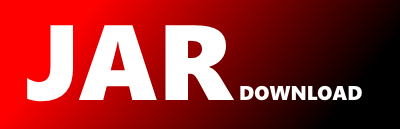
io.fabric8.certmanager.api.model.acme.v1.ACMEIssuerFluent Maven / Gradle / Ivy
package io.fabric8.certmanager.api.model.acme.v1;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import io.fabric8.certmanager.api.model.meta.v1.SecretKeySelector;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Iterator;
import java.util.List;
import java.lang.Boolean;
import io.fabric8.certmanager.api.model.meta.v1.SecretKeySelectorBuilder;
import io.fabric8.certmanager.api.model.meta.v1.SecretKeySelectorFluent;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class ACMEIssuerFluent> extends BaseFluent{
public ACMEIssuerFluent() {
}
public ACMEIssuerFluent(ACMEIssuer instance) {
this.copyInstance(instance);
}
private String caBundle;
private Boolean disableAccountKeyGeneration;
private String email;
private Boolean enableDurationFeature;
private ACMEExternalAccountBindingBuilder externalAccountBinding;
private String preferredChain;
private SecretKeySelectorBuilder privateKeySecretRef;
private String server;
private Boolean skipTLSVerify;
private ArrayList solvers = new ArrayList();
private Map additionalProperties;
protected void copyInstance(ACMEIssuer instance) {
instance = (instance != null ? instance : new ACMEIssuer());
if (instance != null) {
this.withCaBundle(instance.getCaBundle());
this.withDisableAccountKeyGeneration(instance.getDisableAccountKeyGeneration());
this.withEmail(instance.getEmail());
this.withEnableDurationFeature(instance.getEnableDurationFeature());
this.withExternalAccountBinding(instance.getExternalAccountBinding());
this.withPreferredChain(instance.getPreferredChain());
this.withPrivateKeySecretRef(instance.getPrivateKeySecretRef());
this.withServer(instance.getServer());
this.withSkipTLSVerify(instance.getSkipTLSVerify());
this.withSolvers(instance.getSolvers());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public String getCaBundle() {
return this.caBundle;
}
public A withCaBundle(String caBundle) {
this.caBundle = caBundle;
return (A) this;
}
public boolean hasCaBundle() {
return this.caBundle != null;
}
public Boolean getDisableAccountKeyGeneration() {
return this.disableAccountKeyGeneration;
}
public A withDisableAccountKeyGeneration(Boolean disableAccountKeyGeneration) {
this.disableAccountKeyGeneration = disableAccountKeyGeneration;
return (A) this;
}
public boolean hasDisableAccountKeyGeneration() {
return this.disableAccountKeyGeneration != null;
}
public String getEmail() {
return this.email;
}
public A withEmail(String email) {
this.email = email;
return (A) this;
}
public boolean hasEmail() {
return this.email != null;
}
public Boolean getEnableDurationFeature() {
return this.enableDurationFeature;
}
public A withEnableDurationFeature(Boolean enableDurationFeature) {
this.enableDurationFeature = enableDurationFeature;
return (A) this;
}
public boolean hasEnableDurationFeature() {
return this.enableDurationFeature != null;
}
public ACMEExternalAccountBinding buildExternalAccountBinding() {
return this.externalAccountBinding != null ? this.externalAccountBinding.build() : null;
}
public A withExternalAccountBinding(ACMEExternalAccountBinding externalAccountBinding) {
this._visitables.remove("externalAccountBinding");
if (externalAccountBinding != null) {
this.externalAccountBinding = new ACMEExternalAccountBindingBuilder(externalAccountBinding);
this._visitables.get("externalAccountBinding").add(this.externalAccountBinding);
} else {
this.externalAccountBinding = null;
this._visitables.get("externalAccountBinding").remove(this.externalAccountBinding);
}
return (A) this;
}
public boolean hasExternalAccountBinding() {
return this.externalAccountBinding != null;
}
public ExternalAccountBindingNested withNewExternalAccountBinding() {
return new ExternalAccountBindingNested(null);
}
public ExternalAccountBindingNested withNewExternalAccountBindingLike(ACMEExternalAccountBinding item) {
return new ExternalAccountBindingNested(item);
}
public ExternalAccountBindingNested editExternalAccountBinding() {
return withNewExternalAccountBindingLike(java.util.Optional.ofNullable(buildExternalAccountBinding()).orElse(null));
}
public ExternalAccountBindingNested editOrNewExternalAccountBinding() {
return withNewExternalAccountBindingLike(java.util.Optional.ofNullable(buildExternalAccountBinding()).orElse(new ACMEExternalAccountBindingBuilder().build()));
}
public ExternalAccountBindingNested editOrNewExternalAccountBindingLike(ACMEExternalAccountBinding item) {
return withNewExternalAccountBindingLike(java.util.Optional.ofNullable(buildExternalAccountBinding()).orElse(item));
}
public String getPreferredChain() {
return this.preferredChain;
}
public A withPreferredChain(String preferredChain) {
this.preferredChain = preferredChain;
return (A) this;
}
public boolean hasPreferredChain() {
return this.preferredChain != null;
}
public SecretKeySelector buildPrivateKeySecretRef() {
return this.privateKeySecretRef != null ? this.privateKeySecretRef.build() : null;
}
public A withPrivateKeySecretRef(SecretKeySelector privateKeySecretRef) {
this._visitables.remove("privateKeySecretRef");
if (privateKeySecretRef != null) {
this.privateKeySecretRef = new SecretKeySelectorBuilder(privateKeySecretRef);
this._visitables.get("privateKeySecretRef").add(this.privateKeySecretRef);
} else {
this.privateKeySecretRef = null;
this._visitables.get("privateKeySecretRef").remove(this.privateKeySecretRef);
}
return (A) this;
}
public boolean hasPrivateKeySecretRef() {
return this.privateKeySecretRef != null;
}
public A withNewPrivateKeySecretRef(String key,String name) {
return (A)withPrivateKeySecretRef(new SecretKeySelector(key, name));
}
public PrivateKeySecretRefNested withNewPrivateKeySecretRef() {
return new PrivateKeySecretRefNested(null);
}
public PrivateKeySecretRefNested withNewPrivateKeySecretRefLike(SecretKeySelector item) {
return new PrivateKeySecretRefNested(item);
}
public PrivateKeySecretRefNested editPrivateKeySecretRef() {
return withNewPrivateKeySecretRefLike(java.util.Optional.ofNullable(buildPrivateKeySecretRef()).orElse(null));
}
public PrivateKeySecretRefNested editOrNewPrivateKeySecretRef() {
return withNewPrivateKeySecretRefLike(java.util.Optional.ofNullable(buildPrivateKeySecretRef()).orElse(new SecretKeySelectorBuilder().build()));
}
public PrivateKeySecretRefNested editOrNewPrivateKeySecretRefLike(SecretKeySelector item) {
return withNewPrivateKeySecretRefLike(java.util.Optional.ofNullable(buildPrivateKeySecretRef()).orElse(item));
}
public String getServer() {
return this.server;
}
public A withServer(String server) {
this.server = server;
return (A) this;
}
public boolean hasServer() {
return this.server != null;
}
public Boolean getSkipTLSVerify() {
return this.skipTLSVerify;
}
public A withSkipTLSVerify(Boolean skipTLSVerify) {
this.skipTLSVerify = skipTLSVerify;
return (A) this;
}
public boolean hasSkipTLSVerify() {
return this.skipTLSVerify != null;
}
public A addToSolvers(int index,ACMEChallengeSolver item) {
if (this.solvers == null) {this.solvers = new ArrayList();}
ACMEChallengeSolverBuilder builder = new ACMEChallengeSolverBuilder(item);
if (index < 0 || index >= solvers.size()) { _visitables.get("solvers").add(builder); solvers.add(builder); } else { _visitables.get("solvers").add(index, builder); solvers.add(index, builder);}
return (A)this;
}
public A setToSolvers(int index,ACMEChallengeSolver item) {
if (this.solvers == null) {this.solvers = new ArrayList();}
ACMEChallengeSolverBuilder builder = new ACMEChallengeSolverBuilder(item);
if (index < 0 || index >= solvers.size()) { _visitables.get("solvers").add(builder); solvers.add(builder); } else { _visitables.get("solvers").set(index, builder); solvers.set(index, builder);}
return (A)this;
}
public A addToSolvers(io.fabric8.certmanager.api.model.acme.v1.ACMEChallengeSolver... items) {
if (this.solvers == null) {this.solvers = new ArrayList();}
for (ACMEChallengeSolver item : items) {ACMEChallengeSolverBuilder builder = new ACMEChallengeSolverBuilder(item);_visitables.get("solvers").add(builder);this.solvers.add(builder);} return (A)this;
}
public A addAllToSolvers(Collection items) {
if (this.solvers == null) {this.solvers = new ArrayList();}
for (ACMEChallengeSolver item : items) {ACMEChallengeSolverBuilder builder = new ACMEChallengeSolverBuilder(item);_visitables.get("solvers").add(builder);this.solvers.add(builder);} return (A)this;
}
public A removeFromSolvers(io.fabric8.certmanager.api.model.acme.v1.ACMEChallengeSolver... items) {
if (this.solvers == null) return (A)this;
for (ACMEChallengeSolver item : items) {ACMEChallengeSolverBuilder builder = new ACMEChallengeSolverBuilder(item);_visitables.get("solvers").remove(builder); this.solvers.remove(builder);} return (A)this;
}
public A removeAllFromSolvers(Collection items) {
if (this.solvers == null) return (A)this;
for (ACMEChallengeSolver item : items) {ACMEChallengeSolverBuilder builder = new ACMEChallengeSolverBuilder(item);_visitables.get("solvers").remove(builder); this.solvers.remove(builder);} return (A)this;
}
public A removeMatchingFromSolvers(Predicate predicate) {
if (solvers == null) return (A) this;
final Iterator each = solvers.iterator();
final List visitables = _visitables.get("solvers");
while (each.hasNext()) {
ACMEChallengeSolverBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildSolvers() {
return this.solvers != null ? build(solvers) : null;
}
public ACMEChallengeSolver buildSolver(int index) {
return this.solvers.get(index).build();
}
public ACMEChallengeSolver buildFirstSolver() {
return this.solvers.get(0).build();
}
public ACMEChallengeSolver buildLastSolver() {
return this.solvers.get(solvers.size() - 1).build();
}
public ACMEChallengeSolver buildMatchingSolver(Predicate predicate) {
for (ACMEChallengeSolverBuilder item : solvers) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingSolver(Predicate predicate) {
for (ACMEChallengeSolverBuilder item : solvers) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withSolvers(List solvers) {
if (this.solvers != null) {
this._visitables.get("solvers").clear();
}
if (solvers != null) {
this.solvers = new ArrayList();
for (ACMEChallengeSolver item : solvers) {
this.addToSolvers(item);
}
} else {
this.solvers = null;
}
return (A) this;
}
public A withSolvers(io.fabric8.certmanager.api.model.acme.v1.ACMEChallengeSolver... solvers) {
if (this.solvers != null) {
this.solvers.clear();
_visitables.remove("solvers");
}
if (solvers != null) {
for (ACMEChallengeSolver item : solvers) {
this.addToSolvers(item);
}
}
return (A) this;
}
public boolean hasSolvers() {
return this.solvers != null && !this.solvers.isEmpty();
}
public SolversNested addNewSolver() {
return new SolversNested(-1, null);
}
public SolversNested addNewSolverLike(ACMEChallengeSolver item) {
return new SolversNested(-1, item);
}
public SolversNested setNewSolverLike(int index,ACMEChallengeSolver item) {
return new SolversNested(index, item);
}
public SolversNested editSolver(int index) {
if (solvers.size() <= index) throw new RuntimeException("Can't edit solvers. Index exceeds size.");
return setNewSolverLike(index, buildSolver(index));
}
public SolversNested editFirstSolver() {
if (solvers.size() == 0) throw new RuntimeException("Can't edit first solvers. The list is empty.");
return setNewSolverLike(0, buildSolver(0));
}
public SolversNested editLastSolver() {
int index = solvers.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last solvers. The list is empty.");
return setNewSolverLike(index, buildSolver(index));
}
public SolversNested editMatchingSolver(Predicate predicate) {
int index = -1;
for (int i=0;i map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) {
this.additionalProperties = null;
} else {
this.additionalProperties = new LinkedHashMap(additionalProperties);
}
return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ACMEIssuerFluent that = (ACMEIssuerFluent) o;
if (!java.util.Objects.equals(caBundle, that.caBundle)) return false;
if (!java.util.Objects.equals(disableAccountKeyGeneration, that.disableAccountKeyGeneration)) return false;
if (!java.util.Objects.equals(email, that.email)) return false;
if (!java.util.Objects.equals(enableDurationFeature, that.enableDurationFeature)) return false;
if (!java.util.Objects.equals(externalAccountBinding, that.externalAccountBinding)) return false;
if (!java.util.Objects.equals(preferredChain, that.preferredChain)) return false;
if (!java.util.Objects.equals(privateKeySecretRef, that.privateKeySecretRef)) return false;
if (!java.util.Objects.equals(server, that.server)) return false;
if (!java.util.Objects.equals(skipTLSVerify, that.skipTLSVerify)) return false;
if (!java.util.Objects.equals(solvers, that.solvers)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(caBundle, disableAccountKeyGeneration, email, enableDurationFeature, externalAccountBinding, preferredChain, privateKeySecretRef, server, skipTLSVerify, solvers, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (caBundle != null) { sb.append("caBundle:"); sb.append(caBundle + ","); }
if (disableAccountKeyGeneration != null) { sb.append("disableAccountKeyGeneration:"); sb.append(disableAccountKeyGeneration + ","); }
if (email != null) { sb.append("email:"); sb.append(email + ","); }
if (enableDurationFeature != null) { sb.append("enableDurationFeature:"); sb.append(enableDurationFeature + ","); }
if (externalAccountBinding != null) { sb.append("externalAccountBinding:"); sb.append(externalAccountBinding + ","); }
if (preferredChain != null) { sb.append("preferredChain:"); sb.append(preferredChain + ","); }
if (privateKeySecretRef != null) { sb.append("privateKeySecretRef:"); sb.append(privateKeySecretRef + ","); }
if (server != null) { sb.append("server:"); sb.append(server + ","); }
if (skipTLSVerify != null) { sb.append("skipTLSVerify:"); sb.append(skipTLSVerify + ","); }
if (solvers != null && !solvers.isEmpty()) { sb.append("solvers:"); sb.append(solvers + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
public A withDisableAccountKeyGeneration() {
return withDisableAccountKeyGeneration(true);
}
public A withEnableDurationFeature() {
return withEnableDurationFeature(true);
}
public A withSkipTLSVerify() {
return withSkipTLSVerify(true);
}
public class ExternalAccountBindingNested extends ACMEExternalAccountBindingFluent> implements Nested{
ExternalAccountBindingNested(ACMEExternalAccountBinding item) {
this.builder = new ACMEExternalAccountBindingBuilder(this, item);
}
ACMEExternalAccountBindingBuilder builder;
public N and() {
return (N) ACMEIssuerFluent.this.withExternalAccountBinding(builder.build());
}
public N endExternalAccountBinding() {
return and();
}
}
public class PrivateKeySecretRefNested extends SecretKeySelectorFluent> implements Nested{
PrivateKeySecretRefNested(SecretKeySelector item) {
this.builder = new SecretKeySelectorBuilder(this, item);
}
SecretKeySelectorBuilder builder;
public N and() {
return (N) ACMEIssuerFluent.this.withPrivateKeySecretRef(builder.build());
}
public N endPrivateKeySecretRef() {
return and();
}
}
public class SolversNested extends ACMEChallengeSolverFluent> implements Nested{
SolversNested(int index,ACMEChallengeSolver item) {
this.index = index;
this.builder = new ACMEChallengeSolverBuilder(this, item);
}
ACMEChallengeSolverBuilder builder;
int index;
public N and() {
return (N) ACMEIssuerFluent.this.setToSolvers(index,builder.build());
}
public N endSolver() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy