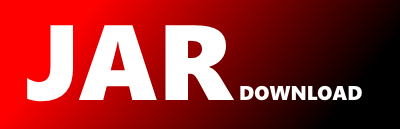
io.fabric8.kubernetes.api.model.apiextensions.AbstractJSONSchemaPropsAssert Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model.apiextensions;
import org.assertj.core.api.AbstractAssert;
import org.assertj.core.api.Assertions;
import org.assertj.core.util.Objects;
import org.assertj.core.api.AssertFactory;
import io.fabric8.kubernetes.assertions.NavigationListAssert;
import static org.assertj.core.api.Assertions.assertThat;
import static io.fabric8.kubernetes.assertions.internal.Assertions.assertThat;
/**
* Abstract base class for {@link JSONSchemaProps} specific assertions - Generated by CustomAssertionGenerator.
*/
public abstract class AbstractJSONSchemaPropsAssert, A extends JSONSchemaProps> extends AbstractAssert {
/**
* Creates a new {@link AbstractJSONSchemaPropsAssert}
to make assertions on actual JSONSchemaProps.
* @param actual the JSONSchemaProps we want to make assertions on.
*/
protected AbstractJSONSchemaPropsAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Navigates to the property additionalItems so that assertions can be done on it
*/
public JSONSchemaPropsOrBoolAssert additionalItems() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (JSONSchemaPropsOrBoolAssert) assertThat(actual.getAdditionalItems()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "additionalItems"));
}
/**
* Navigates to the property additionalProperties so that assertions can be done on it
*/
public JSONSchemaPropsOrBoolAssert additionalProperties() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (JSONSchemaPropsOrBoolAssert) assertThat(actual.getAdditionalProperties()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "additionalProperties"));
}
/**
* Navigates to the property allOf so that assertions can be done on it
*/
public NavigationListAssert allOf() {
isNotNull();
// return the assertion for the property
AssertFactory assertFactory = new AssertFactory() {
public JSONSchemaPropsAssert createAssert(JSONSchemaProps t) {
return (JSONSchemaPropsAssert) assertThat(t);
}
};
NavigationListAssert answer = new NavigationListAssert(actual.getAllOf(), assertFactory);
answer.describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "allOf"));
return answer;
}
/**
* Navigates to the property anyOf so that assertions can be done on it
*/
public NavigationListAssert anyOf() {
isNotNull();
// return the assertion for the property
AssertFactory assertFactory = new AssertFactory() {
public JSONSchemaPropsAssert createAssert(JSONSchemaProps t) {
return (JSONSchemaPropsAssert) assertThat(t);
}
};
NavigationListAssert answer = new NavigationListAssert(actual.getAnyOf(), assertFactory);
answer.describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "anyOf"));
return answer;
}
/**
* Navigates to the property default so that assertions can be done on it
*/
public JSONAssert expectedDefault() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (JSONAssert) assertThat(actual.getDefault()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "default"));
}
/**
* Navigates to the property definitions so that assertions can be done on it
*/
public org.assertj.core.api.MapAssert definitions() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.MapAssert) assertThat(actual.getDefinitions()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "definitions"));
}
/**
* Navigates to the property dependencies so that assertions can be done on it
*/
public org.assertj.core.api.MapAssert dependencies() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.MapAssert) assertThat(actual.getDependencies()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "dependencies"));
}
/**
* Navigates to the property description so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert description() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getDescription()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "description"));
}
/**
* Navigates to the property enum so that assertions can be done on it
*/
public NavigationListAssert expectedEnum() {
isNotNull();
// return the assertion for the property
AssertFactory assertFactory = new AssertFactory() {
public org.assertj.core.api.StringAssert createAssert(String t) {
return (org.assertj.core.api.StringAssert) assertThat(t);
}
};
NavigationListAssert answer = new NavigationListAssert(actual.getEnum(), assertFactory);
answer.describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "enum"));
return answer;
}
/**
* Navigates to the property example so that assertions can be done on it
*/
public JSONAssert example() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (JSONAssert) assertThat(actual.getExample()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "example"));
}
/**
* Navigates to the property exclusiveMaximum so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert exclusiveMaximum() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getExclusiveMaximum()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "exclusiveMaximum"));
}
/**
* Navigates to the property exclusiveMinimum so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert exclusiveMinimum() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getExclusiveMinimum()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "exclusiveMinimum"));
}
/**
* Navigates to the property externalDocs so that assertions can be done on it
*/
public ExternalDocumentationAssert externalDocs() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (ExternalDocumentationAssert) assertThat(actual.getExternalDocs()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "externalDocs"));
}
/**
* Navigates to the property format so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert format() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getFormat()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "format"));
}
/**
* Navigates to the property id so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert id() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getId()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "id"));
}
/**
* Navigates to the property items so that assertions can be done on it
*/
public JSONSchemaPropsOrArrayAssert items() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (JSONSchemaPropsOrArrayAssert) assertThat(actual.getItems()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "items"));
}
/**
* Verifies that the actual JSONSchemaProps's maxItems is equal to the given one.
* @param maxItems the given maxItems to compare the actual JSONSchemaProps's maxItems to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's maxItems is not equal to the given one.
*/
public S hasMaxItems(Long maxItems) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting maxItems of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Long actualMaxItems = actual.getMaxItems();
if (!Objects.areEqual(actualMaxItems, maxItems)) {
failWithMessage(assertjErrorMessage, actual, maxItems, actualMaxItems);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's maxLength is equal to the given one.
* @param maxLength the given maxLength to compare the actual JSONSchemaProps's maxLength to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's maxLength is not equal to the given one.
*/
public S hasMaxLength(Long maxLength) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting maxLength of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Long actualMaxLength = actual.getMaxLength();
if (!Objects.areEqual(actualMaxLength, maxLength)) {
failWithMessage(assertjErrorMessage, actual, maxLength, actualMaxLength);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's maxProperties is equal to the given one.
* @param maxProperties the given maxProperties to compare the actual JSONSchemaProps's maxProperties to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's maxProperties is not equal to the given one.
*/
public S hasMaxProperties(Long maxProperties) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting maxProperties of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Long actualMaxProperties = actual.getMaxProperties();
if (!Objects.areEqual(actualMaxProperties, maxProperties)) {
failWithMessage(assertjErrorMessage, actual, maxProperties, actualMaxProperties);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's maximum is equal to the given one.
* @param maximum the given maximum to compare the actual JSONSchemaProps's maximum to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's maximum is not equal to the given one.
*/
public S hasMaximum(Double maximum) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting maximum of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Double actualMaximum = actual.getMaximum();
if (!Objects.areEqual(actualMaximum, maximum)) {
failWithMessage(assertjErrorMessage, actual, maximum, actualMaximum);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's maximum is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param maximum the value to compare the actual JSONSchemaProps's maximum to.
* @param offset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's maximum is not close enough to the given value.
*/
public S hasMaximumCloseTo(Double maximum, Double offset) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
Double actualMaximum = actual.getMaximum();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting maximum:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualMaximum, maximum, offset, Math.abs(maximum - actualMaximum));
// check
Assertions.assertThat(actualMaximum).overridingErrorMessage(assertjErrorMessage).isCloseTo(maximum, Assertions.within(offset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's minItems is equal to the given one.
* @param minItems the given minItems to compare the actual JSONSchemaProps's minItems to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's minItems is not equal to the given one.
*/
public S hasMinItems(Long minItems) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minItems of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Long actualMinItems = actual.getMinItems();
if (!Objects.areEqual(actualMinItems, minItems)) {
failWithMessage(assertjErrorMessage, actual, minItems, actualMinItems);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's minLength is equal to the given one.
* @param minLength the given minLength to compare the actual JSONSchemaProps's minLength to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's minLength is not equal to the given one.
*/
public S hasMinLength(Long minLength) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minLength of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Long actualMinLength = actual.getMinLength();
if (!Objects.areEqual(actualMinLength, minLength)) {
failWithMessage(assertjErrorMessage, actual, minLength, actualMinLength);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's minProperties is equal to the given one.
* @param minProperties the given minProperties to compare the actual JSONSchemaProps's minProperties to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's minProperties is not equal to the given one.
*/
public S hasMinProperties(Long minProperties) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minProperties of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Long actualMinProperties = actual.getMinProperties();
if (!Objects.areEqual(actualMinProperties, minProperties)) {
failWithMessage(assertjErrorMessage, actual, minProperties, actualMinProperties);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's minimum is equal to the given one.
* @param minimum the given minimum to compare the actual JSONSchemaProps's minimum to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's minimum is not equal to the given one.
*/
public S hasMinimum(Double minimum) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minimum of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Double actualMinimum = actual.getMinimum();
if (!Objects.areEqual(actualMinimum, minimum)) {
failWithMessage(assertjErrorMessage, actual, minimum, actualMinimum);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's minimum is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param minimum the value to compare the actual JSONSchemaProps's minimum to.
* @param offset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's minimum is not close enough to the given value.
*/
public S hasMinimumCloseTo(Double minimum, Double offset) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
Double actualMinimum = actual.getMinimum();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting minimum:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualMinimum, minimum, offset, Math.abs(minimum - actualMinimum));
// check
Assertions.assertThat(actualMinimum).overridingErrorMessage(assertjErrorMessage).isCloseTo(minimum, Assertions.within(offset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's multipleOf is equal to the given one.
* @param multipleOf the given multipleOf to compare the actual JSONSchemaProps's multipleOf to.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's multipleOf is not equal to the given one.
*/
public S hasMultipleOf(Double multipleOf) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting multipleOf of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Double actualMultipleOf = actual.getMultipleOf();
if (!Objects.areEqual(actualMultipleOf, multipleOf)) {
failWithMessage(assertjErrorMessage, actual, multipleOf, actualMultipleOf);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual JSONSchemaProps's multipleOf is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param multipleOf the value to compare the actual JSONSchemaProps's multipleOf to.
* @param offset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual JSONSchemaProps's multipleOf is not close enough to the given value.
*/
public S hasMultipleOfCloseTo(Double multipleOf, Double offset) {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
Double actualMultipleOf = actual.getMultipleOf();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting multipleOf:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualMultipleOf, multipleOf, offset, Math.abs(multipleOf - actualMultipleOf));
// check
Assertions.assertThat(actualMultipleOf).overridingErrorMessage(assertjErrorMessage).isCloseTo(multipleOf, Assertions.within(offset));
// return the current assertion for method chaining
return myself;
}
/**
* Navigates to the property not so that assertions can be done on it
*/
public JSONSchemaPropsAssert not() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (JSONSchemaPropsAssert) assertThat(actual.getNot()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "not"));
}
/**
* Navigates to the property nullable so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert nullable() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getNullable()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "nullable"));
}
/**
* Navigates to the property oneOf so that assertions can be done on it
*/
public NavigationListAssert oneOf() {
isNotNull();
// return the assertion for the property
AssertFactory assertFactory = new AssertFactory() {
public JSONSchemaPropsAssert createAssert(JSONSchemaProps t) {
return (JSONSchemaPropsAssert) assertThat(t);
}
};
NavigationListAssert answer = new NavigationListAssert(actual.getOneOf(), assertFactory);
answer.describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "oneOf"));
return answer;
}
/**
* Navigates to the property pattern so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert pattern() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getPattern()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "pattern"));
}
/**
* Navigates to the property patternProperties so that assertions can be done on it
*/
public org.assertj.core.api.MapAssert patternProperties() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.MapAssert) assertThat(actual.getPatternProperties()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "patternProperties"));
}
/**
* Navigates to the property properties so that assertions can be done on it
*/
public org.assertj.core.api.MapAssert properties() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.MapAssert) assertThat(actual.getProperties()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "properties"));
}
/**
* Navigates to the property required so that assertions can be done on it
*/
public NavigationListAssert required() {
isNotNull();
// return the assertion for the property
AssertFactory assertFactory = new AssertFactory() {
public org.assertj.core.api.StringAssert createAssert(String t) {
return (org.assertj.core.api.StringAssert) assertThat(t);
}
};
NavigationListAssert answer = new NavigationListAssert(actual.getRequired(), assertFactory);
answer.describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "required"));
return answer;
}
/**
* Navigates to the property title so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert title() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getTitle()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "title"));
}
/**
* Navigates to the property type so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert type() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getType()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "type"));
}
/**
* Navigates to the property uniqueItems so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert uniqueItems() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getUniqueItems()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "uniqueItems"));
}
/**
* Navigates to the property xKubernetesEmbeddedResource so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert xKubernetesEmbeddedResource() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getXKubernetesEmbeddedResource()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "xKubernetesEmbeddedResource"));
}
/**
* Navigates to the property xKubernetesIntOrString so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert xKubernetesIntOrString() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getXKubernetesIntOrString()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "xKubernetesIntOrString"));
}
/**
* Navigates to the property xKubernetesListMapKeys so that assertions can be done on it
*/
public NavigationListAssert xKubernetesListMapKeys() {
isNotNull();
// return the assertion for the property
AssertFactory assertFactory = new AssertFactory() {
public org.assertj.core.api.StringAssert createAssert(String t) {
return (org.assertj.core.api.StringAssert) assertThat(t);
}
};
NavigationListAssert answer = new NavigationListAssert(actual.getXKubernetesListMapKeys(), assertFactory);
answer.describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "xKubernetesListMapKeys"));
return answer;
}
/**
* Navigates to the property xKubernetesListType so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert xKubernetesListType() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getXKubernetesListType()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "xKubernetesListType"));
}
/**
* Navigates to the property xKubernetesMapType so that assertions can be done on it
*/
public org.assertj.core.api.StringAssert xKubernetesMapType() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.StringAssert) assertThat(actual.getXKubernetesMapType()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "xKubernetesMapType"));
}
/**
* Navigates to the property xKubernetesPreserveUnknownFields so that assertions can be done on it
*/
public org.assertj.core.api.BooleanAssert xKubernetesPreserveUnknownFields() {
// check that actual JSONSchemaProps we want to make assertions on is not null.
isNotNull();
// return the assertion for the property
return (org.assertj.core.api.BooleanAssert) assertThat(actual.getXKubernetesPreserveUnknownFields()).describedAs(io.fabric8.kubernetes.assertions.Assertions.joinDescription(this, "xKubernetesPreserveUnknownFields"));
}
}