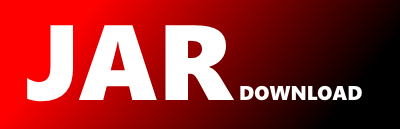
io.fabric8.kubernetes.client.Client Maven / Gradle / Ivy
/*
* Copyright (C) 2015 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.fabric8.kubernetes.client;
import io.fabric8.kubernetes.api.model.APIGroup;
import io.fabric8.kubernetes.api.model.APIGroupList;
import io.fabric8.kubernetes.api.model.APIResourceList;
import io.fabric8.kubernetes.api.model.APIVersions;
import io.fabric8.kubernetes.api.model.HasMetadata;
import io.fabric8.kubernetes.api.model.KubernetesResource;
import io.fabric8.kubernetes.api.model.KubernetesResourceList;
import io.fabric8.kubernetes.api.model.RootPaths;
import io.fabric8.kubernetes.client.dsl.MixedOperation;
import io.fabric8.kubernetes.client.dsl.Resource;
import io.fabric8.kubernetes.client.http.HttpClient;
import java.io.Closeable;
import java.net.URL;
public interface Client extends Closeable {
/**
* Checks the Kubernetes server for support for the given KubernetesResource type.
*
*
* The response is not cached, a new check will be performed for each method invocation. In case custom resource
* definition is installed in between invocations, this method might return different values.
*
* @param type to check for support
* @return boolean value indicating whether this type is supported
*/
boolean supports(Class type);
/**
* Checks the Kubernetes server for support for the given type.
*
*
* The response is not cached, a new check will be performed for each method invocation. In case custom resource
* definition is installed in between invocations, this method might return different values.
*
* @param apiVersion the api/version. This should be fully qualified - that is for openshift, please include the api.
* @param kind the resource kind
* @return boolean value indicating whether this type is supported
*/
boolean supports(String apiVersion, String kind);
/**
* Checks for the api group. exact = false will scan all groups
* for a suffix match. exact = true will look only for that apiGroup.
*
* @param apiGroup to check for
* @param exact true for an exact match
* @return true if there is a match
*/
boolean hasApiGroup(String apiGroup, boolean exact);
/**
* Adapt the client to another type. This will not perform any check of whether the new client
* type is supported. It may even return the same object if it already supports the given
* client type.
*
* @param type the instance of {@link Client} to adapt.
* @return The refined instance of the {@link Client}.
*/
C adapt(Class type);
URL getMasterUrl();
String getApiVersion();
String getNamespace();
RootPaths rootPaths();
/**
* Returns true if this cluster supports the given API path or API Group ID
*
* @param path Path as string
* @return returns boolean value indicating whether it supports.
* @deprecated use {@link #supports(Class)} instead
*/
@Deprecated
boolean supportsApiPath(String path);
@Override
void close();
/**
* Get the available APIversions. APIVersions lists the versions that are available,
* to allow clients to discover the API at /api, which is the root path of the
* legacy v1 API.
*
* @return the {@link APIVersions} object
*/
APIVersions getAPIVersions();
/**
* Returns the api groups. This does not include the core/legacy v1 apiVersion.
*
* @return the {@link APIGroupList} metadata
*/
APIGroupList getApiGroups();
/**
* Return a single api group
*
* @param name of the group
* @return the {@link APIGroup} metadata
*/
APIGroup getApiGroup(String name);
/**
* Return the api resource metadata for the given groupVersion
*
* Use v1 to indicate the core/legacy resources
*
* @param groupVersion the groupVersion - group/version
* @return the {@link APIResourceList} for the groupVersion
*/
APIResourceList getApiResources(String groupVersion);
/**
* Typed API for managing resources. Any properly annotated POJO can be utilized as a resource.
*
* Note: this call is generally for use internally within the DSL, not by end users
*
*
* Note: your resource POJO (T in this context) must implement
* {@link io.fabric8.kubernetes.api.model.Namespaced} if it is a namespace-scoped resource.
*
*
* @param resourceType Class for resource
* @param represents resource type. If it's a namespaced resource, it must implement
* {@link io.fabric8.kubernetes.api.model.Namespaced}
* @param represents resource list type
* @param represents the Resource operation type
* @return returns a MixedOperation object with which you can do basic resource operations. If the class is a known type the
* dsl operation logic will be used.
*/
, R extends Resource> MixedOperation resources(
Class resourceType, Class listClass, Class resourceClass);
/**
* Typed API for managing resources. Any properly annotated POJO can be utilized as a resource.
*
*
* Note: your resource POJO (T in this context) must implement
* {@link io.fabric8.kubernetes.api.model.Namespaced} if it is a namespace-scoped resource.
*
*
* @param resourceType Class for resource
* @param represents resource type. If it's a namespaced resource, it must implement
* {@link io.fabric8.kubernetes.api.model.Namespaced}
* @param represents resource list type
* @return returns a MixedOperation object with which you can do basic resource operations. If the class is a known type the
* dsl operation logic will be used.
*/
default > MixedOperation> resources(
Class resourceType, Class listClass) {
return resources(resourceType, listClass, null);
}
/**
* Creates a new client based upon the current except with a different
* {@link RequestConfig}. It uses the same resources as the current client, thus
* closing it will close the original client.
*
* @param requestConfig
* @return a new client
*/
Client newClient(RequestConfig requestConfig);
HttpClient getHttpClient();
Config getConfiguration();
/**
* GET the response from the given uri as a String
*
* @param uri must start with / if relative
* @return the response, or null if a 404 code
*/
default String raw(String uri) {
return raw(uri, "GET", null);
}
/**
* The response from the given uri as a String
*
* @param uri must start with / if relative
* @param method an http method verb such as GET, DELETE, PUT, POST
* @param payload a non-String value will be converted to json
* @return the response
*/
String raw(String uri, String method, Object payload);
}