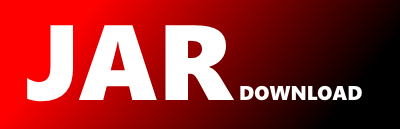
io.fabric8.kubernetes.api.model.VolumeFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.HashMap;
import java.util.Map;
import io.fabric8.common.Nested;
import io.fabric8.common.Builder;
import io.fabric8.common.Fluent;
import io.fabric8.common.Visitable;
import io.fabric8.common.BaseFluent;
public class VolumeFluent> extends BaseFluent implements Fluent{
AWSElasticBlockStoreVolumeSource awsElasticBlockStore; EmptyDirVolumeSourceBuilder emptyDir; GCEPersistentDiskVolumeSourceBuilder gcePersistentDisk; GitRepoVolumeSourceBuilder gitRepo; GlusterfsVolumeSourceBuilder glusterfs; HostPathVolumeSourceBuilder hostPath; ISCSIVolumeSourceBuilder iscsi; String name; NFSVolumeSourceBuilder nfs; PersistentVolumeClaimVolumeSource persistentVolumeClaim; SecretVolumeSourceBuilder secret; Map additionalProperties = new HashMap();
public AWSElasticBlockStoreVolumeSource getAwsElasticBlockStore(){
return this.awsElasticBlockStore;
}
public T withAwsElasticBlockStore( AWSElasticBlockStoreVolumeSource awsElasticBlockStore){
this.awsElasticBlockStore=awsElasticBlockStore; return (T) this;
}
public EmptyDirVolumeSource getEmptyDir(){
return this.emptyDir!=null?this.emptyDir.build():null;
}
public T withEmptyDir( EmptyDirVolumeSource emptyDir){
if (emptyDir!=null){ this.emptyDir= new EmptyDirVolumeSourceBuilder(emptyDir); _visitables.add(this.emptyDir);} return (T) this;
}
public EmptyDirNested withNewEmptyDir(){
return new EmptyDirNested();
}
public T withNewEmptyDir( String medium){
return withEmptyDir(new EmptyDirVolumeSource(medium));
}
public GCEPersistentDiskVolumeSource getGcePersistentDisk(){
return this.gcePersistentDisk!=null?this.gcePersistentDisk.build():null;
}
public T withGcePersistentDisk( GCEPersistentDiskVolumeSource gcePersistentDisk){
if (gcePersistentDisk!=null){ this.gcePersistentDisk= new GCEPersistentDiskVolumeSourceBuilder(gcePersistentDisk); _visitables.add(this.gcePersistentDisk);} return (T) this;
}
public GcePersistentDiskNested withNewGcePersistentDisk(){
return new GcePersistentDiskNested();
}
public T withNewGcePersistentDisk( String fsType, Integer partition, String pdName, Boolean readOnly){
return withGcePersistentDisk(new GCEPersistentDiskVolumeSource(fsType, partition, pdName, readOnly));
}
public GitRepoVolumeSource getGitRepo(){
return this.gitRepo!=null?this.gitRepo.build():null;
}
public T withGitRepo( GitRepoVolumeSource gitRepo){
if (gitRepo!=null){ this.gitRepo= new GitRepoVolumeSourceBuilder(gitRepo); _visitables.add(this.gitRepo);} return (T) this;
}
public GitRepoNested withNewGitRepo(){
return new GitRepoNested();
}
public T withNewGitRepo( String repository, String revision){
return withGitRepo(new GitRepoVolumeSource(repository, revision));
}
public GlusterfsVolumeSource getGlusterfs(){
return this.glusterfs!=null?this.glusterfs.build():null;
}
public T withGlusterfs( GlusterfsVolumeSource glusterfs){
if (glusterfs!=null){ this.glusterfs= new GlusterfsVolumeSourceBuilder(glusterfs); _visitables.add(this.glusterfs);} return (T) this;
}
public GlusterfsNested withNewGlusterfs(){
return new GlusterfsNested();
}
public T withNewGlusterfs( String endpoints, String path, Boolean readOnly){
return withGlusterfs(new GlusterfsVolumeSource(endpoints, path, readOnly));
}
public HostPathVolumeSource getHostPath(){
return this.hostPath!=null?this.hostPath.build():null;
}
public T withHostPath( HostPathVolumeSource hostPath){
if (hostPath!=null){ this.hostPath= new HostPathVolumeSourceBuilder(hostPath); _visitables.add(this.hostPath);} return (T) this;
}
public HostPathNested withNewHostPath(){
return new HostPathNested();
}
public T withNewHostPath( String path){
return withHostPath(new HostPathVolumeSource(path));
}
public ISCSIVolumeSource getIscsi(){
return this.iscsi!=null?this.iscsi.build():null;
}
public T withIscsi( ISCSIVolumeSource iscsi){
if (iscsi!=null){ this.iscsi= new ISCSIVolumeSourceBuilder(iscsi); _visitables.add(this.iscsi);} return (T) this;
}
public IscsiNested withNewIscsi(){
return new IscsiNested();
}
public T withNewIscsi( String fsType, String iqn, Integer lun, Boolean readOnly, String targetPortal){
return withIscsi(new ISCSIVolumeSource(fsType, iqn, lun, readOnly, targetPortal));
}
public String getName(){
return this.name;
}
public T withName( String name){
this.name=name; return (T) this;
}
public NFSVolumeSource getNfs(){
return this.nfs!=null?this.nfs.build():null;
}
public T withNfs( NFSVolumeSource nfs){
if (nfs!=null){ this.nfs= new NFSVolumeSourceBuilder(nfs); _visitables.add(this.nfs);} return (T) this;
}
public NfsNested withNewNfs(){
return new NfsNested();
}
public T withNewNfs( String path, Boolean readOnly, String server){
return withNfs(new NFSVolumeSource(path, readOnly, server));
}
public PersistentVolumeClaimVolumeSource getPersistentVolumeClaim(){
return this.persistentVolumeClaim;
}
public T withPersistentVolumeClaim( PersistentVolumeClaimVolumeSource persistentVolumeClaim){
this.persistentVolumeClaim=persistentVolumeClaim; return (T) this;
}
public SecretVolumeSource getSecret(){
return this.secret!=null?this.secret.build():null;
}
public T withSecret( SecretVolumeSource secret){
if (secret!=null){ this.secret= new SecretVolumeSourceBuilder(secret); _visitables.add(this.secret);} return (T) this;
}
public SecretNested withNewSecret(){
return new SecretNested();
}
public T withNewSecret( String secretName){
return withSecret(new SecretVolumeSource(secretName));
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public class EmptyDirNested extends EmptyDirVolumeSourceFluent> implements Nested{
private final EmptyDirVolumeSourceBuilder builder = new EmptyDirVolumeSourceBuilder(this);
public N and(){
return (N) VolumeFluent.this.withEmptyDir(builder.build());
}
public N endEmptyDir(){
return and();
}
}
public class GcePersistentDiskNested extends GCEPersistentDiskVolumeSourceFluent> implements Nested{
private final GCEPersistentDiskVolumeSourceBuilder builder = new GCEPersistentDiskVolumeSourceBuilder(this);
public N and(){
return (N) VolumeFluent.this.withGcePersistentDisk(builder.build());
}
public N endGcePersistentDisk(){
return and();
}
}
public class GitRepoNested extends GitRepoVolumeSourceFluent> implements Nested{
private final GitRepoVolumeSourceBuilder builder = new GitRepoVolumeSourceBuilder(this);
public N endGitRepo(){
return and();
}
public N and(){
return (N) VolumeFluent.this.withGitRepo(builder.build());
}
}
public class GlusterfsNested extends GlusterfsVolumeSourceFluent> implements Nested{
private final GlusterfsVolumeSourceBuilder builder = new GlusterfsVolumeSourceBuilder(this);
public N and(){
return (N) VolumeFluent.this.withGlusterfs(builder.build());
}
public N endGlusterfs(){
return and();
}
}
public class HostPathNested extends HostPathVolumeSourceFluent> implements Nested{
private final HostPathVolumeSourceBuilder builder = new HostPathVolumeSourceBuilder(this);
public N and(){
return (N) VolumeFluent.this.withHostPath(builder.build());
}
public N endHostPath(){
return and();
}
}
public class IscsiNested extends ISCSIVolumeSourceFluent> implements Nested{
private final ISCSIVolumeSourceBuilder builder = new ISCSIVolumeSourceBuilder(this);
public N and(){
return (N) VolumeFluent.this.withIscsi(builder.build());
}
public N endIscsi(){
return and();
}
}
public class NfsNested extends NFSVolumeSourceFluent> implements Nested{
private final NFSVolumeSourceBuilder builder = new NFSVolumeSourceBuilder(this);
public N and(){
return (N) VolumeFluent.this.withNfs(builder.build());
}
public N endNfs(){
return and();
}
}
public class SecretNested extends SecretVolumeSourceFluent> implements Nested{
private final SecretVolumeSourceBuilder builder = new SecretVolumeSourceBuilder(this);
public N endSecret(){
return and();
}
public N and(){
return (N) VolumeFluent.this.withSecret(builder.build());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy