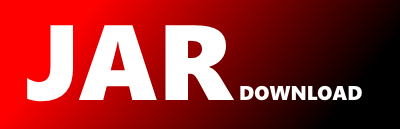
commonMain.io.eqoty.dapp.secret.utils.DeployContractUtils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deploy-utils-jvm Show documentation
Show all versions of deploy-utils-jvm Show documentation
A Kotlin multiplatform REST client utilizing secret network's gRPC gateway endpoints.
import io.eqoty.cosmwasm.std.types.CodeInfo
import io.eqoty.dapp.secret.types.ContractInstance
import io.eqoty.dapp.secret.utils.fileSystem
import io.eqoty.dapp.secret.utils.logger
import io.eqoty.secretk.client.SigningCosmWasmClient
import io.eqoty.secretk.types.MsgInstantiateContract
import io.eqoty.secretk.types.MsgStoreCode
import io.eqoty.secretk.types.TxOptions
import okio.Path
object DeployContractUtils {
private val storedCode = mutableMapOf()
suspend fun getOrStoreCode(
client: SigningCosmWasmClient,
contractCodePath: Path
): CodeInfo {
val wasmBytes =
fileSystem.read(contractCodePath) {
readByteArray()
}
return storedCode.getOrPut(wasmBytes.contentHashCode()) {
storeCode(client, wasmBytes)
}
}
suspend fun storeCode(
client: SigningCosmWasmClient,
contractCodePath: Path
): CodeInfo {
val wasmBytes =
fileSystem.read(contractCodePath) {
readByteArray()
}
return storeCode(client, wasmBytes)
}
private suspend fun storeCode(
client: SigningCosmWasmClient,
wasmBytes: ByteArray
): CodeInfo {
val msgs0 = listOf(
MsgStoreCode(
sender = client.senderAddress,
wasmByteCode = wasmBytes.toUByteArray(),
)
)
val simulate = client.simulate(msgs0)
val gasLimit = (simulate.gasUsed.toDouble() * 1.1).toInt()
val response = client.execute(
msgs0,
txOptions = TxOptions(gasLimit = gasLimit)
)
val codeId = response.logs[0].events
.find { it.type == "message" }
?.attributes
?.find { it.key == "code_id" }?.value!!
logger.i("codeId: $codeId")
return client.getCodeInfoByCodeId(codeId)
}
suspend fun instantiateCode(
client: SigningCosmWasmClient,
codeInfo: CodeInfo,
instantiateMsgs: List,
): ContractInstance {
instantiateMsgs.forEach {
it.codeId = codeInfo.codeId.toInt()
it.codeHash = codeInfo.codeHash
}
val simulate = client.simulate(instantiateMsgs)
val gasLimit = (simulate.gasUsed.toDouble() * 1.1).toInt()
val instantiateResponse = client.execute(
instantiateMsgs,
txOptions = TxOptions(gasLimit = gasLimit)
)
val contractAddress = instantiateResponse.logs[0].events
.find { it.type == "message" }
?.attributes
?.find { it.key == "contract_address" }?.value!!
logger.i("contract address: $contractAddress")
return ContractInstance(codeInfo, contractAddress)
}
suspend fun getOrStoreCodeAndInstantiate(
client: SigningCosmWasmClient,
codePath: Path,
instantiateMsgs: List,
): ContractInstance {
val codeInfo = getOrStoreCode(client, codePath)
return instantiateCode(client, codeInfo, instantiateMsgs)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy