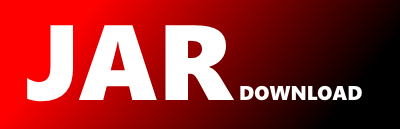
io.elsci.signals.mock.entities.SignalsEntityApi Maven / Gradle / Ivy
package io.elsci.signals.mock.entities;
import io.elsci.signals.mock.assets.AssetsDao;
import io.elsci.signals.mock.assets.TestObjectsFactory;
import io.elsci.signals.sdk.assets.Asset;
import io.elsci.signals.sdk.common.ListEntry;
import io.elsci.signals.sdk.common.SgMetaResponse;
import io.elsci.signals.sdk.entities.SearchEntry;
import io.elsci.signals.sdk.entities.SearchQuery;
import org.bson.Document;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.time.OffsetDateTime;
import java.time.ZoneId;
import java.util.*;
import static io.elsci.signals.mock.SignalsCommonUtils.SIGNALS_API_ROOT;
import static io.elsci.signals.sdk.SignalsSdkHttpUtil.SIGNALS_JSON_MIMETYPE;
@RestController
@RequestMapping(value = SIGNALS_API_ROOT + "/entities", produces = SIGNALS_JSON_MIMETYPE)
public class SignalsEntityApi {
private final AssetsDao assetsDao;
public SignalsEntityApi(AssetsDao assetsDao) {
this.assetsDao = assetsDao;
}
/**
* For now it handles only requests to find with these $and and $or
*
* {
* "query": {
* "$and": [
* {"$match": {"field": "type", "value": "asset", "mode": "keyword"}},
* {"$or": [
* {"$match": {"field": "name", "value": "PROT_ID1", "mode": "keyword"}},
* {"$match": {"field": "name", "value": "PROT_ID2", "mode": "keyword"}}
* ]
* }
* ]
* }
* }
*
*/
@PostMapping(value = "/search", consumes = SIGNALS_JSON_MIMETYPE)
SgMetaResponse>> search(@RequestBody SearchQuery query) {
List> result = new ArrayList<>();
Map mongoQuery = toMongoQuery(query.get());
assetsDao.find(new Document(mongoQuery)).forEach(doc -> {
Map fields = withOffsetDateTimeInsteadOfDate(doc);
result.add(new ListEntry<>(new SearchEntry(
(String) fields.get("_id"), (String) fields.get("type"),
(String) fields.get("name"), (String) fields.get("description"),
(OffsetDateTime) fields.get("createdAt"),
// Technically I think editedAt and modifiedAt are stored differently. Heard something about
// the search index having its own modifiedAt, and they may be out of sync. But for mocks
// it probably doesn't matter.
(OffsetDateTime) fields.get("editedAt")
)));
});
return new SgMetaResponse<>(result, Map.of("self", "TBD"), Map.of());
}
@PostMapping("/random-proteins")
public List createRandomProteins(int n) {
List result = new ArrayList<>();
for (int i = 0; i < n; i++)
result.add(assetsDao.save(TestObjectsFactory.protein()));
return result;
}
@SuppressWarnings("unchecked")
private static Map toMongoQuery(Map query) {
Map result = new HashMap<>();
for (Map.Entry e : query.entrySet()) {
if (e.getKey().equals("$match")) {
Map match = (Map) e.getValue();
result.put((String) match.get("field"), match.get("value"));
continue;
}
if (e.getValue() instanceof List) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy