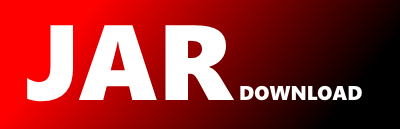
io.engineblock.planning.BucketSequencer Maven / Gradle / Ivy
/*
*
* Copyright 2016 jshook
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* /
*/
package io.engineblock.planning;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.function.ToLongFunction;
import java.util.stream.Collectors;
/**
* Introduction
* This class allows you to create a cyclic schedule that will maintain a mix
* of elements according to individual ratios. This particular sequencer
* simulates the effect of drawing from each bucket in round robin order until they
* are empty, putting each event into the planned sequence in turn.
*
* While this is not a good way to evenly interleave events over a large number,
* it is easy to reason about when looking at ratios like
* A:5,B:3,C:1. In this case, the order of events would be A B C A B A B A A.
#
* Explanation and Example
* Given a set of three events A, B, and C, and a set of respective frequencies
* of these events 5, 5, and 1. In short form: A:5,B:5,C:1. This means that a total
* of 11 events will be scheduled. With this sequencing algorithm, source buckets of
* events of type A, B, and C are initialized with the respective number of instances.
* Then, each bucket in turn is drawn from in round robin fashion, with each bucket
* being removed from the rotation when it becomes empty.
*
*
Further Examples
* These examples simply show in symbolic terms how the ordering is affected by
* different ratios.
*
* - X:1,Y:1,Z:1 - X Y Z
* - L:4,M:1 - L M L L L
* - A:4,B:3,C:2,D:1 - A B C D A B C A B A
* - A:1,B:2:C:3:D:4 - A B C D B C D C D D
* - D:4,C:3,B:2,A:1 - D C B A D C B D C D
*
*
* @param The element which is to be scheduled.
*/
public class BucketSequencer implements ElementSequencer {
private List elems;
private ToLongFunction ratioFunc;
@Override
public int[] sequenceByIndex(List elems, ToLongFunction ratioFunc) {
List> buckets = new ArrayList<>();
List sequence = new ArrayList<>();
for (int i = 0; i < elems.size(); i++) {
T elem = elems.get(i);
buckets.add(new OpBucket<>(elem,i,ratioFunc.applyAsLong(elem)));
}
while(!buckets.isEmpty()) {
buckets.forEach(b -> sequence.add(b.dispenseRank()));
buckets = buckets.stream().filter(b -> b.count>0).collect(Collectors.toCollection(LinkedList::new));
}
return sequence.stream().mapToInt(i -> (int)i).toArray();
}
private final static class OpBucket {
private final int rank;
private T elem;
private long count;
OpBucket(T elem, int rank, long ratio) {
this.elem = elem;
this.rank = rank;
this.count = ratio;
}
public boolean isEmpty() {
return (count==0);
}
int dispenseRank() {
count--;
return rank;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy