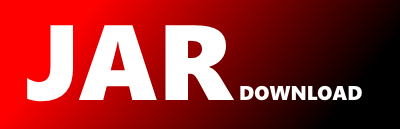
io.fabric8.kubernetes.api.model.PodSpec Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"containers",
"dnsPolicy",
"host",
"hostNetwork",
"nodeSelector",
"restartPolicy",
"terminationGracePeriodSeconds",
"volumes"
})
public class PodSpec {
/**
* list of containers belonging to the pod; cannot be updated; containers cannot currently be added or removed; there must be at least one container in a Pod
*
*/
@JsonProperty("containers")
@Valid
private List containers = new ArrayList();
/**
* DNS policy for containers within the pod; one of 'ClusterFirst' or 'Default'
*
*/
@JsonProperty("dnsPolicy")
private java.lang.String dnsPolicy;
/**
* host requested for this pod
*
*/
@JsonProperty("host")
private java.lang.String host;
/**
* host networking requested for this pod
*
*/
@JsonProperty("hostNetwork")
private Boolean hostNetwork;
/**
* selector which must match a node's labels for the pod to be scheduled on that node
*
*/
@JsonProperty("nodeSelector")
@Valid
private Map nodeSelector;
/**
* restart policy for all containers within the pod; one of RestartPolicyAlways
*
*/
@JsonProperty("restartPolicy")
private java.lang.String restartPolicy;
/**
* optional duration in seconds the pod needs to terminate gracefully; may be decreased in delete request; value must be non-negative integer; the value zero indicates delete immediately; if this value is not set
*
*/
@JsonProperty("terminationGracePeriodSeconds")
private Long terminationGracePeriodSeconds;
/**
* list of volumes that can be mounted by containers belonging to the pod
*
*/
@JsonProperty("volumes")
@Valid
private List volumes = new ArrayList();
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
* No args constructor for use in serialization
*
*/
public PodSpec() {
}
/**
*
* @param terminationGracePeriodSeconds
* @param containers
* @param host
* @param hostNetwork
* @param dnsPolicy
* @param volumes
* @param restartPolicy
* @param nodeSelector
*/
public PodSpec(List containers, java.lang.String dnsPolicy, java.lang.String host, Boolean hostNetwork, Map nodeSelector, java.lang.String restartPolicy, Long terminationGracePeriodSeconds, List volumes) {
this.containers = containers;
this.dnsPolicy = dnsPolicy;
this.host = host;
this.hostNetwork = hostNetwork;
this.nodeSelector = nodeSelector;
this.restartPolicy = restartPolicy;
this.terminationGracePeriodSeconds = terminationGracePeriodSeconds;
this.volumes = volumes;
}
/**
* list of containers belonging to the pod; cannot be updated; containers cannot currently be added or removed; there must be at least one container in a Pod
*
* @return
* The containers
*/
@JsonProperty("containers")
public List getContainers() {
return containers;
}
/**
* list of containers belonging to the pod; cannot be updated; containers cannot currently be added or removed; there must be at least one container in a Pod
*
* @param containers
* The containers
*/
@JsonProperty("containers")
public void setContainers(List containers) {
this.containers = containers;
}
/**
* DNS policy for containers within the pod; one of 'ClusterFirst' or 'Default'
*
* @return
* The dnsPolicy
*/
@JsonProperty("dnsPolicy")
public java.lang.String getDnsPolicy() {
return dnsPolicy;
}
/**
* DNS policy for containers within the pod; one of 'ClusterFirst' or 'Default'
*
* @param dnsPolicy
* The dnsPolicy
*/
@JsonProperty("dnsPolicy")
public void setDnsPolicy(java.lang.String dnsPolicy) {
this.dnsPolicy = dnsPolicy;
}
/**
* host requested for this pod
*
* @return
* The host
*/
@JsonProperty("host")
public java.lang.String getHost() {
return host;
}
/**
* host requested for this pod
*
* @param host
* The host
*/
@JsonProperty("host")
public void setHost(java.lang.String host) {
this.host = host;
}
/**
* host networking requested for this pod
*
* @return
* The hostNetwork
*/
@JsonProperty("hostNetwork")
public Boolean getHostNetwork() {
return hostNetwork;
}
/**
* host networking requested for this pod
*
* @param hostNetwork
* The hostNetwork
*/
@JsonProperty("hostNetwork")
public void setHostNetwork(Boolean hostNetwork) {
this.hostNetwork = hostNetwork;
}
/**
* selector which must match a node's labels for the pod to be scheduled on that node
*
* @return
* The nodeSelector
*/
@JsonProperty("nodeSelector")
public Map getNodeSelector() {
return nodeSelector;
}
/**
* selector which must match a node's labels for the pod to be scheduled on that node
*
* @param nodeSelector
* The nodeSelector
*/
@JsonProperty("nodeSelector")
public void setNodeSelector(Map nodeSelector) {
this.nodeSelector = nodeSelector;
}
/**
* restart policy for all containers within the pod; one of RestartPolicyAlways
*
* @return
* The restartPolicy
*/
@JsonProperty("restartPolicy")
public java.lang.String getRestartPolicy() {
return restartPolicy;
}
/**
* restart policy for all containers within the pod; one of RestartPolicyAlways
*
* @param restartPolicy
* The restartPolicy
*/
@JsonProperty("restartPolicy")
public void setRestartPolicy(java.lang.String restartPolicy) {
this.restartPolicy = restartPolicy;
}
/**
* optional duration in seconds the pod needs to terminate gracefully; may be decreased in delete request; value must be non-negative integer; the value zero indicates delete immediately; if this value is not set
*
* @return
* The terminationGracePeriodSeconds
*/
@JsonProperty("terminationGracePeriodSeconds")
public Long getTerminationGracePeriodSeconds() {
return terminationGracePeriodSeconds;
}
/**
* optional duration in seconds the pod needs to terminate gracefully; may be decreased in delete request; value must be non-negative integer; the value zero indicates delete immediately; if this value is not set
*
* @param terminationGracePeriodSeconds
* The terminationGracePeriodSeconds
*/
@JsonProperty("terminationGracePeriodSeconds")
public void setTerminationGracePeriodSeconds(Long terminationGracePeriodSeconds) {
this.terminationGracePeriodSeconds = terminationGracePeriodSeconds;
}
/**
* list of volumes that can be mounted by containers belonging to the pod
*
* @return
* The volumes
*/
@JsonProperty("volumes")
public List getVolumes() {
return volumes;
}
/**
* list of volumes that can be mounted by containers belonging to the pod
*
* @param volumes
* The volumes
*/
@JsonProperty("volumes")
public void setVolumes(List volumes) {
this.volumes = volumes;
}
@Override
public java.lang.String toString() {
return ToStringBuilder.reflectionToString(this);
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(java.lang.String name, Object value) {
this.additionalProperties.put(name, value);
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(containers).append(dnsPolicy).append(host).append(hostNetwork).append(nodeSelector).append(restartPolicy).append(terminationGracePeriodSeconds).append(volumes).append(additionalProperties).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof PodSpec) == false) {
return false;
}
PodSpec rhs = ((PodSpec) other);
return new EqualsBuilder().append(containers, rhs.containers).append(dnsPolicy, rhs.dnsPolicy).append(host, rhs.host).append(hostNetwork, rhs.hostNetwork).append(nodeSelector, rhs.nodeSelector).append(restartPolicy, rhs.restartPolicy).append(terminationGracePeriodSeconds, rhs.terminationGracePeriodSeconds).append(volumes, rhs.volumes).append(additionalProperties, rhs.additionalProperties).isEquals();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy