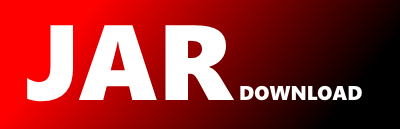
annotations.io.fabric8.openshift.api.model.DeploymentConfigSpecFluent Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.model.ObjectReference;
import java.util.HashMap;
import java.util.Map;
import java.util.List;
import java.util.ArrayList;
import io.fabric8.kubernetes.api.model.PodTemplateSpec;
import io.fabric8.common.Nested;
import io.fabric8.kubernetes.api.model.PodTemplateSpecBuilder;
import io.fabric8.common.Builder;
import io.fabric8.common.Fluent;
import io.fabric8.common.Visitable;
import io.fabric8.kubernetes.api.model.PodTemplateSpecFluent;
import io.fabric8.kubernetes.api.model.ObjectReferenceFluent;
import io.fabric8.common.BaseFluent;
import io.fabric8.kubernetes.api.model.ObjectReferenceBuilder;
public class DeploymentConfigSpecFluent> extends BaseFluent implements Fluent{
Integer replicas; Map selector = new HashMap(); DeploymentStrategyBuilder strategy; PodTemplateSpecBuilder template; ObjectReferenceBuilder templateRef; List triggers = new ArrayList(); Map additionalProperties = new HashMap();
public Integer getReplicas(){
return this.replicas;
}
public T withReplicas( Integer replicas){
this.replicas=replicas; return (T) this;
}
public T addToSelector( String key, String value){
if(key != null && value != null) {this.selector.put(key, value);} return (T)this;
}
public Map getSelector(){
return this.selector;
}
public T withSelector( Map selector){
this.selector.clear();if (selector != null) {this.selector.putAll(selector);} return (T) this;
}
public DeploymentStrategy getStrategy(){
return this.strategy!=null?this.strategy.build():null;
}
public T withStrategy( DeploymentStrategy strategy){
if (strategy!=null){ this.strategy= new DeploymentStrategyBuilder(strategy); _visitables.add(this.strategy);} return (T) this;
}
public StrategyNested withNewStrategy(){
return new StrategyNested();
}
public PodTemplateSpec getTemplate(){
return this.template!=null?this.template.build():null;
}
public T withTemplate( PodTemplateSpec template){
if (template!=null){ this.template= new PodTemplateSpecBuilder(template); _visitables.add(this.template);} return (T) this;
}
public TemplateNested withNewTemplate(){
return new TemplateNested();
}
public ObjectReference getTemplateRef(){
return this.templateRef!=null?this.templateRef.build():null;
}
public T withTemplateRef( ObjectReference templateRef){
if (templateRef!=null){ this.templateRef= new ObjectReferenceBuilder(templateRef); _visitables.add(this.templateRef);} return (T) this;
}
public TemplateRefNested withNewTemplateRef(){
return new TemplateRefNested();
}
public T addToTriggers( DeploymentTriggerPolicy item){
if (item != null) {DeploymentTriggerPolicyBuilder builder = new DeploymentTriggerPolicyBuilder(item);_visitables.add(builder);this.triggers.add(builder);} return (T)this;
}
public List getTriggers(){
return build(triggers);
}
public T withTriggers( List triggers){
this.triggers.clear();if (triggers != null) {for (DeploymentTriggerPolicy item : triggers){this.addToTriggers(item);}} return (T) this;
}
public TriggersNested addNewTrigger(){
return new TriggersNested();
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public class StrategyNested extends DeploymentStrategyFluent> implements Nested{
private final DeploymentStrategyBuilder builder = new DeploymentStrategyBuilder(this);
public N and(){
return (N) DeploymentConfigSpecFluent.this.withStrategy(builder.build());
}
public N endStrategy(){
return and();
}
}
public class TemplateNested extends PodTemplateSpecFluent> implements Nested{
private final PodTemplateSpecBuilder builder = new PodTemplateSpecBuilder(this);
public N and(){
return (N) DeploymentConfigSpecFluent.this.withTemplate(builder.build());
}
public N endTemplate(){
return and();
}
}
public class TemplateRefNested extends ObjectReferenceFluent> implements Nested{
private final ObjectReferenceBuilder builder = new ObjectReferenceBuilder(this);
public N and(){
return (N) DeploymentConfigSpecFluent.this.withTemplateRef(builder.build());
}
public N endTemplateRef(){
return and();
}
}
public class TriggersNested extends DeploymentTriggerPolicyFluent> implements Nested{
private final DeploymentTriggerPolicyBuilder builder = new DeploymentTriggerPolicyBuilder(this);
public N and(){
return (N) DeploymentConfigSpecFluent.this.addToTriggers(builder.build());
}
public N endTrigger(){
return and();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy