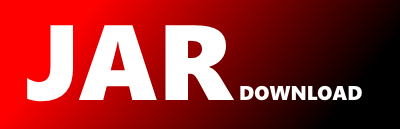
org.assertj.assertions.generator.description.GetterDescription Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* Copyright 2012-2015 the original author or authors.
*/
package org.assertj.assertions.generator.description;
import java.util.ArrayList;
import java.util.List;
import org.assertj.assertions.generator.util.ClassUtil;
/**
* Stores the information needed to generate an assertion for a getter method.
*
* Let's say we have the following method in class Person
:
*
*
* public int getAge()
*
*
* To generate PersonAssert
hasAge(int expectedAge)
assertion in PersonAssert
, we
* need to know :
*
* - the property name, here "age"
* - property type
*
* Note that Person
doesn't need to have an age
field, just the getAge
method.
*
*
* @author Joel Costigliola
*
*/
public class GetterDescription extends DataDescription implements Comparable {
private final List exceptions;
public GetterDescription(String propertyName, String origMethodName, TypeDescription typeDescription,
List exceptions) {
super(propertyName, origMethodName, typeDescription);
this.exceptions = new ArrayList(exceptions);
}
public String getPropertyName() {
return getName();
}
@Override
public int compareTo(GetterDescription other) {
return getOriginalMember().compareTo(other.getOriginalMember());
}
@Override
public String toString() {
return "GetterDescription [propertyName=" + getName() + ", typeDescription=" + typeDescription + "]";
}
public List getExceptions() {
return exceptions;
}
@Override
public boolean isPredicate() {
return typeDescription.isBoolean() && ClassUtil.isValidPredicateName(originalMember);
}
}