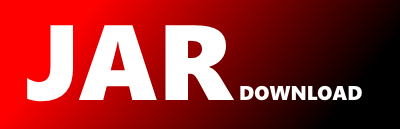
io.fabric8.kubernetes.api.model.ObjectMetaFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.HashMap;
import java.util.Map;
import io.fabric8.common.Fluent;
import io.fabric8.common.BaseFluent;
import io.fabric8.common.Visitable;
public class ObjectMetaFluent> extends BaseFluent implements Fluent{
Map annotations = new HashMap(); String creationTimestamp; String deletionTimestamp; String generateName; Map labels = new HashMap(); String name; String namespace; String resourceVersion; String selfLink; String uid; Map additionalProperties = new HashMap();
public T addToAnnotations( String key, String value){
if(key != null && value != null) {this.annotations.put(key, value);} return (T)this;
}
public Map getAnnotations(){
return this.annotations;
}
public T withAnnotations( Map annotations){
this.annotations.clear();if (annotations != null) {this.annotations.putAll(annotations);} return (T) this;
}
public String getCreationTimestamp(){
return this.creationTimestamp;
}
public T withCreationTimestamp( String creationTimestamp){
this.creationTimestamp=creationTimestamp; return (T) this;
}
public String getDeletionTimestamp(){
return this.deletionTimestamp;
}
public T withDeletionTimestamp( String deletionTimestamp){
this.deletionTimestamp=deletionTimestamp; return (T) this;
}
public String getGenerateName(){
return this.generateName;
}
public T withGenerateName( String generateName){
this.generateName=generateName; return (T) this;
}
public T addToLabels( String key, String value){
if(key != null && value != null) {this.labels.put(key, value);} return (T)this;
}
public Map getLabels(){
return this.labels;
}
public T withLabels( Map labels){
this.labels.clear();if (labels != null) {this.labels.putAll(labels);} return (T) this;
}
public String getName(){
return this.name;
}
public T withName( String name){
this.name=name; return (T) this;
}
public String getNamespace(){
return this.namespace;
}
public T withNamespace( String namespace){
this.namespace=namespace; return (T) this;
}
public String getResourceVersion(){
return this.resourceVersion;
}
public T withResourceVersion( String resourceVersion){
this.resourceVersion=resourceVersion; return (T) this;
}
public String getSelfLink(){
return this.selfLink;
}
public T withSelfLink( String selfLink){
this.selfLink=selfLink; return (T) this;
}
public String getUid(){
return this.uid;
}
public T withUid( String uid){
this.uid=uid; return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy