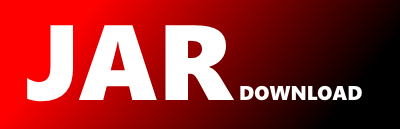
io.fabric8.certmanager.api.model.v1.CertificateSpecFluent Maven / Gradle / Ivy
package io.fabric8.certmanager.api.model.v1;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.util.ArrayList;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.List;
import java.lang.Boolean;
import io.fabric8.kubernetes.api.model.Duration;
import io.fabric8.certmanager.api.model.meta.v1.ObjectReferenceFluent;
import io.fabric8.certmanager.api.model.meta.v1.ObjectReferenceBuilder;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.certmanager.api.model.meta.v1.ObjectReference;
import java.util.Iterator;
import java.lang.Integer;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class CertificateSpecFluent> extends BaseFluent{
public CertificateSpecFluent() {
}
public CertificateSpecFluent(CertificateSpec instance) {
this.copyInstance(instance);
}
private ArrayList additionalOutputFormats = new ArrayList();
private String commonName;
private List dnsNames = new ArrayList();
private Duration duration;
private List emailAddresses = new ArrayList();
private Boolean encodeUsagesInRequest;
private List ipAddresses = new ArrayList();
private Boolean isCA;
private ObjectReferenceBuilder issuerRef;
private CertificateKeystoresBuilder keystores;
private String literalSubject;
private NameConstraintsBuilder nameConstraints;
private ArrayList otherNames = new ArrayList();
private CertificatePrivateKeyBuilder privateKey;
private Duration renewBefore;
private Integer revisionHistoryLimit;
private String secretName;
private CertificateSecretTemplateBuilder secretTemplate;
private X509SubjectBuilder subject;
private List uris = new ArrayList();
private List usages = new ArrayList();
private Map additionalProperties;
protected void copyInstance(CertificateSpec instance) {
instance = (instance != null ? instance : new CertificateSpec());
if (instance != null) {
this.withAdditionalOutputFormats(instance.getAdditionalOutputFormats());
this.withCommonName(instance.getCommonName());
this.withDnsNames(instance.getDnsNames());
this.withDuration(instance.getDuration());
this.withEmailAddresses(instance.getEmailAddresses());
this.withEncodeUsagesInRequest(instance.getEncodeUsagesInRequest());
this.withIpAddresses(instance.getIpAddresses());
this.withIsCA(instance.getIsCA());
this.withIssuerRef(instance.getIssuerRef());
this.withKeystores(instance.getKeystores());
this.withLiteralSubject(instance.getLiteralSubject());
this.withNameConstraints(instance.getNameConstraints());
this.withOtherNames(instance.getOtherNames());
this.withPrivateKey(instance.getPrivateKey());
this.withRenewBefore(instance.getRenewBefore());
this.withRevisionHistoryLimit(instance.getRevisionHistoryLimit());
this.withSecretName(instance.getSecretName());
this.withSecretTemplate(instance.getSecretTemplate());
this.withSubject(instance.getSubject());
this.withUris(instance.getUris());
this.withUsages(instance.getUsages());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public A addToAdditionalOutputFormats(int index,CertificateAdditionalOutputFormat item) {
if (this.additionalOutputFormats == null) {this.additionalOutputFormats = new ArrayList();}
CertificateAdditionalOutputFormatBuilder builder = new CertificateAdditionalOutputFormatBuilder(item);
if (index < 0 || index >= additionalOutputFormats.size()) { _visitables.get("additionalOutputFormats").add(builder); additionalOutputFormats.add(builder); } else { _visitables.get("additionalOutputFormats").add(index, builder); additionalOutputFormats.add(index, builder);}
return (A)this;
}
public A setToAdditionalOutputFormats(int index,CertificateAdditionalOutputFormat item) {
if (this.additionalOutputFormats == null) {this.additionalOutputFormats = new ArrayList();}
CertificateAdditionalOutputFormatBuilder builder = new CertificateAdditionalOutputFormatBuilder(item);
if (index < 0 || index >= additionalOutputFormats.size()) { _visitables.get("additionalOutputFormats").add(builder); additionalOutputFormats.add(builder); } else { _visitables.get("additionalOutputFormats").set(index, builder); additionalOutputFormats.set(index, builder);}
return (A)this;
}
public A addToAdditionalOutputFormats(io.fabric8.certmanager.api.model.v1.CertificateAdditionalOutputFormat... items) {
if (this.additionalOutputFormats == null) {this.additionalOutputFormats = new ArrayList();}
for (CertificateAdditionalOutputFormat item : items) {CertificateAdditionalOutputFormatBuilder builder = new CertificateAdditionalOutputFormatBuilder(item);_visitables.get("additionalOutputFormats").add(builder);this.additionalOutputFormats.add(builder);} return (A)this;
}
public A addAllToAdditionalOutputFormats(Collection items) {
if (this.additionalOutputFormats == null) {this.additionalOutputFormats = new ArrayList();}
for (CertificateAdditionalOutputFormat item : items) {CertificateAdditionalOutputFormatBuilder builder = new CertificateAdditionalOutputFormatBuilder(item);_visitables.get("additionalOutputFormats").add(builder);this.additionalOutputFormats.add(builder);} return (A)this;
}
public A removeFromAdditionalOutputFormats(io.fabric8.certmanager.api.model.v1.CertificateAdditionalOutputFormat... items) {
if (this.additionalOutputFormats == null) return (A)this;
for (CertificateAdditionalOutputFormat item : items) {CertificateAdditionalOutputFormatBuilder builder = new CertificateAdditionalOutputFormatBuilder(item);_visitables.get("additionalOutputFormats").remove(builder); this.additionalOutputFormats.remove(builder);} return (A)this;
}
public A removeAllFromAdditionalOutputFormats(Collection items) {
if (this.additionalOutputFormats == null) return (A)this;
for (CertificateAdditionalOutputFormat item : items) {CertificateAdditionalOutputFormatBuilder builder = new CertificateAdditionalOutputFormatBuilder(item);_visitables.get("additionalOutputFormats").remove(builder); this.additionalOutputFormats.remove(builder);} return (A)this;
}
public A removeMatchingFromAdditionalOutputFormats(Predicate predicate) {
if (additionalOutputFormats == null) return (A) this;
final Iterator each = additionalOutputFormats.iterator();
final List visitables = _visitables.get("additionalOutputFormats");
while (each.hasNext()) {
CertificateAdditionalOutputFormatBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildAdditionalOutputFormats() {
return this.additionalOutputFormats != null ? build(additionalOutputFormats) : null;
}
public CertificateAdditionalOutputFormat buildAdditionalOutputFormat(int index) {
return this.additionalOutputFormats.get(index).build();
}
public CertificateAdditionalOutputFormat buildFirstAdditionalOutputFormat() {
return this.additionalOutputFormats.get(0).build();
}
public CertificateAdditionalOutputFormat buildLastAdditionalOutputFormat() {
return this.additionalOutputFormats.get(additionalOutputFormats.size() - 1).build();
}
public CertificateAdditionalOutputFormat buildMatchingAdditionalOutputFormat(Predicate predicate) {
for (CertificateAdditionalOutputFormatBuilder item : additionalOutputFormats) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingAdditionalOutputFormat(Predicate predicate) {
for (CertificateAdditionalOutputFormatBuilder item : additionalOutputFormats) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withAdditionalOutputFormats(List additionalOutputFormats) {
if (this.additionalOutputFormats != null) {
this._visitables.get("additionalOutputFormats").clear();
}
if (additionalOutputFormats != null) {
this.additionalOutputFormats = new ArrayList();
for (CertificateAdditionalOutputFormat item : additionalOutputFormats) {
this.addToAdditionalOutputFormats(item);
}
} else {
this.additionalOutputFormats = null;
}
return (A) this;
}
public A withAdditionalOutputFormats(io.fabric8.certmanager.api.model.v1.CertificateAdditionalOutputFormat... additionalOutputFormats) {
if (this.additionalOutputFormats != null) {
this.additionalOutputFormats.clear();
_visitables.remove("additionalOutputFormats");
}
if (additionalOutputFormats != null) {
for (CertificateAdditionalOutputFormat item : additionalOutputFormats) {
this.addToAdditionalOutputFormats(item);
}
}
return (A) this;
}
public boolean hasAdditionalOutputFormats() {
return this.additionalOutputFormats != null && !this.additionalOutputFormats.isEmpty();
}
public A addNewAdditionalOutputFormat(String type) {
return (A)addToAdditionalOutputFormats(new CertificateAdditionalOutputFormat(type));
}
public AdditionalOutputFormatsNested addNewAdditionalOutputFormat() {
return new AdditionalOutputFormatsNested(-1, null);
}
public AdditionalOutputFormatsNested addNewAdditionalOutputFormatLike(CertificateAdditionalOutputFormat item) {
return new AdditionalOutputFormatsNested(-1, item);
}
public AdditionalOutputFormatsNested setNewAdditionalOutputFormatLike(int index,CertificateAdditionalOutputFormat item) {
return new AdditionalOutputFormatsNested(index, item);
}
public AdditionalOutputFormatsNested editAdditionalOutputFormat(int index) {
if (additionalOutputFormats.size() <= index) throw new RuntimeException("Can't edit additionalOutputFormats. Index exceeds size.");
return setNewAdditionalOutputFormatLike(index, buildAdditionalOutputFormat(index));
}
public AdditionalOutputFormatsNested editFirstAdditionalOutputFormat() {
if (additionalOutputFormats.size() == 0) throw new RuntimeException("Can't edit first additionalOutputFormats. The list is empty.");
return setNewAdditionalOutputFormatLike(0, buildAdditionalOutputFormat(0));
}
public AdditionalOutputFormatsNested editLastAdditionalOutputFormat() {
int index = additionalOutputFormats.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last additionalOutputFormats. The list is empty.");
return setNewAdditionalOutputFormatLike(index, buildAdditionalOutputFormat(index));
}
public AdditionalOutputFormatsNested editMatchingAdditionalOutputFormat(Predicate predicate) {
int index = -1;
for (int i=0;i();}
this.dnsNames.add(index, item);
return (A)this;
}
public A setToDnsNames(int index,String item) {
if (this.dnsNames == null) {this.dnsNames = new ArrayList();}
this.dnsNames.set(index, item); return (A)this;
}
public A addToDnsNames(java.lang.String... items) {
if (this.dnsNames == null) {this.dnsNames = new ArrayList();}
for (String item : items) {this.dnsNames.add(item);} return (A)this;
}
public A addAllToDnsNames(Collection items) {
if (this.dnsNames == null) {this.dnsNames = new ArrayList();}
for (String item : items) {this.dnsNames.add(item);} return (A)this;
}
public A removeFromDnsNames(java.lang.String... items) {
if (this.dnsNames == null) return (A)this;
for (String item : items) { this.dnsNames.remove(item);} return (A)this;
}
public A removeAllFromDnsNames(Collection items) {
if (this.dnsNames == null) return (A)this;
for (String item : items) { this.dnsNames.remove(item);} return (A)this;
}
public List getDnsNames() {
return this.dnsNames;
}
public String getDnsName(int index) {
return this.dnsNames.get(index);
}
public String getFirstDnsName() {
return this.dnsNames.get(0);
}
public String getLastDnsName() {
return this.dnsNames.get(dnsNames.size() - 1);
}
public String getMatchingDnsName(Predicate predicate) {
for (String item : dnsNames) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingDnsName(Predicate predicate) {
for (String item : dnsNames) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withDnsNames(List dnsNames) {
if (dnsNames != null) {
this.dnsNames = new ArrayList();
for (String item : dnsNames) {
this.addToDnsNames(item);
}
} else {
this.dnsNames = null;
}
return (A) this;
}
public A withDnsNames(java.lang.String... dnsNames) {
if (this.dnsNames != null) {
this.dnsNames.clear();
_visitables.remove("dnsNames");
}
if (dnsNames != null) {
for (String item : dnsNames) {
this.addToDnsNames(item);
}
}
return (A) this;
}
public boolean hasDnsNames() {
return this.dnsNames != null && !this.dnsNames.isEmpty();
}
public Duration getDuration() {
return this.duration;
}
public A withDuration(Duration duration) {
this.duration = duration;
return (A) this;
}
public boolean hasDuration() {
return this.duration != null;
}
public A addToEmailAddresses(int index,String item) {
if (this.emailAddresses == null) {this.emailAddresses = new ArrayList();}
this.emailAddresses.add(index, item);
return (A)this;
}
public A setToEmailAddresses(int index,String item) {
if (this.emailAddresses == null) {this.emailAddresses = new ArrayList();}
this.emailAddresses.set(index, item); return (A)this;
}
public A addToEmailAddresses(java.lang.String... items) {
if (this.emailAddresses == null) {this.emailAddresses = new ArrayList();}
for (String item : items) {this.emailAddresses.add(item);} return (A)this;
}
public A addAllToEmailAddresses(Collection items) {
if (this.emailAddresses == null) {this.emailAddresses = new ArrayList();}
for (String item : items) {this.emailAddresses.add(item);} return (A)this;
}
public A removeFromEmailAddresses(java.lang.String... items) {
if (this.emailAddresses == null) return (A)this;
for (String item : items) { this.emailAddresses.remove(item);} return (A)this;
}
public A removeAllFromEmailAddresses(Collection items) {
if (this.emailAddresses == null) return (A)this;
for (String item : items) { this.emailAddresses.remove(item);} return (A)this;
}
public List getEmailAddresses() {
return this.emailAddresses;
}
public String getEmailAddress(int index) {
return this.emailAddresses.get(index);
}
public String getFirstEmailAddress() {
return this.emailAddresses.get(0);
}
public String getLastEmailAddress() {
return this.emailAddresses.get(emailAddresses.size() - 1);
}
public String getMatchingEmailAddress(Predicate predicate) {
for (String item : emailAddresses) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingEmailAddress(Predicate predicate) {
for (String item : emailAddresses) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withEmailAddresses(List emailAddresses) {
if (emailAddresses != null) {
this.emailAddresses = new ArrayList();
for (String item : emailAddresses) {
this.addToEmailAddresses(item);
}
} else {
this.emailAddresses = null;
}
return (A) this;
}
public A withEmailAddresses(java.lang.String... emailAddresses) {
if (this.emailAddresses != null) {
this.emailAddresses.clear();
_visitables.remove("emailAddresses");
}
if (emailAddresses != null) {
for (String item : emailAddresses) {
this.addToEmailAddresses(item);
}
}
return (A) this;
}
public boolean hasEmailAddresses() {
return this.emailAddresses != null && !this.emailAddresses.isEmpty();
}
public Boolean getEncodeUsagesInRequest() {
return this.encodeUsagesInRequest;
}
public A withEncodeUsagesInRequest(Boolean encodeUsagesInRequest) {
this.encodeUsagesInRequest = encodeUsagesInRequest;
return (A) this;
}
public boolean hasEncodeUsagesInRequest() {
return this.encodeUsagesInRequest != null;
}
public A addToIpAddresses(int index,String item) {
if (this.ipAddresses == null) {this.ipAddresses = new ArrayList();}
this.ipAddresses.add(index, item);
return (A)this;
}
public A setToIpAddresses(int index,String item) {
if (this.ipAddresses == null) {this.ipAddresses = new ArrayList();}
this.ipAddresses.set(index, item); return (A)this;
}
public A addToIpAddresses(java.lang.String... items) {
if (this.ipAddresses == null) {this.ipAddresses = new ArrayList();}
for (String item : items) {this.ipAddresses.add(item);} return (A)this;
}
public A addAllToIpAddresses(Collection items) {
if (this.ipAddresses == null) {this.ipAddresses = new ArrayList();}
for (String item : items) {this.ipAddresses.add(item);} return (A)this;
}
public A removeFromIpAddresses(java.lang.String... items) {
if (this.ipAddresses == null) return (A)this;
for (String item : items) { this.ipAddresses.remove(item);} return (A)this;
}
public A removeAllFromIpAddresses(Collection items) {
if (this.ipAddresses == null) return (A)this;
for (String item : items) { this.ipAddresses.remove(item);} return (A)this;
}
public List getIpAddresses() {
return this.ipAddresses;
}
public String getIpAddress(int index) {
return this.ipAddresses.get(index);
}
public String getFirstIpAddress() {
return this.ipAddresses.get(0);
}
public String getLastIpAddress() {
return this.ipAddresses.get(ipAddresses.size() - 1);
}
public String getMatchingIpAddress(Predicate predicate) {
for (String item : ipAddresses) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingIpAddress(Predicate predicate) {
for (String item : ipAddresses) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withIpAddresses(List ipAddresses) {
if (ipAddresses != null) {
this.ipAddresses = new ArrayList();
for (String item : ipAddresses) {
this.addToIpAddresses(item);
}
} else {
this.ipAddresses = null;
}
return (A) this;
}
public A withIpAddresses(java.lang.String... ipAddresses) {
if (this.ipAddresses != null) {
this.ipAddresses.clear();
_visitables.remove("ipAddresses");
}
if (ipAddresses != null) {
for (String item : ipAddresses) {
this.addToIpAddresses(item);
}
}
return (A) this;
}
public boolean hasIpAddresses() {
return this.ipAddresses != null && !this.ipAddresses.isEmpty();
}
public Boolean getIsCA() {
return this.isCA;
}
public A withIsCA(Boolean isCA) {
this.isCA = isCA;
return (A) this;
}
public boolean hasIsCA() {
return this.isCA != null;
}
public ObjectReference buildIssuerRef() {
return this.issuerRef != null ? this.issuerRef.build() : null;
}
public A withIssuerRef(ObjectReference issuerRef) {
this._visitables.remove("issuerRef");
if (issuerRef != null) {
this.issuerRef = new ObjectReferenceBuilder(issuerRef);
this._visitables.get("issuerRef").add(this.issuerRef);
} else {
this.issuerRef = null;
this._visitables.get("issuerRef").remove(this.issuerRef);
}
return (A) this;
}
public boolean hasIssuerRef() {
return this.issuerRef != null;
}
public A withNewIssuerRef(String group,String kind,String name) {
return (A)withIssuerRef(new ObjectReference(group, kind, name));
}
public IssuerRefNested withNewIssuerRef() {
return new IssuerRefNested(null);
}
public IssuerRefNested withNewIssuerRefLike(ObjectReference item) {
return new IssuerRefNested(item);
}
public IssuerRefNested editIssuerRef() {
return withNewIssuerRefLike(java.util.Optional.ofNullable(buildIssuerRef()).orElse(null));
}
public IssuerRefNested editOrNewIssuerRef() {
return withNewIssuerRefLike(java.util.Optional.ofNullable(buildIssuerRef()).orElse(new ObjectReferenceBuilder().build()));
}
public IssuerRefNested editOrNewIssuerRefLike(ObjectReference item) {
return withNewIssuerRefLike(java.util.Optional.ofNullable(buildIssuerRef()).orElse(item));
}
public CertificateKeystores buildKeystores() {
return this.keystores != null ? this.keystores.build() : null;
}
public A withKeystores(CertificateKeystores keystores) {
this._visitables.remove("keystores");
if (keystores != null) {
this.keystores = new CertificateKeystoresBuilder(keystores);
this._visitables.get("keystores").add(this.keystores);
} else {
this.keystores = null;
this._visitables.get("keystores").remove(this.keystores);
}
return (A) this;
}
public boolean hasKeystores() {
return this.keystores != null;
}
public KeystoresNested withNewKeystores() {
return new KeystoresNested(null);
}
public KeystoresNested withNewKeystoresLike(CertificateKeystores item) {
return new KeystoresNested(item);
}
public KeystoresNested editKeystores() {
return withNewKeystoresLike(java.util.Optional.ofNullable(buildKeystores()).orElse(null));
}
public KeystoresNested editOrNewKeystores() {
return withNewKeystoresLike(java.util.Optional.ofNullable(buildKeystores()).orElse(new CertificateKeystoresBuilder().build()));
}
public KeystoresNested editOrNewKeystoresLike(CertificateKeystores item) {
return withNewKeystoresLike(java.util.Optional.ofNullable(buildKeystores()).orElse(item));
}
public String getLiteralSubject() {
return this.literalSubject;
}
public A withLiteralSubject(String literalSubject) {
this.literalSubject = literalSubject;
return (A) this;
}
public boolean hasLiteralSubject() {
return this.literalSubject != null;
}
public NameConstraints buildNameConstraints() {
return this.nameConstraints != null ? this.nameConstraints.build() : null;
}
public A withNameConstraints(NameConstraints nameConstraints) {
this._visitables.remove("nameConstraints");
if (nameConstraints != null) {
this.nameConstraints = new NameConstraintsBuilder(nameConstraints);
this._visitables.get("nameConstraints").add(this.nameConstraints);
} else {
this.nameConstraints = null;
this._visitables.get("nameConstraints").remove(this.nameConstraints);
}
return (A) this;
}
public boolean hasNameConstraints() {
return this.nameConstraints != null;
}
public NameConstraintsNested withNewNameConstraints() {
return new NameConstraintsNested(null);
}
public NameConstraintsNested withNewNameConstraintsLike(NameConstraints item) {
return new NameConstraintsNested(item);
}
public NameConstraintsNested editNameConstraints() {
return withNewNameConstraintsLike(java.util.Optional.ofNullable(buildNameConstraints()).orElse(null));
}
public NameConstraintsNested editOrNewNameConstraints() {
return withNewNameConstraintsLike(java.util.Optional.ofNullable(buildNameConstraints()).orElse(new NameConstraintsBuilder().build()));
}
public NameConstraintsNested editOrNewNameConstraintsLike(NameConstraints item) {
return withNewNameConstraintsLike(java.util.Optional.ofNullable(buildNameConstraints()).orElse(item));
}
public A addToOtherNames(int index,OtherName item) {
if (this.otherNames == null) {this.otherNames = new ArrayList();}
OtherNameBuilder builder = new OtherNameBuilder(item);
if (index < 0 || index >= otherNames.size()) { _visitables.get("otherNames").add(builder); otherNames.add(builder); } else { _visitables.get("otherNames").add(index, builder); otherNames.add(index, builder);}
return (A)this;
}
public A setToOtherNames(int index,OtherName item) {
if (this.otherNames == null) {this.otherNames = new ArrayList();}
OtherNameBuilder builder = new OtherNameBuilder(item);
if (index < 0 || index >= otherNames.size()) { _visitables.get("otherNames").add(builder); otherNames.add(builder); } else { _visitables.get("otherNames").set(index, builder); otherNames.set(index, builder);}
return (A)this;
}
public A addToOtherNames(io.fabric8.certmanager.api.model.v1.OtherName... items) {
if (this.otherNames == null) {this.otherNames = new ArrayList();}
for (OtherName item : items) {OtherNameBuilder builder = new OtherNameBuilder(item);_visitables.get("otherNames").add(builder);this.otherNames.add(builder);} return (A)this;
}
public A addAllToOtherNames(Collection items) {
if (this.otherNames == null) {this.otherNames = new ArrayList();}
for (OtherName item : items) {OtherNameBuilder builder = new OtherNameBuilder(item);_visitables.get("otherNames").add(builder);this.otherNames.add(builder);} return (A)this;
}
public A removeFromOtherNames(io.fabric8.certmanager.api.model.v1.OtherName... items) {
if (this.otherNames == null) return (A)this;
for (OtherName item : items) {OtherNameBuilder builder = new OtherNameBuilder(item);_visitables.get("otherNames").remove(builder); this.otherNames.remove(builder);} return (A)this;
}
public A removeAllFromOtherNames(Collection items) {
if (this.otherNames == null) return (A)this;
for (OtherName item : items) {OtherNameBuilder builder = new OtherNameBuilder(item);_visitables.get("otherNames").remove(builder); this.otherNames.remove(builder);} return (A)this;
}
public A removeMatchingFromOtherNames(Predicate predicate) {
if (otherNames == null) return (A) this;
final Iterator each = otherNames.iterator();
final List visitables = _visitables.get("otherNames");
while (each.hasNext()) {
OtherNameBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildOtherNames() {
return this.otherNames != null ? build(otherNames) : null;
}
public OtherName buildOtherName(int index) {
return this.otherNames.get(index).build();
}
public OtherName buildFirstOtherName() {
return this.otherNames.get(0).build();
}
public OtherName buildLastOtherName() {
return this.otherNames.get(otherNames.size() - 1).build();
}
public OtherName buildMatchingOtherName(Predicate predicate) {
for (OtherNameBuilder item : otherNames) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingOtherName(Predicate predicate) {
for (OtherNameBuilder item : otherNames) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withOtherNames(List otherNames) {
if (this.otherNames != null) {
this._visitables.get("otherNames").clear();
}
if (otherNames != null) {
this.otherNames = new ArrayList();
for (OtherName item : otherNames) {
this.addToOtherNames(item);
}
} else {
this.otherNames = null;
}
return (A) this;
}
public A withOtherNames(io.fabric8.certmanager.api.model.v1.OtherName... otherNames) {
if (this.otherNames != null) {
this.otherNames.clear();
_visitables.remove("otherNames");
}
if (otherNames != null) {
for (OtherName item : otherNames) {
this.addToOtherNames(item);
}
}
return (A) this;
}
public boolean hasOtherNames() {
return this.otherNames != null && !this.otherNames.isEmpty();
}
public A addNewOtherName(String oid,String utf8Value) {
return (A)addToOtherNames(new OtherName(oid, utf8Value));
}
public OtherNamesNested addNewOtherName() {
return new OtherNamesNested(-1, null);
}
public OtherNamesNested addNewOtherNameLike(OtherName item) {
return new OtherNamesNested(-1, item);
}
public OtherNamesNested setNewOtherNameLike(int index,OtherName item) {
return new OtherNamesNested(index, item);
}
public OtherNamesNested editOtherName(int index) {
if (otherNames.size() <= index) throw new RuntimeException("Can't edit otherNames. Index exceeds size.");
return setNewOtherNameLike(index, buildOtherName(index));
}
public OtherNamesNested editFirstOtherName() {
if (otherNames.size() == 0) throw new RuntimeException("Can't edit first otherNames. The list is empty.");
return setNewOtherNameLike(0, buildOtherName(0));
}
public OtherNamesNested editLastOtherName() {
int index = otherNames.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last otherNames. The list is empty.");
return setNewOtherNameLike(index, buildOtherName(index));
}
public OtherNamesNested editMatchingOtherName(Predicate predicate) {
int index = -1;
for (int i=0;i withNewPrivateKey() {
return new PrivateKeyNested(null);
}
public PrivateKeyNested withNewPrivateKeyLike(CertificatePrivateKey item) {
return new PrivateKeyNested(item);
}
public PrivateKeyNested editPrivateKey() {
return withNewPrivateKeyLike(java.util.Optional.ofNullable(buildPrivateKey()).orElse(null));
}
public PrivateKeyNested editOrNewPrivateKey() {
return withNewPrivateKeyLike(java.util.Optional.ofNullable(buildPrivateKey()).orElse(new CertificatePrivateKeyBuilder().build()));
}
public PrivateKeyNested editOrNewPrivateKeyLike(CertificatePrivateKey item) {
return withNewPrivateKeyLike(java.util.Optional.ofNullable(buildPrivateKey()).orElse(item));
}
public Duration getRenewBefore() {
return this.renewBefore;
}
public A withRenewBefore(Duration renewBefore) {
this.renewBefore = renewBefore;
return (A) this;
}
public boolean hasRenewBefore() {
return this.renewBefore != null;
}
public Integer getRevisionHistoryLimit() {
return this.revisionHistoryLimit;
}
public A withRevisionHistoryLimit(Integer revisionHistoryLimit) {
this.revisionHistoryLimit = revisionHistoryLimit;
return (A) this;
}
public boolean hasRevisionHistoryLimit() {
return this.revisionHistoryLimit != null;
}
public String getSecretName() {
return this.secretName;
}
public A withSecretName(String secretName) {
this.secretName = secretName;
return (A) this;
}
public boolean hasSecretName() {
return this.secretName != null;
}
public CertificateSecretTemplate buildSecretTemplate() {
return this.secretTemplate != null ? this.secretTemplate.build() : null;
}
public A withSecretTemplate(CertificateSecretTemplate secretTemplate) {
this._visitables.remove("secretTemplate");
if (secretTemplate != null) {
this.secretTemplate = new CertificateSecretTemplateBuilder(secretTemplate);
this._visitables.get("secretTemplate").add(this.secretTemplate);
} else {
this.secretTemplate = null;
this._visitables.get("secretTemplate").remove(this.secretTemplate);
}
return (A) this;
}
public boolean hasSecretTemplate() {
return this.secretTemplate != null;
}
public SecretTemplateNested withNewSecretTemplate() {
return new SecretTemplateNested(null);
}
public SecretTemplateNested withNewSecretTemplateLike(CertificateSecretTemplate item) {
return new SecretTemplateNested(item);
}
public SecretTemplateNested editSecretTemplate() {
return withNewSecretTemplateLike(java.util.Optional.ofNullable(buildSecretTemplate()).orElse(null));
}
public SecretTemplateNested editOrNewSecretTemplate() {
return withNewSecretTemplateLike(java.util.Optional.ofNullable(buildSecretTemplate()).orElse(new CertificateSecretTemplateBuilder().build()));
}
public SecretTemplateNested editOrNewSecretTemplateLike(CertificateSecretTemplate item) {
return withNewSecretTemplateLike(java.util.Optional.ofNullable(buildSecretTemplate()).orElse(item));
}
public X509Subject buildSubject() {
return this.subject != null ? this.subject.build() : null;
}
public A withSubject(X509Subject subject) {
this._visitables.remove("subject");
if (subject != null) {
this.subject = new X509SubjectBuilder(subject);
this._visitables.get("subject").add(this.subject);
} else {
this.subject = null;
this._visitables.get("subject").remove(this.subject);
}
return (A) this;
}
public boolean hasSubject() {
return this.subject != null;
}
public SubjectNested withNewSubject() {
return new SubjectNested(null);
}
public SubjectNested withNewSubjectLike(X509Subject item) {
return new SubjectNested(item);
}
public SubjectNested editSubject() {
return withNewSubjectLike(java.util.Optional.ofNullable(buildSubject()).orElse(null));
}
public SubjectNested editOrNewSubject() {
return withNewSubjectLike(java.util.Optional.ofNullable(buildSubject()).orElse(new X509SubjectBuilder().build()));
}
public SubjectNested editOrNewSubjectLike(X509Subject item) {
return withNewSubjectLike(java.util.Optional.ofNullable(buildSubject()).orElse(item));
}
public A addToUris(int index,String item) {
if (this.uris == null) {this.uris = new ArrayList();}
this.uris.add(index, item);
return (A)this;
}
public A setToUris(int index,String item) {
if (this.uris == null) {this.uris = new ArrayList();}
this.uris.set(index, item); return (A)this;
}
public A addToUris(java.lang.String... items) {
if (this.uris == null) {this.uris = new ArrayList();}
for (String item : items) {this.uris.add(item);} return (A)this;
}
public A addAllToUris(Collection items) {
if (this.uris == null) {this.uris = new ArrayList();}
for (String item : items) {this.uris.add(item);} return (A)this;
}
public A removeFromUris(java.lang.String... items) {
if (this.uris == null) return (A)this;
for (String item : items) { this.uris.remove(item);} return (A)this;
}
public A removeAllFromUris(Collection items) {
if (this.uris == null) return (A)this;
for (String item : items) { this.uris.remove(item);} return (A)this;
}
public List getUris() {
return this.uris;
}
public String getUri(int index) {
return this.uris.get(index);
}
public String getFirstUri() {
return this.uris.get(0);
}
public String getLastUri() {
return this.uris.get(uris.size() - 1);
}
public String getMatchingUri(Predicate predicate) {
for (String item : uris) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingUri(Predicate predicate) {
for (String item : uris) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withUris(List uris) {
if (uris != null) {
this.uris = new ArrayList();
for (String item : uris) {
this.addToUris(item);
}
} else {
this.uris = null;
}
return (A) this;
}
public A withUris(java.lang.String... uris) {
if (this.uris != null) {
this.uris.clear();
_visitables.remove("uris");
}
if (uris != null) {
for (String item : uris) {
this.addToUris(item);
}
}
return (A) this;
}
public boolean hasUris() {
return this.uris != null && !this.uris.isEmpty();
}
public A addToUsages(int index,String item) {
if (this.usages == null) {this.usages = new ArrayList();}
this.usages.add(index, item);
return (A)this;
}
public A setToUsages(int index,String item) {
if (this.usages == null) {this.usages = new ArrayList();}
this.usages.set(index, item); return (A)this;
}
public A addToUsages(java.lang.String... items) {
if (this.usages == null) {this.usages = new ArrayList();}
for (String item : items) {this.usages.add(item);} return (A)this;
}
public A addAllToUsages(Collection items) {
if (this.usages == null) {this.usages = new ArrayList();}
for (String item : items) {this.usages.add(item);} return (A)this;
}
public A removeFromUsages(java.lang.String... items) {
if (this.usages == null) return (A)this;
for (String item : items) { this.usages.remove(item);} return (A)this;
}
public A removeAllFromUsages(Collection items) {
if (this.usages == null) return (A)this;
for (String item : items) { this.usages.remove(item);} return (A)this;
}
public List getUsages() {
return this.usages;
}
public String getUsage(int index) {
return this.usages.get(index);
}
public String getFirstUsage() {
return this.usages.get(0);
}
public String getLastUsage() {
return this.usages.get(usages.size() - 1);
}
public String getMatchingUsage(Predicate predicate) {
for (String item : usages) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingUsage(Predicate predicate) {
for (String item : usages) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withUsages(List usages) {
if (usages != null) {
this.usages = new ArrayList();
for (String item : usages) {
this.addToUsages(item);
}
} else {
this.usages = null;
}
return (A) this;
}
public A withUsages(java.lang.String... usages) {
if (this.usages != null) {
this.usages.clear();
_visitables.remove("usages");
}
if (usages != null) {
for (String item : usages) {
this.addToUsages(item);
}
}
return (A) this;
}
public boolean hasUsages() {
return this.usages != null && !this.usages.isEmpty();
}
public A addToAdditionalProperties(String key,Object value) {
if(this.additionalProperties == null && key != null && value != null) { this.additionalProperties = new LinkedHashMap(); }
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (A)this;
}
public A addToAdditionalProperties(Map map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) {
this.additionalProperties = null;
} else {
this.additionalProperties = new LinkedHashMap(additionalProperties);
}
return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
CertificateSpecFluent that = (CertificateSpecFluent) o;
if (!java.util.Objects.equals(additionalOutputFormats, that.additionalOutputFormats)) return false;
if (!java.util.Objects.equals(commonName, that.commonName)) return false;
if (!java.util.Objects.equals(dnsNames, that.dnsNames)) return false;
if (!java.util.Objects.equals(duration, that.duration)) return false;
if (!java.util.Objects.equals(emailAddresses, that.emailAddresses)) return false;
if (!java.util.Objects.equals(encodeUsagesInRequest, that.encodeUsagesInRequest)) return false;
if (!java.util.Objects.equals(ipAddresses, that.ipAddresses)) return false;
if (!java.util.Objects.equals(isCA, that.isCA)) return false;
if (!java.util.Objects.equals(issuerRef, that.issuerRef)) return false;
if (!java.util.Objects.equals(keystores, that.keystores)) return false;
if (!java.util.Objects.equals(literalSubject, that.literalSubject)) return false;
if (!java.util.Objects.equals(nameConstraints, that.nameConstraints)) return false;
if (!java.util.Objects.equals(otherNames, that.otherNames)) return false;
if (!java.util.Objects.equals(privateKey, that.privateKey)) return false;
if (!java.util.Objects.equals(renewBefore, that.renewBefore)) return false;
if (!java.util.Objects.equals(revisionHistoryLimit, that.revisionHistoryLimit)) return false;
if (!java.util.Objects.equals(secretName, that.secretName)) return false;
if (!java.util.Objects.equals(secretTemplate, that.secretTemplate)) return false;
if (!java.util.Objects.equals(subject, that.subject)) return false;
if (!java.util.Objects.equals(uris, that.uris)) return false;
if (!java.util.Objects.equals(usages, that.usages)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(additionalOutputFormats, commonName, dnsNames, duration, emailAddresses, encodeUsagesInRequest, ipAddresses, isCA, issuerRef, keystores, literalSubject, nameConstraints, otherNames, privateKey, renewBefore, revisionHistoryLimit, secretName, secretTemplate, subject, uris, usages, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (additionalOutputFormats != null && !additionalOutputFormats.isEmpty()) { sb.append("additionalOutputFormats:"); sb.append(additionalOutputFormats + ","); }
if (commonName != null) { sb.append("commonName:"); sb.append(commonName + ","); }
if (dnsNames != null && !dnsNames.isEmpty()) { sb.append("dnsNames:"); sb.append(dnsNames + ","); }
if (duration != null) { sb.append("duration:"); sb.append(duration + ","); }
if (emailAddresses != null && !emailAddresses.isEmpty()) { sb.append("emailAddresses:"); sb.append(emailAddresses + ","); }
if (encodeUsagesInRequest != null) { sb.append("encodeUsagesInRequest:"); sb.append(encodeUsagesInRequest + ","); }
if (ipAddresses != null && !ipAddresses.isEmpty()) { sb.append("ipAddresses:"); sb.append(ipAddresses + ","); }
if (isCA != null) { sb.append("isCA:"); sb.append(isCA + ","); }
if (issuerRef != null) { sb.append("issuerRef:"); sb.append(issuerRef + ","); }
if (keystores != null) { sb.append("keystores:"); sb.append(keystores + ","); }
if (literalSubject != null) { sb.append("literalSubject:"); sb.append(literalSubject + ","); }
if (nameConstraints != null) { sb.append("nameConstraints:"); sb.append(nameConstraints + ","); }
if (otherNames != null && !otherNames.isEmpty()) { sb.append("otherNames:"); sb.append(otherNames + ","); }
if (privateKey != null) { sb.append("privateKey:"); sb.append(privateKey + ","); }
if (renewBefore != null) { sb.append("renewBefore:"); sb.append(renewBefore + ","); }
if (revisionHistoryLimit != null) { sb.append("revisionHistoryLimit:"); sb.append(revisionHistoryLimit + ","); }
if (secretName != null) { sb.append("secretName:"); sb.append(secretName + ","); }
if (secretTemplate != null) { sb.append("secretTemplate:"); sb.append(secretTemplate + ","); }
if (subject != null) { sb.append("subject:"); sb.append(subject + ","); }
if (uris != null && !uris.isEmpty()) { sb.append("uris:"); sb.append(uris + ","); }
if (usages != null && !usages.isEmpty()) { sb.append("usages:"); sb.append(usages + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
public A withEncodeUsagesInRequest() {
return withEncodeUsagesInRequest(true);
}
public A withIsCA() {
return withIsCA(true);
}
public class AdditionalOutputFormatsNested extends CertificateAdditionalOutputFormatFluent> implements Nested{
AdditionalOutputFormatsNested(int index,CertificateAdditionalOutputFormat item) {
this.index = index;
this.builder = new CertificateAdditionalOutputFormatBuilder(this, item);
}
CertificateAdditionalOutputFormatBuilder builder;
int index;
public N and() {
return (N) CertificateSpecFluent.this.setToAdditionalOutputFormats(index,builder.build());
}
public N endAdditionalOutputFormat() {
return and();
}
}
public class IssuerRefNested extends ObjectReferenceFluent> implements Nested{
IssuerRefNested(ObjectReference item) {
this.builder = new ObjectReferenceBuilder(this, item);
}
ObjectReferenceBuilder builder;
public N and() {
return (N) CertificateSpecFluent.this.withIssuerRef(builder.build());
}
public N endIssuerRef() {
return and();
}
}
public class KeystoresNested extends CertificateKeystoresFluent> implements Nested{
KeystoresNested(CertificateKeystores item) {
this.builder = new CertificateKeystoresBuilder(this, item);
}
CertificateKeystoresBuilder builder;
public N and() {
return (N) CertificateSpecFluent.this.withKeystores(builder.build());
}
public N endKeystores() {
return and();
}
}
public class NameConstraintsNested extends NameConstraintsFluent> implements Nested{
NameConstraintsNested(NameConstraints item) {
this.builder = new NameConstraintsBuilder(this, item);
}
NameConstraintsBuilder builder;
public N and() {
return (N) CertificateSpecFluent.this.withNameConstraints(builder.build());
}
public N endNameConstraints() {
return and();
}
}
public class OtherNamesNested extends OtherNameFluent> implements Nested{
OtherNamesNested(int index,OtherName item) {
this.index = index;
this.builder = new OtherNameBuilder(this, item);
}
OtherNameBuilder builder;
int index;
public N and() {
return (N) CertificateSpecFluent.this.setToOtherNames(index,builder.build());
}
public N endOtherName() {
return and();
}
}
public class PrivateKeyNested extends CertificatePrivateKeyFluent> implements Nested{
PrivateKeyNested(CertificatePrivateKey item) {
this.builder = new CertificatePrivateKeyBuilder(this, item);
}
CertificatePrivateKeyBuilder builder;
public N and() {
return (N) CertificateSpecFluent.this.withPrivateKey(builder.build());
}
public N endPrivateKey() {
return and();
}
}
public class SecretTemplateNested extends CertificateSecretTemplateFluent> implements Nested{
SecretTemplateNested(CertificateSecretTemplate item) {
this.builder = new CertificateSecretTemplateBuilder(this, item);
}
CertificateSecretTemplateBuilder builder;
public N and() {
return (N) CertificateSpecFluent.this.withSecretTemplate(builder.build());
}
public N endSecretTemplate() {
return and();
}
}
public class SubjectNested extends X509SubjectFluent> implements Nested{
SubjectNested(X509Subject item) {
this.builder = new X509SubjectBuilder(this, item);
}
X509SubjectBuilder builder;
public N and() {
return (N) CertificateSpecFluent.this.withSubject(builder.build());
}
public N endSubject() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy