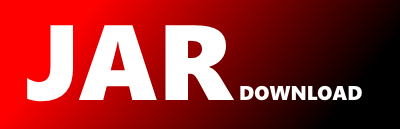
io.fabric8.kubernetes.client.informers.SharedIndexInformer Maven / Gradle / Ivy
/*
* Copyright (C) 2015 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.fabric8.kubernetes.client.informers;
import io.fabric8.kubernetes.client.WatcherException;
import io.fabric8.kubernetes.client.informers.cache.Cache;
import io.fabric8.kubernetes.client.informers.cache.Indexer;
import io.fabric8.kubernetes.client.informers.cache.ItemStore;
import io.fabric8.kubernetes.client.informers.cache.Store;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
import java.util.function.Function;
import java.util.stream.Stream;
/**
* SharedInxedInformer extends SharedInformer and provides indexer operability additionally.
*/
public interface SharedIndexInformer extends AutoCloseable {
/**
* Add indexers
*
* @param indexers indexers
*/
SharedIndexInformer addIndexers(Map>> indexers);
/**
* Remove the namesapce index
*
* @return this
*/
default SharedIndexInformer removeNamespaceIndex() {
return removeIndexer(Cache.NAMESPACE_INDEX);
}
/**
* Remove the named index
*
* @param name
* @return this
*/
SharedIndexInformer removeIndexer(String name);
/**
* returns the internal indexer store.
*
* @return the internal indexer store
*/
Indexer getIndexer();
/**
* Add event handler.
*
* The handler methods will be called using the client's {@link Executor}
*
* @param handler event handler
*/
SharedIndexInformer addEventHandler(ResourceEventHandler super T> handler);
/**
* Remove event handler.
*
* @param handler event handler
*/
SharedIndexInformer removeEventHandler(ResourceEventHandler super T> handler);
/**
* Adds an event handler to the shared informer using the specified resync period.
* Events to a single handler are delivered sequentially, but there is no
* coordination between different handlers.
*
* The handler methods will be called using the client's {@link Executor}
*
* @param handle the event handler
* @param resyncPeriod the specific resync period
*/
SharedIndexInformer addEventHandlerWithResyncPeriod(ResourceEventHandler super T> handle,
long resyncPeriod);
/**
* Starts the shared informer, which will be stopped when {@link #stop()} is called.
*
*
* Only one start attempt is made - subsequent calls will not re-start the informer.
*
*
* If the informer is not already running, this is a blocking call
*/
SharedIndexInformer run();
/**
* Stops the shared informer. The informer cannot be started again.
*
* Once this call completes the informer will stop processing events, but the underlying watch closure may not yet be
* completed
*/
void stop();
@Override
default void close() {
stop();
}
/**
* Return true if the informer has ever synced
*/
default boolean hasSynced() {
return lastSyncResourceVersion() != null;
}
/**
* The resource version observed when last synced with the underlying store.
* The value returned is not synchronized with access to the underlying store
* and is not thread-safe.
*
* Since the store processes events asynchronously this value should not be
* used as an indication of the last resourceVersion seen. Also after an
* informer is stopped any pending event processing may not happen.
*
* @return string value or null if never synced
*/
String lastSyncResourceVersion();
/**
* Return true if the informer is running
*
* See also {@link #stopped()}
*/
boolean isRunning();
/**
* Return the class this informer is watching
*/
Class getApiTypeClass();
/**
* Return true if the informer is actively watching
*
* Will return false when {@link #isRunning()} is true when the watch needs to be re-established.
*/
boolean isWatching();
/**
* Return the Store associated with this informer
*
* @return the store
*/
Store getStore();
/**
* Sets the initial state of the informer store, which will
* be replaced by the initial list operation. This will emit
* relevant delete and update events, rather than just adds.
*
* Can only be called before the informer is running
*
* @param items
*/
SharedIndexInformer initialState(Stream items);
SharedIndexInformer itemStore(ItemStore itemStore);
/**
* A non-blocking alternative to run. Starts the shared informer, which will normally be stopped when {@link #stop()} is
* called.
*
* The stage will be completed normally once the Informer starts watching successfully for the first time.
*
* By default the informer will attempt only a single start attempt. Use {@link #exceptionHandler(ExceptionHandler)} to
* modify this behavior.
*/
CompletionStage start();
/**
* Sets the {@link ExceptionHandler} for this informer. For example, exceptionHandler((b, t) -> true)), will
* keep retrying no matter what the exception is.
*
* May only be called prior to the informer starting
*
* @param handler
*/
SharedIndexInformer exceptionHandler(ExceptionHandler handler);
/**
* Return a {@link CompletionStage} that will allow notification of the informer stopping. This will be completed after
* event processing has stopped.
*
* If {@link #stop()} is called, the CompletionStage will complete normally.
*
* If an exception occurs that terminates the informer, then it will be exceptionally completed with that exception
* - typically a {@link WatcherException}
*/
CompletionStage stopped();
}