import com.rabbitmq.client.AMQP;
import io.elastic.api.Message;
import java.util.HashMap;
import java.util.Map;
public class ExecutionContext {
private final Step step;
private final Message message;
private final Map headers;
public ExecutionContext(
final Step step,
final Message message,
final Map headers) {
this.step = step;
this.message = message;
this.headers = headers;
}
public Step getStep() {
return this.step;
}
public Map buildDefaultHeaders() {
final Map result = new HashMap();
result.put("execId", headers.get("execId"));
result.put("taskId", headers.get("taskId"));
result.put("userId", headers.get("userId"));
result.put("stepId", this.step.getId());
result.put("compId", this.step.getCompId());
result.put("function", this.step.getFunction());
result.put("start", System.currentTimeMillis());
return result;
}
public AMQP.BasicProperties buildDefaultOptions() {
return new AMQP.BasicProperties.Builder()
.contentType("application/json")
.contentEncoding("utf8")
.headers(buildDefaultHeaders())
.priority(1)// this should equal to mandatory true
.deliveryMode(2)//TODO: check if flag .mandatory(true) was set
.build();
}
public Message getMessage() {
return message;
}
public Map getHeaders() {
return headers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy