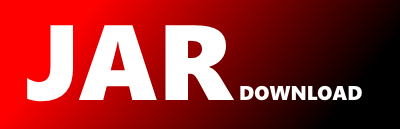
io.fabric8.utils.json.JsonReader Maven / Gradle / Ivy
/**
* Copyright 2005-2016 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version
* 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package io.fabric8.utils.json;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
public class JsonReader {
public static Object read(Reader reader) throws IOException {
return new JsonReader(reader).parse();
}
public static Object read(InputStream is) throws IOException {
return new JsonReader(new InputStreamReader(is)).parse();
}
//
// Implementation
//
private final Reader reader;
private final StringBuilder recorder;
private int current;
private int line = 1;
private int column = 0;
JsonReader(Reader reader) {
this.reader = reader;
recorder = new StringBuilder();
}
public Object parse() throws IOException {
read();
skipWhiteSpace();
Object result = readValue();
skipWhiteSpace();
if (!endOfText()) {
throw error("Unexpected character");
}
return result;
}
private Object readValue() throws IOException {
switch (current) {
case 'n':
return readNull();
case 't':
return readTrue();
case 'f':
return readFalse();
case '"':
return readString();
case '[':
return readArray();
case '{':
return readObject();
case '-':
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
return readNumber();
default:
throw expected("value");
}
}
private Collection> readArray() throws IOException {
read();
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy