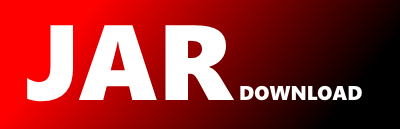
annotations.io.fabric8.kubernetes.api.model.ContainerStatusFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.HashMap;
import java.util.Map;
import io.fabric8.common.Nested;
import io.fabric8.common.Builder;
import io.fabric8.common.Fluent;
import io.fabric8.common.BaseFluent;
import io.fabric8.common.Visitable;
public class ContainerStatusFluent> extends BaseFluent implements Fluent{
String containerID; String image; String imageID; ContainerStateBuilder lastState; String name; Boolean ready; Integer restartCount; ContainerStateBuilder state; Map additionalProperties = new HashMap();
public String getContainerID(){
return this.containerID;
}
public T withContainerID( String containerID){
this.containerID=containerID; return (T) this;
}
public String getImage(){
return this.image;
}
public T withImage( String image){
this.image=image; return (T) this;
}
public String getImageID(){
return this.imageID;
}
public T withImageID( String imageID){
this.imageID=imageID; return (T) this;
}
public ContainerState getLastState(){
return this.lastState!=null?this.lastState.build():null;
}
public T withLastState( ContainerState lastState){
if (lastState!=null){ this.lastState= new ContainerStateBuilder(lastState); _visitables.add(this.lastState);} return (T) this;
}
public LastStateNested withNewLastState(){
return new LastStateNested();
}
public String getName(){
return this.name;
}
public T withName( String name){
this.name=name; return (T) this;
}
public Boolean isReady(){
return this.ready;
}
public T withReady( Boolean ready){
this.ready=ready; return (T) this;
}
public Integer getRestartCount(){
return this.restartCount;
}
public T withRestartCount( Integer restartCount){
this.restartCount=restartCount; return (T) this;
}
public ContainerState getState(){
return this.state!=null?this.state.build():null;
}
public T withState( ContainerState state){
if (state!=null){ this.state= new ContainerStateBuilder(state); _visitables.add(this.state);} return (T) this;
}
public StateNested withNewState(){
return new StateNested();
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public class LastStateNested extends ContainerStateFluent> implements Nested{
private final ContainerStateBuilder builder = new ContainerStateBuilder(this);
public N endLastState(){
return and();
}
public N and(){
return (N) ContainerStatusFluent.this.withLastState(builder.build());
}
}
public class StateNested extends ContainerStateFluent> implements Nested{
private final ContainerStateBuilder builder = new ContainerStateBuilder(this);
public N endState(){
return and();
}
public N and(){
return (N) ContainerStatusFluent.this.withState(builder.build());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy