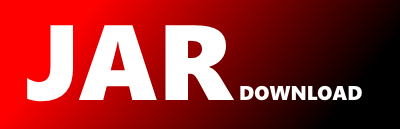
io.fabric8.kubernetes.api.model.KubeSchemaBuilder Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import javax.validation.ConstraintViolation;
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import javax.validation.ValidationException;
import java.util.Set;
import io.fabric8.openshift.api.model.ImageList;
import io.fabric8.openshift.api.model.ImageStreamList;
import io.fabric8.kubernetes.api.model.resource.Quantity;
import io.fabric8.openshift.api.model.OAuthClient;
import java.util.Map;
import io.fabric8.openshift.api.model.OAuthAccessTokenList;
import io.fabric8.openshift.api.model.OAuthAuthorizeToken;
import io.fabric8.openshift.api.model.template.Template;
import io.fabric8.openshift.api.model.BuildList;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenList;
import io.fabric8.openshift.api.model.RouteList;
import io.fabric8.openshift.api.model.TagEvent;
import java.util.HashMap;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationList;
import io.fabric8.openshift.api.model.OAuthAccessToken;
import io.fabric8.openshift.api.model.OAuthClientList;
import io.fabric8.kubernetes.api.model.errors.StatusError;
import io.fabric8.openshift.api.model.OAuthClientAuthorization;
import io.fabric8.openshift.api.model.BuildConfigList;
import io.fabric8.openshift.api.model.DeploymentConfigList;
import io.fabric8.common.Builder;
public class KubeSchemaBuilder extends KubeSchemaFluent implements Builder{
KubeSchemaFluent> fluent;
public KubeSchemaBuilder(){
this(new KubeSchema());
}
public KubeSchemaBuilder( KubeSchemaFluent> fluent ){
this(fluent, new KubeSchema());
}
public KubeSchemaBuilder( KubeSchemaFluent> fluent , KubeSchema instance ){
this.fluent = fluent; fluent.withBaseKubernetesList(instance.getBaseKubernetesList()); fluent.withBuildConfigList(instance.getBuildConfigList()); fluent.withBuildList(instance.getBuildList()); fluent.withContainerStatus(instance.getContainerStatus()); fluent.withDeploymentConfigList(instance.getDeploymentConfigList()); fluent.withEndpoints(instance.getEndpoints()); fluent.withEndpointsList(instance.getEndpointsList()); fluent.withEnvVar(instance.getEnvVar()); fluent.withImageList(instance.getImageList()); fluent.withImageStreamList(instance.getImageStreamList()); fluent.withNamespace(instance.getNamespace()); fluent.withNamespaceList(instance.getNamespaceList()); fluent.withNode(instance.getNode()); fluent.withNodeList(instance.getNodeList()); fluent.withOAuthAccessToken(instance.getOAuthAccessToken()); fluent.withOAuthAccessTokenList(instance.getOAuthAccessTokenList()); fluent.withOAuthAuthorizeToken(instance.getOAuthAuthorizeToken()); fluent.withOAuthAuthorizeTokenList(instance.getOAuthAuthorizeTokenList()); fluent.withOAuthClient(instance.getOAuthClient()); fluent.withOAuthClientAuthorization(instance.getOAuthClientAuthorization()); fluent.withOAuthClientAuthorizationList(instance.getOAuthClientAuthorizationList()); fluent.withOAuthClientList(instance.getOAuthClientList()); fluent.withObjectMeta(instance.getObjectMeta()); fluent.withPodList(instance.getPodList()); fluent.withQuantity(instance.getQuantity()); fluent.withReplicationControllerList(instance.getReplicationControllerList()); fluent.withRouteList(instance.getRouteList()); fluent.withSecret(instance.getSecret()); fluent.withSecretList(instance.getSecretList()); fluent.withServiceList(instance.getServiceList()); fluent.withStatusError(instance.getStatusError()); fluent.withTagEvent(instance.getTagEvent()); fluent.withTemplate(instance.getTemplate());
}
public KubeSchemaBuilder( KubeSchema instance ){
this.fluent = this; this.withBaseKubernetesList(instance.getBaseKubernetesList()); this.withBuildConfigList(instance.getBuildConfigList()); this.withBuildList(instance.getBuildList()); this.withContainerStatus(instance.getContainerStatus()); this.withDeploymentConfigList(instance.getDeploymentConfigList()); this.withEndpoints(instance.getEndpoints()); this.withEndpointsList(instance.getEndpointsList()); this.withEnvVar(instance.getEnvVar()); this.withImageList(instance.getImageList()); this.withImageStreamList(instance.getImageStreamList()); this.withNamespace(instance.getNamespace()); this.withNamespaceList(instance.getNamespaceList()); this.withNode(instance.getNode()); this.withNodeList(instance.getNodeList()); this.withOAuthAccessToken(instance.getOAuthAccessToken()); this.withOAuthAccessTokenList(instance.getOAuthAccessTokenList()); this.withOAuthAuthorizeToken(instance.getOAuthAuthorizeToken()); this.withOAuthAuthorizeTokenList(instance.getOAuthAuthorizeTokenList()); this.withOAuthClient(instance.getOAuthClient()); this.withOAuthClientAuthorization(instance.getOAuthClientAuthorization()); this.withOAuthClientAuthorizationList(instance.getOAuthClientAuthorizationList()); this.withOAuthClientList(instance.getOAuthClientList()); this.withObjectMeta(instance.getObjectMeta()); this.withPodList(instance.getPodList()); this.withQuantity(instance.getQuantity()); this.withReplicationControllerList(instance.getReplicationControllerList()); this.withRouteList(instance.getRouteList()); this.withSecret(instance.getSecret()); this.withSecretList(instance.getSecretList()); this.withServiceList(instance.getServiceList()); this.withStatusError(instance.getStatusError()); this.withTagEvent(instance.getTagEvent()); this.withTemplate(instance.getTemplate());
}
public KubeSchema build(){
KubeSchema buildable = new KubeSchema(fluent.getBaseKubernetesList(),fluent.getBuildConfigList(),fluent.getBuildList(),fluent.getContainerStatus(),fluent.getDeploymentConfigList(),fluent.getEndpoints(),fluent.getEndpointsList(),fluent.getEnvVar(),fluent.getImageList(),fluent.getImageStreamList(),fluent.getNamespace(),fluent.getNamespaceList(),fluent.getNode(),fluent.getNodeList(),fluent.getOAuthAccessToken(),fluent.getOAuthAccessTokenList(),fluent.getOAuthAuthorizeToken(),fluent.getOAuthAuthorizeTokenList(),fluent.getOAuthClient(),fluent.getOAuthClientAuthorization(),fluent.getOAuthClientAuthorizationList(),fluent.getOAuthClientList(),fluent.getObjectMeta(),fluent.getPodList(),fluent.getQuantity(),fluent.getReplicationControllerList(),fluent.getRouteList(),fluent.getSecret(),fluent.getSecretList(),fluent.getServiceList(),fluent.getStatusError(),fluent.getTagEvent(),fluent.getTemplate());
validate(buildable);
return buildable;
}
private void validate(T item) {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
Set> violations = validator.validate(item);
if (!violations.isEmpty()) {
StringBuilder sb = new StringBuilder();
sb.append("Constraint Violations:\n");
for (ConstraintViolation violation : violations) {
sb.append("\t").append(violation.getRootBeanClass().getSimpleName()).append(" ").append(violation.getPropertyPath()).append(":").append(violation.getMessage()).append("\n");
}
throw new IllegalStateException(sb.toString());
}
} catch(ValidationException e) {
//ignore
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy