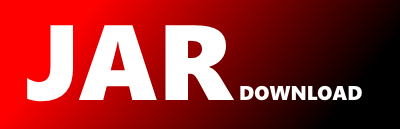
io.fabric8.kubernetes.api.model.ObjectMeta Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.Generated;
import javax.validation.Valid;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"annotations",
"creationTimestamp",
"deletionTimestamp",
"generateName",
"labels",
"name",
"namespace",
"resourceVersion",
"selfLink",
"uid"
})
public class ObjectMeta {
/**
* map of string keys and values that can be used by external tooling to store and retrieve arbitrary metadata about objects
*
*/
@JsonProperty("annotations")
@Valid
private Map annotations;
/**
* RFC 3339 date and time at which the object was created; populated by the system
*
*/
@JsonProperty("creationTimestamp")
private java.lang.String creationTimestamp;
/**
* RFC 3339 date and time at which the object will be deleted; populated by the system when a graceful deletion is requested
*
*/
@JsonProperty("deletionTimestamp")
private java.lang.String deletionTimestamp;
/**
* an optional prefix to use to generate a unique name; has the same validation rules as name; optional
*
*/
@JsonProperty("generateName")
private java.lang.String generateName;
/**
* map of string keys and values that can be used to organize and categorize objects; may match selectors of replication controllers and services
*
*/
@JsonProperty("labels")
@Valid
private Map labels;
/**
* string that identifies an object. Must be unique within a namespace; cannot be updated
*
*/
@JsonProperty("name")
@Pattern(regexp = "^[a-z0-9]([-a-z0-9]*[a-z0-9])?$")
@Size(max = 63)
private java.lang.String name;
/**
* namespace of the object; cannot be updated
*
*/
@JsonProperty("namespace")
@Pattern(regexp = "^[a-z0-9]([-a-z0-9]*[a-z0-9])?(\\.[a-z0-9]([-a-z0-9]*[a-z0-9])?)*$")
@Size(max = 253)
private java.lang.String namespace;
/**
* string that identifies the internal version of this object that can be used by clients to determine when objects have changed; populated by the system
*
*/
@JsonProperty("resourceVersion")
private java.lang.String resourceVersion;
/**
* URL for the object; populated by the system
*
*/
@JsonProperty("selfLink")
private java.lang.String selfLink;
/**
* unique UUID across space and time; populated by the system; read-only
*
*/
@JsonProperty("uid")
private java.lang.String uid;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
* No args constructor for use in serialization
*
*/
public ObjectMeta() {
}
/**
*
* @param uid
* @param deletionTimestamp
* @param resourceVersion
* @param name
* @param labels
* @param generateName
* @param selfLink
* @param creationTimestamp
* @param annotations
* @param namespace
*/
public ObjectMeta(Map annotations, java.lang.String creationTimestamp, java.lang.String deletionTimestamp, java.lang.String generateName, Map labels, java.lang.String name, java.lang.String namespace, java.lang.String resourceVersion, java.lang.String selfLink, java.lang.String uid) {
this.annotations = annotations;
this.creationTimestamp = creationTimestamp;
this.deletionTimestamp = deletionTimestamp;
this.generateName = generateName;
this.labels = labels;
this.name = name;
this.namespace = namespace;
this.resourceVersion = resourceVersion;
this.selfLink = selfLink;
this.uid = uid;
}
/**
* map of string keys and values that can be used by external tooling to store and retrieve arbitrary metadata about objects
*
* @return
* The annotations
*/
@JsonProperty("annotations")
public Map getAnnotations() {
return annotations;
}
/**
* map of string keys and values that can be used by external tooling to store and retrieve arbitrary metadata about objects
*
* @param annotations
* The annotations
*/
@JsonProperty("annotations")
public void setAnnotations(Map annotations) {
this.annotations = annotations;
}
/**
* RFC 3339 date and time at which the object was created; populated by the system
*
* @return
* The creationTimestamp
*/
@JsonProperty("creationTimestamp")
public java.lang.String getCreationTimestamp() {
return creationTimestamp;
}
/**
* RFC 3339 date and time at which the object was created; populated by the system
*
* @param creationTimestamp
* The creationTimestamp
*/
@JsonProperty("creationTimestamp")
public void setCreationTimestamp(java.lang.String creationTimestamp) {
this.creationTimestamp = creationTimestamp;
}
/**
* RFC 3339 date and time at which the object will be deleted; populated by the system when a graceful deletion is requested
*
* @return
* The deletionTimestamp
*/
@JsonProperty("deletionTimestamp")
public java.lang.String getDeletionTimestamp() {
return deletionTimestamp;
}
/**
* RFC 3339 date and time at which the object will be deleted; populated by the system when a graceful deletion is requested
*
* @param deletionTimestamp
* The deletionTimestamp
*/
@JsonProperty("deletionTimestamp")
public void setDeletionTimestamp(java.lang.String deletionTimestamp) {
this.deletionTimestamp = deletionTimestamp;
}
/**
* an optional prefix to use to generate a unique name; has the same validation rules as name; optional
*
* @return
* The generateName
*/
@JsonProperty("generateName")
public java.lang.String getGenerateName() {
return generateName;
}
/**
* an optional prefix to use to generate a unique name; has the same validation rules as name; optional
*
* @param generateName
* The generateName
*/
@JsonProperty("generateName")
public void setGenerateName(java.lang.String generateName) {
this.generateName = generateName;
}
/**
* map of string keys and values that can be used to organize and categorize objects; may match selectors of replication controllers and services
*
* @return
* The labels
*/
@JsonProperty("labels")
public Map getLabels() {
return labels;
}
/**
* map of string keys and values that can be used to organize and categorize objects; may match selectors of replication controllers and services
*
* @param labels
* The labels
*/
@JsonProperty("labels")
public void setLabels(Map labels) {
this.labels = labels;
}
/**
* string that identifies an object. Must be unique within a namespace; cannot be updated
*
* @return
* The name
*/
@JsonProperty("name")
public java.lang.String getName() {
return name;
}
/**
* string that identifies an object. Must be unique within a namespace; cannot be updated
*
* @param name
* The name
*/
@JsonProperty("name")
public void setName(java.lang.String name) {
this.name = name;
}
/**
* namespace of the object; cannot be updated
*
* @return
* The namespace
*/
@JsonProperty("namespace")
public java.lang.String getNamespace() {
return namespace;
}
/**
* namespace of the object; cannot be updated
*
* @param namespace
* The namespace
*/
@JsonProperty("namespace")
public void setNamespace(java.lang.String namespace) {
this.namespace = namespace;
}
/**
* string that identifies the internal version of this object that can be used by clients to determine when objects have changed; populated by the system
*
* @return
* The resourceVersion
*/
@JsonProperty("resourceVersion")
public java.lang.String getResourceVersion() {
return resourceVersion;
}
/**
* string that identifies the internal version of this object that can be used by clients to determine when objects have changed; populated by the system
*
* @param resourceVersion
* The resourceVersion
*/
@JsonProperty("resourceVersion")
public void setResourceVersion(java.lang.String resourceVersion) {
this.resourceVersion = resourceVersion;
}
/**
* URL for the object; populated by the system
*
* @return
* The selfLink
*/
@JsonProperty("selfLink")
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* URL for the object; populated by the system
*
* @param selfLink
* The selfLink
*/
@JsonProperty("selfLink")
public void setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
}
/**
* unique UUID across space and time; populated by the system; read-only
*
* @return
* The uid
*/
@JsonProperty("uid")
public java.lang.String getUid() {
return uid;
}
/**
* unique UUID across space and time; populated by the system; read-only
*
* @param uid
* The uid
*/
@JsonProperty("uid")
public void setUid(java.lang.String uid) {
this.uid = uid;
}
@Override
public java.lang.String toString() {
return ToStringBuilder.reflectionToString(this);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(java.lang.String name, Object value) {
this.additionalProperties.put(name, value);
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(annotations).append(creationTimestamp).append(deletionTimestamp).append(generateName).append(labels).append(name).append(namespace).append(resourceVersion).append(selfLink).append(uid).append(additionalProperties).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof ObjectMeta) == false) {
return false;
}
ObjectMeta rhs = ((ObjectMeta) other);
return new EqualsBuilder().append(annotations, rhs.annotations).append(creationTimestamp, rhs.creationTimestamp).append(deletionTimestamp, rhs.deletionTimestamp).append(generateName, rhs.generateName).append(labels, rhs.labels).append(name, rhs.name).append(namespace, rhs.namespace).append(resourceVersion, rhs.resourceVersion).append(selfLink, rhs.selfLink).append(uid, rhs.uid).append(additionalProperties, rhs.additionalProperties).isEquals();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy