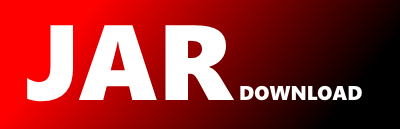
io.fabric8.kubernetes.api.model.KubeSchemaFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import io.fabric8.openshift.api.model.ImageList;
import io.fabric8.openshift.api.model.ImageStreamList;
import io.fabric8.kubernetes.api.model.resource.Quantity;
import io.fabric8.openshift.api.model.OAuthClient;
import java.util.Map;
import io.fabric8.openshift.api.model.OAuthAccessTokenList;
import io.fabric8.openshift.api.model.OAuthAuthorizeToken;
import io.fabric8.openshift.api.model.template.Template;
import io.fabric8.openshift.api.model.BuildList;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenList;
import io.fabric8.openshift.api.model.RouteList;
import io.fabric8.openshift.api.model.TagEvent;
import java.util.HashMap;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationList;
import io.fabric8.openshift.api.model.OAuthAccessToken;
import io.fabric8.openshift.api.model.OAuthClientList;
import io.fabric8.kubernetes.api.model.errors.StatusError;
import io.fabric8.openshift.api.model.OAuthClientAuthorization;
import io.fabric8.openshift.api.model.BuildConfigList;
import io.fabric8.openshift.api.model.DeploymentConfigList;
import io.fabric8.openshift.api.model.ImageStreamListBuilder;
import io.fabric8.common.Fluent;
import io.fabric8.common.Visitable;
import io.fabric8.openshift.api.model.ImageStreamListFluent;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenListFluent;
import io.fabric8.openshift.api.model.BuildConfigListFluent;
import io.fabric8.openshift.api.model.template.TemplateBuilder;
import io.fabric8.kubernetes.api.model.resource.QuantityBuilder;
import io.fabric8.openshift.api.model.OAuthClientBuilder;
import io.fabric8.openshift.api.model.OAuthAccessTokenBuilder;
import io.fabric8.openshift.api.model.OAuthAccessTokenListFluent;
import io.fabric8.openshift.api.model.DeploymentConfigListBuilder;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenListBuilder;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationBuilder;
import io.fabric8.openshift.api.model.OAuthClientListFluent;
import io.fabric8.openshift.api.model.RouteListFluent;
import io.fabric8.common.BaseFluent;
import io.fabric8.openshift.api.model.BuildListFluent;
import io.fabric8.openshift.api.model.BuildConfigListBuilder;
import io.fabric8.openshift.api.model.template.TemplateFluent;
import io.fabric8.openshift.api.model.OAuthAccessTokenFluent;
import io.fabric8.common.Nested;
import io.fabric8.openshift.api.model.ImageListBuilder;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenFluent;
import io.fabric8.openshift.api.model.BuildListBuilder;
import io.fabric8.common.Builder;
import io.fabric8.kubernetes.api.model.resource.QuantityFluent;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenBuilder;
import io.fabric8.openshift.api.model.ImageListFluent;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationListFluent;
import io.fabric8.openshift.api.model.TagEventBuilder;
import io.fabric8.openshift.api.model.OAuthClientListBuilder;
import io.fabric8.openshift.api.model.DeploymentConfigListFluent;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationListBuilder;
import io.fabric8.openshift.api.model.OAuthClientFluent;
import io.fabric8.openshift.api.model.OAuthAccessTokenListBuilder;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationFluent;
import io.fabric8.openshift.api.model.TagEventFluent;
import io.fabric8.openshift.api.model.RouteListBuilder;
public class KubeSchemaFluent> extends BaseFluent implements Fluent{
BaseKubernetesListBuilder BaseKubernetesList; BuildConfigListBuilder BuildConfigList; BuildListBuilder BuildList; ContainerStatusBuilder ContainerStatus; DeploymentConfigListBuilder DeploymentConfigList; EndpointsBuilder Endpoints; EndpointsListBuilder EndpointsList; EnvVarBuilder EnvVar; ImageListBuilder ImageList; ImageStreamListBuilder ImageStreamList; NamespaceBuilder Namespace; NamespaceListBuilder NamespaceList; NodeBuilder Node; NodeListBuilder NodeList; OAuthAccessTokenBuilder OAuthAccessToken; OAuthAccessTokenListBuilder OAuthAccessTokenList; OAuthAuthorizeTokenBuilder OAuthAuthorizeToken; OAuthAuthorizeTokenListBuilder OAuthAuthorizeTokenList; OAuthClientBuilder OAuthClient; OAuthClientAuthorizationBuilder OAuthClientAuthorization; OAuthClientAuthorizationListBuilder OAuthClientAuthorizationList; OAuthClientListBuilder OAuthClientList; ObjectMetaBuilder ObjectMeta; PodListBuilder PodList; QuantityBuilder Quantity; ReplicationControllerListBuilder ReplicationControllerList; RouteListBuilder RouteList; SecretBuilder Secret; SecretListBuilder SecretList; ServiceListBuilder ServiceList; StatusError StatusError; TagEventBuilder TagEvent; TemplateBuilder Template; Map additionalProperties = new HashMap();
public BaseKubernetesList getBaseKubernetesList(){
return this.BaseKubernetesList!=null?this.BaseKubernetesList.build():null;
}
public T withBaseKubernetesList( BaseKubernetesList BaseKubernetesList){
if (BaseKubernetesList!=null){ this.BaseKubernetesList= new BaseKubernetesListBuilder(BaseKubernetesList); _visitables.add(this.BaseKubernetesList);} return (T) this;
}
public BaseKubernetesListNested withNewBaseKubernetesList(){
return new BaseKubernetesListNested();
}
public BuildConfigList getBuildConfigList(){
return this.BuildConfigList!=null?this.BuildConfigList.build():null;
}
public T withBuildConfigList( BuildConfigList BuildConfigList){
if (BuildConfigList!=null){ this.BuildConfigList= new BuildConfigListBuilder(BuildConfigList); _visitables.add(this.BuildConfigList);} return (T) this;
}
public BuildConfigListNested withNewBuildConfigList(){
return new BuildConfigListNested();
}
public BuildList getBuildList(){
return this.BuildList!=null?this.BuildList.build():null;
}
public T withBuildList( BuildList BuildList){
if (BuildList!=null){ this.BuildList= new BuildListBuilder(BuildList); _visitables.add(this.BuildList);} return (T) this;
}
public BuildListNested withNewBuildList(){
return new BuildListNested();
}
public ContainerStatus getContainerStatus(){
return this.ContainerStatus!=null?this.ContainerStatus.build():null;
}
public T withContainerStatus( ContainerStatus ContainerStatus){
if (ContainerStatus!=null){ this.ContainerStatus= new ContainerStatusBuilder(ContainerStatus); _visitables.add(this.ContainerStatus);} return (T) this;
}
public ContainerStatusNested withNewContainerStatus(){
return new ContainerStatusNested();
}
public DeploymentConfigList getDeploymentConfigList(){
return this.DeploymentConfigList!=null?this.DeploymentConfigList.build():null;
}
public T withDeploymentConfigList( DeploymentConfigList DeploymentConfigList){
if (DeploymentConfigList!=null){ this.DeploymentConfigList= new DeploymentConfigListBuilder(DeploymentConfigList); _visitables.add(this.DeploymentConfigList);} return (T) this;
}
public DeploymentConfigListNested withNewDeploymentConfigList(){
return new DeploymentConfigListNested();
}
public Endpoints getEndpoints(){
return this.Endpoints!=null?this.Endpoints.build():null;
}
public T withEndpoints( Endpoints Endpoints){
if (Endpoints!=null){ this.Endpoints= new EndpointsBuilder(Endpoints); _visitables.add(this.Endpoints);} return (T) this;
}
public EndpointsNested withNewEndpoints(){
return new EndpointsNested();
}
public EndpointsList getEndpointsList(){
return this.EndpointsList!=null?this.EndpointsList.build():null;
}
public T withEndpointsList( EndpointsList EndpointsList){
if (EndpointsList!=null){ this.EndpointsList= new EndpointsListBuilder(EndpointsList); _visitables.add(this.EndpointsList);} return (T) this;
}
public EndpointsListNested withNewEndpointsList(){
return new EndpointsListNested();
}
public EnvVar getEnvVar(){
return this.EnvVar!=null?this.EnvVar.build():null;
}
public T withEnvVar( EnvVar EnvVar){
if (EnvVar!=null){ this.EnvVar= new EnvVarBuilder(EnvVar); _visitables.add(this.EnvVar);} return (T) this;
}
public EnvVarNested withNewEnvVar(){
return new EnvVarNested();
}
public ImageList getImageList(){
return this.ImageList!=null?this.ImageList.build():null;
}
public T withImageList( ImageList ImageList){
if (ImageList!=null){ this.ImageList= new ImageListBuilder(ImageList); _visitables.add(this.ImageList);} return (T) this;
}
public ImageListNested withNewImageList(){
return new ImageListNested();
}
public ImageStreamList getImageStreamList(){
return this.ImageStreamList!=null?this.ImageStreamList.build():null;
}
public T withImageStreamList( ImageStreamList ImageStreamList){
if (ImageStreamList!=null){ this.ImageStreamList= new ImageStreamListBuilder(ImageStreamList); _visitables.add(this.ImageStreamList);} return (T) this;
}
public ImageStreamListNested withNewImageStreamList(){
return new ImageStreamListNested();
}
public Namespace getNamespace(){
return this.Namespace!=null?this.Namespace.build():null;
}
public T withNamespace( Namespace Namespace){
if (Namespace!=null){ this.Namespace= new NamespaceBuilder(Namespace); _visitables.add(this.Namespace);} return (T) this;
}
public NamespaceNested withNewNamespace(){
return new NamespaceNested();
}
public NamespaceList getNamespaceList(){
return this.NamespaceList!=null?this.NamespaceList.build():null;
}
public T withNamespaceList( NamespaceList NamespaceList){
if (NamespaceList!=null){ this.NamespaceList= new NamespaceListBuilder(NamespaceList); _visitables.add(this.NamespaceList);} return (T) this;
}
public NamespaceListNested withNewNamespaceList(){
return new NamespaceListNested();
}
public Node getNode(){
return this.Node!=null?this.Node.build():null;
}
public T withNode( Node Node){
if (Node!=null){ this.Node= new NodeBuilder(Node); _visitables.add(this.Node);} return (T) this;
}
public NodeNested withNewNode(){
return new NodeNested();
}
public NodeList getNodeList(){
return this.NodeList!=null?this.NodeList.build():null;
}
public T withNodeList( NodeList NodeList){
if (NodeList!=null){ this.NodeList= new NodeListBuilder(NodeList); _visitables.add(this.NodeList);} return (T) this;
}
public NodeListNested withNewNodeList(){
return new NodeListNested();
}
public OAuthAccessToken getOAuthAccessToken(){
return this.OAuthAccessToken!=null?this.OAuthAccessToken.build():null;
}
public T withOAuthAccessToken( OAuthAccessToken OAuthAccessToken){
if (OAuthAccessToken!=null){ this.OAuthAccessToken= new OAuthAccessTokenBuilder(OAuthAccessToken); _visitables.add(this.OAuthAccessToken);} return (T) this;
}
public OAuthAccessTokenNested withNewOAuthAccessToken(){
return new OAuthAccessTokenNested();
}
public OAuthAccessTokenList getOAuthAccessTokenList(){
return this.OAuthAccessTokenList!=null?this.OAuthAccessTokenList.build():null;
}
public T withOAuthAccessTokenList( OAuthAccessTokenList OAuthAccessTokenList){
if (OAuthAccessTokenList!=null){ this.OAuthAccessTokenList= new OAuthAccessTokenListBuilder(OAuthAccessTokenList); _visitables.add(this.OAuthAccessTokenList);} return (T) this;
}
public OAuthAccessTokenListNested withNewOAuthAccessTokenList(){
return new OAuthAccessTokenListNested();
}
public OAuthAuthorizeToken getOAuthAuthorizeToken(){
return this.OAuthAuthorizeToken!=null?this.OAuthAuthorizeToken.build():null;
}
public T withOAuthAuthorizeToken( OAuthAuthorizeToken OAuthAuthorizeToken){
if (OAuthAuthorizeToken!=null){ this.OAuthAuthorizeToken= new OAuthAuthorizeTokenBuilder(OAuthAuthorizeToken); _visitables.add(this.OAuthAuthorizeToken);} return (T) this;
}
public OAuthAuthorizeTokenNested withNewOAuthAuthorizeToken(){
return new OAuthAuthorizeTokenNested();
}
public OAuthAuthorizeTokenList getOAuthAuthorizeTokenList(){
return this.OAuthAuthorizeTokenList!=null?this.OAuthAuthorizeTokenList.build():null;
}
public T withOAuthAuthorizeTokenList( OAuthAuthorizeTokenList OAuthAuthorizeTokenList){
if (OAuthAuthorizeTokenList!=null){ this.OAuthAuthorizeTokenList= new OAuthAuthorizeTokenListBuilder(OAuthAuthorizeTokenList); _visitables.add(this.OAuthAuthorizeTokenList);} return (T) this;
}
public OAuthAuthorizeTokenListNested withNewOAuthAuthorizeTokenList(){
return new OAuthAuthorizeTokenListNested();
}
public OAuthClient getOAuthClient(){
return this.OAuthClient!=null?this.OAuthClient.build():null;
}
public T withOAuthClient( OAuthClient OAuthClient){
if (OAuthClient!=null){ this.OAuthClient= new OAuthClientBuilder(OAuthClient); _visitables.add(this.OAuthClient);} return (T) this;
}
public OAuthClientNested withNewOAuthClient(){
return new OAuthClientNested();
}
public OAuthClientAuthorization getOAuthClientAuthorization(){
return this.OAuthClientAuthorization!=null?this.OAuthClientAuthorization.build():null;
}
public T withOAuthClientAuthorization( OAuthClientAuthorization OAuthClientAuthorization){
if (OAuthClientAuthorization!=null){ this.OAuthClientAuthorization= new OAuthClientAuthorizationBuilder(OAuthClientAuthorization); _visitables.add(this.OAuthClientAuthorization);} return (T) this;
}
public OAuthClientAuthorizationNested withNewOAuthClientAuthorization(){
return new OAuthClientAuthorizationNested();
}
public OAuthClientAuthorizationList getOAuthClientAuthorizationList(){
return this.OAuthClientAuthorizationList!=null?this.OAuthClientAuthorizationList.build():null;
}
public T withOAuthClientAuthorizationList( OAuthClientAuthorizationList OAuthClientAuthorizationList){
if (OAuthClientAuthorizationList!=null){ this.OAuthClientAuthorizationList= new OAuthClientAuthorizationListBuilder(OAuthClientAuthorizationList); _visitables.add(this.OAuthClientAuthorizationList);} return (T) this;
}
public OAuthClientAuthorizationListNested withNewOAuthClientAuthorizationList(){
return new OAuthClientAuthorizationListNested();
}
public OAuthClientList getOAuthClientList(){
return this.OAuthClientList!=null?this.OAuthClientList.build():null;
}
public T withOAuthClientList( OAuthClientList OAuthClientList){
if (OAuthClientList!=null){ this.OAuthClientList= new OAuthClientListBuilder(OAuthClientList); _visitables.add(this.OAuthClientList);} return (T) this;
}
public OAuthClientListNested withNewOAuthClientList(){
return new OAuthClientListNested();
}
public ObjectMeta getObjectMeta(){
return this.ObjectMeta!=null?this.ObjectMeta.build():null;
}
public T withObjectMeta( ObjectMeta ObjectMeta){
if (ObjectMeta!=null){ this.ObjectMeta= new ObjectMetaBuilder(ObjectMeta); _visitables.add(this.ObjectMeta);} return (T) this;
}
public ObjectMetaNested withNewObjectMeta(){
return new ObjectMetaNested();
}
public PodList getPodList(){
return this.PodList!=null?this.PodList.build():null;
}
public T withPodList( PodList PodList){
if (PodList!=null){ this.PodList= new PodListBuilder(PodList); _visitables.add(this.PodList);} return (T) this;
}
public PodListNested withNewPodList(){
return new PodListNested();
}
public Quantity getQuantity(){
return this.Quantity!=null?this.Quantity.build():null;
}
public T withQuantity( Quantity Quantity){
if (Quantity!=null){ this.Quantity= new QuantityBuilder(Quantity); _visitables.add(this.Quantity);} return (T) this;
}
public QuantityNested withNewQuantity(){
return new QuantityNested();
}
public T withNewQuantity( String amount, String format){
return withQuantity(new Quantity(amount, format));
}
public T withNewQuantity( String amount){
return withQuantity(new Quantity(amount));
}
public ReplicationControllerList getReplicationControllerList(){
return this.ReplicationControllerList!=null?this.ReplicationControllerList.build():null;
}
public T withReplicationControllerList( ReplicationControllerList ReplicationControllerList){
if (ReplicationControllerList!=null){ this.ReplicationControllerList= new ReplicationControllerListBuilder(ReplicationControllerList); _visitables.add(this.ReplicationControllerList);} return (T) this;
}
public ReplicationControllerListNested withNewReplicationControllerList(){
return new ReplicationControllerListNested();
}
public RouteList getRouteList(){
return this.RouteList!=null?this.RouteList.build():null;
}
public T withRouteList( RouteList RouteList){
if (RouteList!=null){ this.RouteList= new RouteListBuilder(RouteList); _visitables.add(this.RouteList);} return (T) this;
}
public RouteListNested withNewRouteList(){
return new RouteListNested();
}
public Secret getSecret(){
return this.Secret!=null?this.Secret.build():null;
}
public T withSecret( Secret Secret){
if (Secret!=null){ this.Secret= new SecretBuilder(Secret); _visitables.add(this.Secret);} return (T) this;
}
public SecretNested withNewSecret(){
return new SecretNested();
}
public SecretList getSecretList(){
return this.SecretList!=null?this.SecretList.build():null;
}
public T withSecretList( SecretList SecretList){
if (SecretList!=null){ this.SecretList= new SecretListBuilder(SecretList); _visitables.add(this.SecretList);} return (T) this;
}
public SecretListNested withNewSecretList(){
return new SecretListNested();
}
public ServiceList getServiceList(){
return this.ServiceList!=null?this.ServiceList.build():null;
}
public T withServiceList( ServiceList ServiceList){
if (ServiceList!=null){ this.ServiceList= new ServiceListBuilder(ServiceList); _visitables.add(this.ServiceList);} return (T) this;
}
public ServiceListNested withNewServiceList(){
return new ServiceListNested();
}
public StatusError getStatusError(){
return this.StatusError;
}
public T withStatusError( StatusError StatusError){
this.StatusError=StatusError; return (T) this;
}
public TagEvent getTagEvent(){
return this.TagEvent!=null?this.TagEvent.build():null;
}
public T withTagEvent( TagEvent TagEvent){
if (TagEvent!=null){ this.TagEvent= new TagEventBuilder(TagEvent); _visitables.add(this.TagEvent);} return (T) this;
}
public TagEventNested withNewTagEvent(){
return new TagEventNested();
}
public T withNewTagEvent( String created, String dockerImageReference, String image){
return withTagEvent(new TagEvent(created, dockerImageReference, image));
}
public Template getTemplate(){
return this.Template!=null?this.Template.build():null;
}
public T withTemplate( Template Template){
if (Template!=null){ this.Template= new TemplateBuilder(Template); _visitables.add(this.Template);} return (T) this;
}
public TemplateNested withNewTemplate(){
return new TemplateNested();
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public class BaseKubernetesListNested extends BaseKubernetesListFluent> implements Nested{
private final BaseKubernetesListBuilder builder = new BaseKubernetesListBuilder(this);
public N endBaseKubernetesList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withBaseKubernetesList(builder.build());
}
}
public class BuildConfigListNested extends BuildConfigListFluent> implements Nested{
private final BuildConfigListBuilder builder = new BuildConfigListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withBuildConfigList(builder.build());
}
public N endBuildConfigList(){
return and();
}
}
public class BuildListNested extends BuildListFluent> implements Nested{
private final BuildListBuilder builder = new BuildListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withBuildList(builder.build());
}
public N endBuildList(){
return and();
}
}
public class ContainerStatusNested extends ContainerStatusFluent> implements Nested{
private final ContainerStatusBuilder builder = new ContainerStatusBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withContainerStatus(builder.build());
}
public N endContainerStatus(){
return and();
}
}
public class DeploymentConfigListNested extends DeploymentConfigListFluent> implements Nested{
private final DeploymentConfigListBuilder builder = new DeploymentConfigListBuilder(this);
public N endDeploymentConfigList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withDeploymentConfigList(builder.build());
}
}
public class EndpointsNested extends EndpointsFluent> implements Nested{
private final EndpointsBuilder builder = new EndpointsBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withEndpoints(builder.build());
}
public N endEndpoints(){
return and();
}
}
public class EndpointsListNested extends EndpointsListFluent> implements Nested{
private final EndpointsListBuilder builder = new EndpointsListBuilder(this);
public N endEndpointsList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withEndpointsList(builder.build());
}
}
public class EnvVarNested extends EnvVarFluent> implements Nested{
private final EnvVarBuilder builder = new EnvVarBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withEnvVar(builder.build());
}
public N endEnvVar(){
return and();
}
}
public class ImageListNested extends ImageListFluent> implements Nested{
private final ImageListBuilder builder = new ImageListBuilder(this);
public N endImageList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withImageList(builder.build());
}
}
public class ImageStreamListNested extends ImageStreamListFluent> implements Nested{
private final ImageStreamListBuilder builder = new ImageStreamListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withImageStreamList(builder.build());
}
public N endImageStreamList(){
return and();
}
}
public class NamespaceNested extends NamespaceFluent> implements Nested{
private final NamespaceBuilder builder = new NamespaceBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withNamespace(builder.build());
}
public N endNamespace(){
return and();
}
}
public class NamespaceListNested extends NamespaceListFluent> implements Nested{
private final NamespaceListBuilder builder = new NamespaceListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withNamespaceList(builder.build());
}
public N endNamespaceList(){
return and();
}
}
public class NodeNested extends NodeFluent> implements Nested{
private final NodeBuilder builder = new NodeBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withNode(builder.build());
}
public N endNode(){
return and();
}
}
public class NodeListNested extends NodeListFluent> implements Nested{
private final NodeListBuilder builder = new NodeListBuilder(this);
public N endNodeList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withNodeList(builder.build());
}
}
public class OAuthAccessTokenNested extends OAuthAccessTokenFluent> implements Nested{
private final OAuthAccessTokenBuilder builder = new OAuthAccessTokenBuilder(this);
public N endOAuthAccessToken(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withOAuthAccessToken(builder.build());
}
}
public class OAuthAccessTokenListNested extends OAuthAccessTokenListFluent> implements Nested{
private final OAuthAccessTokenListBuilder builder = new OAuthAccessTokenListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withOAuthAccessTokenList(builder.build());
}
public N endOAuthAccessTokenList(){
return and();
}
}
public class OAuthAuthorizeTokenNested extends OAuthAuthorizeTokenFluent> implements Nested{
private final OAuthAuthorizeTokenBuilder builder = new OAuthAuthorizeTokenBuilder(this);
public N endOAuthAuthorizeToken(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withOAuthAuthorizeToken(builder.build());
}
}
public class OAuthAuthorizeTokenListNested extends OAuthAuthorizeTokenListFluent> implements Nested{
private final OAuthAuthorizeTokenListBuilder builder = new OAuthAuthorizeTokenListBuilder(this);
public N endOAuthAuthorizeTokenList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withOAuthAuthorizeTokenList(builder.build());
}
}
public class OAuthClientNested extends OAuthClientFluent> implements Nested{
private final OAuthClientBuilder builder = new OAuthClientBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withOAuthClient(builder.build());
}
public N endOAuthClient(){
return and();
}
}
public class OAuthClientAuthorizationNested extends OAuthClientAuthorizationFluent> implements Nested{
private final OAuthClientAuthorizationBuilder builder = new OAuthClientAuthorizationBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withOAuthClientAuthorization(builder.build());
}
public N endOAuthClientAuthorization(){
return and();
}
}
public class OAuthClientAuthorizationListNested extends OAuthClientAuthorizationListFluent> implements Nested{
private final OAuthClientAuthorizationListBuilder builder = new OAuthClientAuthorizationListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withOAuthClientAuthorizationList(builder.build());
}
public N endOAuthClientAuthorizationList(){
return and();
}
}
public class OAuthClientListNested extends OAuthClientListFluent> implements Nested{
private final OAuthClientListBuilder builder = new OAuthClientListBuilder(this);
public N endOAuthClientList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withOAuthClientList(builder.build());
}
}
public class ObjectMetaNested extends ObjectMetaFluent> implements Nested{
private final ObjectMetaBuilder builder = new ObjectMetaBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withObjectMeta(builder.build());
}
public N endObjectMeta(){
return and();
}
}
public class PodListNested extends PodListFluent> implements Nested{
private final PodListBuilder builder = new PodListBuilder(this);
public N endPodList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withPodList(builder.build());
}
}
public class QuantityNested extends QuantityFluent> implements Nested{
private final QuantityBuilder builder = new QuantityBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withQuantity(builder.build());
}
public N endQuantity(){
return and();
}
}
public class ReplicationControllerListNested extends ReplicationControllerListFluent> implements Nested{
private final ReplicationControllerListBuilder builder = new ReplicationControllerListBuilder(this);
public N endReplicationControllerList(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withReplicationControllerList(builder.build());
}
}
public class RouteListNested extends RouteListFluent> implements Nested{
private final RouteListBuilder builder = new RouteListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withRouteList(builder.build());
}
public N endRouteList(){
return and();
}
}
public class SecretNested extends SecretFluent> implements Nested{
private final SecretBuilder builder = new SecretBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withSecret(builder.build());
}
public N endSecret(){
return and();
}
}
public class SecretListNested extends SecretListFluent> implements Nested{
private final SecretListBuilder builder = new SecretListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withSecretList(builder.build());
}
public N endSecretList(){
return and();
}
}
public class ServiceListNested extends ServiceListFluent> implements Nested{
private final ServiceListBuilder builder = new ServiceListBuilder(this);
public N and(){
return (N) KubeSchemaFluent.this.withServiceList(builder.build());
}
public N endServiceList(){
return and();
}
}
public class TagEventNested extends TagEventFluent> implements Nested{
private final TagEventBuilder builder = new TagEventBuilder(this);
public N endTagEvent(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withTagEvent(builder.build());
}
}
public class TemplateNested extends TemplateFluent> implements Nested{
private final TemplateBuilder builder = new TemplateBuilder(this);
public N endTemplate(){
return and();
}
public N and(){
return (N) KubeSchemaFluent.this.withTemplate(builder.build());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy