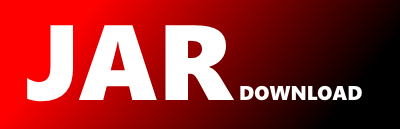
annotations.io.fabric8.openshift.api.model.DeploymentStrategyFluent Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import java.util.HashMap;
import java.util.Map;
import io.fabric8.common.Nested;
import io.fabric8.common.Builder;
import io.fabric8.kubernetes.api.model.ResourceRequirementsBuilder;
import io.fabric8.common.Fluent;
import io.fabric8.common.Visitable;
import io.fabric8.common.BaseFluent;
import io.fabric8.kubernetes.api.model.ResourceRequirementsFluent;
public class DeploymentStrategyFluent> extends BaseFluent implements Fluent{
CustomDeploymentStrategyParamsBuilder customParams; RecreateDeploymentStrategyParamsBuilder recreateParams; ResourceRequirementsBuilder resources; RollingDeploymentStrategyParams rollingParams; String type; Map additionalProperties = new HashMap();
public CustomDeploymentStrategyParams getCustomParams(){
return this.customParams!=null?this.customParams.build():null;
}
public T withCustomParams( CustomDeploymentStrategyParams customParams){
if (customParams!=null){ this.customParams= new CustomDeploymentStrategyParamsBuilder(customParams); _visitables.add(this.customParams);} return (T) this;
}
public CustomParamsNested withNewCustomParams(){
return new CustomParamsNested();
}
public RecreateDeploymentStrategyParams getRecreateParams(){
return this.recreateParams!=null?this.recreateParams.build():null;
}
public T withRecreateParams( RecreateDeploymentStrategyParams recreateParams){
if (recreateParams!=null){ this.recreateParams= new RecreateDeploymentStrategyParamsBuilder(recreateParams); _visitables.add(this.recreateParams);} return (T) this;
}
public RecreateParamsNested withNewRecreateParams(){
return new RecreateParamsNested();
}
public ResourceRequirements getResources(){
return this.resources!=null?this.resources.build():null;
}
public T withResources( ResourceRequirements resources){
if (resources!=null){ this.resources= new ResourceRequirementsBuilder(resources); _visitables.add(this.resources);} return (T) this;
}
public ResourcesNested withNewResources(){
return new ResourcesNested();
}
public RollingDeploymentStrategyParams getRollingParams(){
return this.rollingParams;
}
public T withRollingParams( RollingDeploymentStrategyParams rollingParams){
this.rollingParams=rollingParams; return (T) this;
}
public String getType(){
return this.type;
}
public T withType( String type){
this.type=type; return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public class CustomParamsNested extends CustomDeploymentStrategyParamsFluent> implements Nested{
private final CustomDeploymentStrategyParamsBuilder builder = new CustomDeploymentStrategyParamsBuilder(this);
public N endCustomParams(){
return and();
}
public N and(){
return (N) DeploymentStrategyFluent.this.withCustomParams(builder.build());
}
}
public class RecreateParamsNested extends RecreateDeploymentStrategyParamsFluent> implements Nested{
private final RecreateDeploymentStrategyParamsBuilder builder = new RecreateDeploymentStrategyParamsBuilder(this);
public N endRecreateParams(){
return and();
}
public N and(){
return (N) DeploymentStrategyFluent.this.withRecreateParams(builder.build());
}
}
public class ResourcesNested extends ResourceRequirementsFluent> implements Nested{
private final ResourceRequirementsBuilder builder = new ResourceRequirementsBuilder(this);
public N endResources(){
return and();
}
public N and(){
return (N) DeploymentStrategyFluent.this.withResources(builder.build());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy