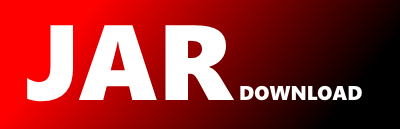
io.everitoken.sdk.java.dto.TransactionDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chain-sdk Show documentation
Show all versions of chain-sdk Show documentation
Official Java SDK for everiToken public chain. https://www.everitoken.io
package io.everitoken.sdk.java.dto;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONException;
import com.alibaba.fastjson.JSONObject;
import com.alibaba.fastjson.annotation.JSONField;
import org.jetbrains.annotations.Contract;
import org.jetbrains.annotations.NotNull;
import io.everitoken.sdk.java.Signature;
public class TransactionDetail {
private final int blockNum;
private final String packedTrx;
private final String id;
private final String compression;
private final List signatures = new ArrayList<>();
private final JSONObject transaction;
private final String blockId;
private TransactionDetail(@NotNull JSONObject raw) throws JSONException {
blockNum = raw.getInteger("block_num");
packedTrx = raw.getString("packed_trx");
id = raw.getString("id");
compression = raw.getString("compression");
JSONArray signaturesArray = raw.getJSONArray("signatures");
for (int i = 0; i < signaturesArray.size(); i++) {
signatures.add(Signature.of((String) signaturesArray.get(i)));
}
transaction = raw.getJSONObject("transaction");
blockId = raw.getString("block_id");
}
@NotNull
@Contract("_ -> new")
public static TransactionDetail create(JSONObject raw) throws JSONException {
Objects.requireNonNull(raw);
return new TransactionDetail(raw);
}
@JSONField(name = "block_num")
public int getBlockNum() {
return blockNum;
}
@JSONField(name = "packed_trx")
public String getPackedTrx() {
return packedTrx;
}
public String getId() {
return id;
}
public String getCompression() {
return compression;
}
public List getSignatures() {
return signatures;
}
public JSONObject getTransaction() {
return transaction;
}
@JSONField(name = "block_id")
public String getBlockId() {
return blockId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy