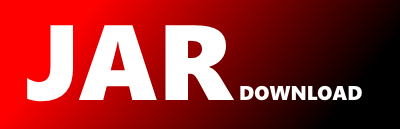
io.fabric8.runtime.itests.support.Provision Maven / Gradle / Ivy
/**
* Copyright 2005-2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version
* 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package io.fabric8.runtime.itests.support;
import io.fabric8.api.Container;
import io.fabric8.api.FabricService;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.CompletionService;
import java.util.concurrent.ExecutorCompletionService;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import javax.management.JMX;
import javax.management.MBeanServerConnection;
import javax.management.ObjectName;
import javax.management.remote.JMXConnector;
import javax.management.remote.JMXConnectorFactory;
import javax.management.remote.JMXServiceURL;
import org.jboss.gravia.runtime.ModuleContext;
import org.jboss.gravia.runtime.RuntimeLocator;
public class Provision {
private static final ExecutorService EXECUTOR = Executors.newCachedThreadPool();
/**
* Waits for all container to provision and reach the specified status.
*/
public static void containersStatus(Collection containers, String status, Long timeout) throws Exception {
CompletionService completionService = new ExecutorCompletionService(EXECUTOR);
List> waitForProvisionTasks = new LinkedList>();
StringBuilder sb = new StringBuilder();
sb.append(" ");
for (Container c : containers) {
waitForProvisionTasks.add(completionService.submit(new WaitForProvisionTask(c, status, timeout)));
sb.append(c.getId()).append(" ");
}
System.out.println("Waiting for containers: [" + sb.toString() + "] to successfully provision");
for (int i = 0; i < containers.size(); i++) {
completionService.poll(timeout, TimeUnit.MILLISECONDS);
}
}
/**
* Wait for all container provision successfully provision and reach status success.
*/
public static void containerStatus(Collection containers, Long timeout) throws Exception {
containersStatus(containers, "success", timeout);
}
/**
* Wait for all containers to become alive.
*/
public static void containersAlive(Collection containers, boolean alive, Long timeout) throws Exception {
if (containers.isEmpty()) {
return;
}
CompletionService completionService = new ExecutorCompletionService(EXECUTOR);
List> waitForProvisionTasks = new LinkedList>();
StringBuilder sb = new StringBuilder();
sb.append(" ");
for (Container container : containers) {
waitForProvisionTasks.add(completionService.submit(new WaitForAliveTask(container, alive, timeout)));
sb.append(container.getId()).append(" ");
}
System.out.println("Waiting for containers: [" + sb.toString() + "] to reach Alive:"+alive);
for (Container container : containers) {
Future f = completionService.poll(timeout, TimeUnit.MILLISECONDS);
if ( f == null || !f.get()) {
throw new Exception("Container " + container.getId() + " failed to reach Alive:" + alive);
}
}
}
/**
* Wait for a condition to become satisfied.
*/
public static boolean waitForCondition(Callable condition, Long timeout) throws Exception {
Future result = EXECUTOR.submit(condition);
return result.get(timeout, TimeUnit.MILLISECONDS);
}
public static boolean waitForCondition(WaitForConditionTask task) throws Exception {
Future result = EXECUTOR.submit(task);
return result.get();
}
/**
* Wait for a condition to become satisfied.
*/
public static boolean waitForCondition(final Collection containers, final ContainerCondition condition, Long timeout) throws Exception {
CompletionService completionService = new ExecutorCompletionService(EXECUTOR);
for (final Container container : containers) {
completionService.submit(
new WaitForConditionTask(new Callable() {
@Override
public Object call() throws Exception {
return condition.checkConditionOnContainer(container);
}
}, timeout));
}
for (Container container : containers) {
Future f = completionService.poll(timeout, TimeUnit.MILLISECONDS);
if (f == null || !f.get()) {
return false;
}
}
return true;
}
/**
* Wait for all containers to become registered.
*/
public static void containersExist(Collection containers, Long timeout) throws Exception {
CompletionService completionService = new ExecutorCompletionService(EXECUTOR);
List> waitForProvisionTasks = new LinkedList>();
StringBuilder sb = new StringBuilder();
sb.append(" ");
for (String container : containers) {
waitForProvisionTasks.add(completionService.submit(new WaitForContainerCreationTask(container, timeout)));
sb.append(container).append(" ");
}
System.out.println("Waiting for containers: [" + sb.toString() + "] to become created.");
for (String container : containers) {
Future f = completionService.poll(timeout, TimeUnit.MILLISECONDS);
if ( f == null || !f.get()) {
throw new Exception("Container " + container + " failed to become created.");
}
}
}
/**
* Wait for all containers to become registered.
*/
public static void instanceStarted(Collection instances, Long timeout) throws Exception {
CompletionService completionService = new ExecutorCompletionService(EXECUTOR);
List> waitForstarted = new LinkedList>();
StringBuilder sb = new StringBuilder();
sb.append(" ");
for (String instance : instances) {
waitForstarted.add(completionService.submit(new WaitForInstanceStartedTask(instance, timeout)));
sb.append(instance).append(" ");
}
System.out.println("Waiting for child instances: [" + sb.toString() + "] to get started.");
for (String instance : instances) {
Future f = completionService.poll(timeout, TimeUnit.MILLISECONDS);
if ( f == null || !f.get()) {
throw new Exception("Instance " + instance + " failed to start.");
}
}
}
/**
* Wait for all containers to become alive.
*/
public static void containerAlive(Collection containers, Long timeout) throws Exception {
containersAlive(containers, true, timeout);
}
/**
* Wait for a container to provision and assert its status.
*/
public static void provisioningSuccess(Collection containers, Long timeout) throws Exception {
if (containers.isEmpty()) {
return;
}
boolean running = true;
long startedAt = System.currentTimeMillis();
long remaining = timeout;
while (running && !Thread.interrupted()) {
containerStatus(containers, remaining);
remaining = timeout + startedAt - System.currentTimeMillis();
for (Container container : containers) {
if (!container.isAliveAndOK()) {
if(container.getProvisionException() != null) {
throw new Exception(container.getProvisionException());
}
else if (startedAt + timeout < System.currentTimeMillis()) {
throw new Exception("Container " + container.getId() + " failed to provision. Status:" + container.getProvisionStatus() + " Exception:" + container.getProvisionException());
}
} else {
running = false;
}
}
}
}
public static Boolean profileAvailable(String profile, String version, Long timeout) throws Exception {
ModuleContext moduleContext = RuntimeLocator.getRequiredRuntime().getModuleContext();
FabricService service = ServiceLocator.awaitService(moduleContext, FabricService.class);
for (long t = 0; (!service.getDataStore().hasProfile(version, profile) && t < timeout); t += 2000L) {
Thread.sleep(2000L);
}
return service.getDataStore().hasProfile(version, profile);
}
public static Object getMBean(final Container container, final ObjectName mbeanName, final Class clazz, final long timeout) throws Exception {
CompletionService
© 2015 - 2025 Weber Informatics LLC | Privacy Policy