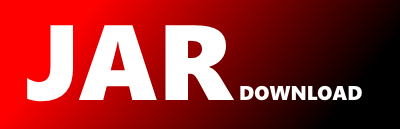
io.electrum.suv.api.models.RedemptionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of suv-service-interface Show documentation
Show all versions of suv-service-interface Show documentation
Single Use Voucher Service Interface
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy