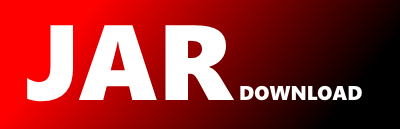
io.etcd.jetcd.api.VertxElectionGrpc Maven / Gradle / Ivy
package io.etcd.jetcd.api;
import static io.etcd.jetcd.api.ElectionGrpc.getServiceDescriptor;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
@javax.annotation.Generated(
value = "by VertxGrpc generator",
comments = "Source: election.proto")
public final class VertxElectionGrpc {
private VertxElectionGrpc() {}
public static ElectionVertxStub newVertxStub(io.grpc.Channel channel) {
return new ElectionVertxStub(channel);
}
/**
*
* The election service exposes client-side election facilities as a gRPC interface.
*
*/
public static final class ElectionVertxStub extends io.grpc.stub.AbstractStub {
private final io.vertx.core.impl.ContextInternal ctx;
private ElectionGrpc.ElectionStub delegateStub;
private ElectionVertxStub(io.grpc.Channel channel) {
super(channel);
delegateStub = ElectionGrpc.newStub(channel);
this.ctx = (io.vertx.core.impl.ContextInternal) io.vertx.core.Vertx.currentContext();
}
private ElectionVertxStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
delegateStub = ElectionGrpc.newStub(channel).build(channel, callOptions);
this.ctx = (io.vertx.core.impl.ContextInternal) io.vertx.core.Vertx.currentContext();
}
@Override
protected ElectionVertxStub build(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ElectionVertxStub(channel, callOptions);
}
/**
*
* Campaign waits to acquire leadership in an election, returning a LeaderKey
* representing the leadership if successful. The LeaderKey can then be used
* to issue new values on the election, transactionally guard API requests on
* leadership still being held, and resign from the election.
*
*/
public io.vertx.core.Future campaign(io.etcd.jetcd.api.CampaignRequest request) {
return io.vertx.grpc.stub.ClientCalls.oneToOne(ctx, request, delegateStub::campaign);
}
/**
*
* Proclaim updates the leader's posted value with a new value.
*
*/
public io.vertx.core.Future proclaim(io.etcd.jetcd.api.ProclaimRequest request) {
return io.vertx.grpc.stub.ClientCalls.oneToOne(ctx, request, delegateStub::proclaim);
}
/**
*
* Leader returns the current election proclamation, if any.
*
*/
public io.vertx.core.Future leader(io.etcd.jetcd.api.LeaderRequest request) {
return io.vertx.grpc.stub.ClientCalls.oneToOne(ctx, request, delegateStub::leader);
}
/**
*
* Resign releases election leadership so other campaigners may acquire
* leadership on the election.
*
*/
public io.vertx.core.Future resign(io.etcd.jetcd.api.ResignRequest request) {
return io.vertx.grpc.stub.ClientCalls.oneToOne(ctx, request, delegateStub::resign);
}
/**
*
* Observe streams election proclamations in-order as made by the election's
* elected leaders.
*
*/
public io.vertx.core.streams.ReadStream observe(io.etcd.jetcd.api.LeaderRequest request) {
return io.vertx.grpc.stub.ClientCalls.oneToMany(ctx, request, delegateStub::observe);
}
public io.vertx.core.streams.ReadStream observeWithHandler(io.etcd.jetcd.api.LeaderRequest request, io.vertx.core.Handler handler, io.vertx.core.Handler endHandler, io.vertx.core.Handler exceptionHandler) {
return io.vertx.grpc.stub.ClientCalls.oneToMany(ctx, request, delegateStub::observe, handler, endHandler, exceptionHandler);
}
}
/**
*
* The election service exposes client-side election facilities as a gRPC interface.
*
*/
public static abstract class ElectionVertxImplBase implements io.grpc.BindableService {
private String compression;
/**
* Set whether the server will try to use a compressed response.
*
* @param compression the compression, e.g {@code gzip}
*/
public ElectionVertxImplBase withCompression(String compression) {
this.compression = compression;
return this;
}
/**
*
* Campaign waits to acquire leadership in an election, returning a LeaderKey
* representing the leadership if successful. The LeaderKey can then be used
* to issue new values on the election, transactionally guard API requests on
* leadership still being held, and resign from the election.
*
*/
public io.vertx.core.Future campaign(io.etcd.jetcd.api.CampaignRequest request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Proclaim updates the leader's posted value with a new value.
*
*/
public io.vertx.core.Future proclaim(io.etcd.jetcd.api.ProclaimRequest request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Leader returns the current election proclamation, if any.
*
*/
public io.vertx.core.Future leader(io.etcd.jetcd.api.LeaderRequest request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Resign releases election leadership so other campaigners may acquire
* leadership on the election.
*
*/
public io.vertx.core.Future resign(io.etcd.jetcd.api.ResignRequest request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Observe streams election proclamations in-order as made by the election's
* elected leaders.
*
*/
public void observe(io.etcd.jetcd.api.LeaderRequest request, io.vertx.core.streams.WriteStream response) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
io.etcd.jetcd.api.ElectionGrpc.getCampaignMethod(),
asyncUnaryCall(
new MethodHandlers<
io.etcd.jetcd.api.CampaignRequest,
io.etcd.jetcd.api.CampaignResponse>(
this, METHODID_CAMPAIGN, compression)))
.addMethod(
io.etcd.jetcd.api.ElectionGrpc.getProclaimMethod(),
asyncUnaryCall(
new MethodHandlers<
io.etcd.jetcd.api.ProclaimRequest,
io.etcd.jetcd.api.ProclaimResponse>(
this, METHODID_PROCLAIM, compression)))
.addMethod(
io.etcd.jetcd.api.ElectionGrpc.getLeaderMethod(),
asyncUnaryCall(
new MethodHandlers<
io.etcd.jetcd.api.LeaderRequest,
io.etcd.jetcd.api.LeaderResponse>(
this, METHODID_LEADER, compression)))
.addMethod(
io.etcd.jetcd.api.ElectionGrpc.getObserveMethod(),
asyncServerStreamingCall(
new MethodHandlers<
io.etcd.jetcd.api.LeaderRequest,
io.etcd.jetcd.api.LeaderResponse>(
this, METHODID_OBSERVE, compression)))
.addMethod(
io.etcd.jetcd.api.ElectionGrpc.getResignMethod(),
asyncUnaryCall(
new MethodHandlers<
io.etcd.jetcd.api.ResignRequest,
io.etcd.jetcd.api.ResignResponse>(
this, METHODID_RESIGN, compression)))
.build();
}
}
private static final int METHODID_CAMPAIGN = 0;
private static final int METHODID_PROCLAIM = 1;
private static final int METHODID_LEADER = 2;
private static final int METHODID_OBSERVE = 3;
private static final int METHODID_RESIGN = 4;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final ElectionVertxImplBase serviceImpl;
private final int methodId;
private final String compression;
MethodHandlers(ElectionVertxImplBase serviceImpl, int methodId, String compression) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
this.compression = compression;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_CAMPAIGN:
io.vertx.grpc.stub.ServerCalls.oneToOne(
(io.etcd.jetcd.api.CampaignRequest) request,
(io.grpc.stub.StreamObserver) responseObserver,
compression,
serviceImpl::campaign);
break;
case METHODID_PROCLAIM:
io.vertx.grpc.stub.ServerCalls.oneToOne(
(io.etcd.jetcd.api.ProclaimRequest) request,
(io.grpc.stub.StreamObserver) responseObserver,
compression,
serviceImpl::proclaim);
break;
case METHODID_LEADER:
io.vertx.grpc.stub.ServerCalls.oneToOne(
(io.etcd.jetcd.api.LeaderRequest) request,
(io.grpc.stub.StreamObserver) responseObserver,
compression,
serviceImpl::leader);
break;
case METHODID_OBSERVE:
io.vertx.grpc.stub.ServerCalls.oneToMany(
(io.etcd.jetcd.api.LeaderRequest) request,
(io.grpc.stub.StreamObserver) responseObserver,
compression,
serviceImpl::observe);
break;
case METHODID_RESIGN:
io.vertx.grpc.stub.ServerCalls.oneToOne(
(io.etcd.jetcd.api.ResignRequest) request,
(io.grpc.stub.StreamObserver) responseObserver,
compression,
serviceImpl::resign);
break;
default:
throw new java.lang.AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new java.lang.AssertionError();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy