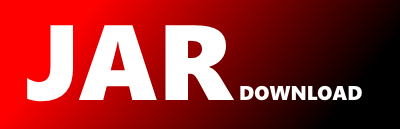
io.fabric8.kubernetes.api.model.KubeSchemaBuilder Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.Object;
import java.lang.Boolean;
public class KubeSchemaBuilder extends KubeSchemaFluentImpl implements VisitableBuilder{
KubeSchemaFluent> fluent;
Boolean validationEnabled;
public KubeSchemaBuilder(){
this(true);
}
public KubeSchemaBuilder(Boolean validationEnabled){
this(new KubeSchema(), validationEnabled);
}
public KubeSchemaBuilder(KubeSchemaFluent> fluent){
this(fluent, true);
}
public KubeSchemaBuilder(KubeSchemaFluent> fluent,Boolean validationEnabled){
this(fluent, new KubeSchema(), validationEnabled);
}
public KubeSchemaBuilder(KubeSchemaFluent> fluent,KubeSchema instance){
this(fluent, instance, true);
}
public KubeSchemaBuilder(KubeSchemaFluent> fluent,KubeSchema instance,Boolean validationEnabled){
this.fluent = fluent;
fluent.withAPIGroup(instance.getAPIGroup());
fluent.withAPIGroupList(instance.getAPIGroupList());
fluent.withBaseKubernetesList(instance.getBaseKubernetesList());
fluent.withBinding(instance.getBinding());
fluent.withComponentStatus(instance.getComponentStatus());
fluent.withComponentStatusList(instance.getComponentStatusList());
fluent.withConfig(instance.getConfig());
fluent.withConfigMap(instance.getConfigMap());
fluent.withConfigMapList(instance.getConfigMapList());
fluent.withContainerStatus(instance.getContainerStatus());
fluent.withCreateOptions(instance.getCreateOptions());
fluent.withDeleteOptions(instance.getDeleteOptions());
fluent.withEndpointPort(instance.getEndpointPort());
fluent.withEndpoints(instance.getEndpoints());
fluent.withEndpointsList(instance.getEndpointsList());
fluent.withEnvVar(instance.getEnvVar());
fluent.withEventSource(instance.getEventSource());
fluent.withGetOptions(instance.getGetOptions());
fluent.withGroupVersionKind(instance.getGroupVersionKind());
fluent.withGroupVersionResource(instance.getGroupVersionResource());
fluent.withInfo(instance.getInfo());
fluent.withLimitRangeList(instance.getLimitRangeList());
fluent.withListOptions(instance.getListOptions());
fluent.withMicroTime(instance.getMicroTime());
fluent.withNamespace(instance.getNamespace());
fluent.withNamespaceList(instance.getNamespaceList());
fluent.withNode(instance.getNode());
fluent.withNodeList(instance.getNodeList());
fluent.withObjectMeta(instance.getObjectMeta());
fluent.withPatch(instance.getPatch());
fluent.withPatchOptions(instance.getPatchOptions());
fluent.withPersistentVolume(instance.getPersistentVolume());
fluent.withPersistentVolumeClaim(instance.getPersistentVolumeClaim());
fluent.withPersistentVolumeClaimList(instance.getPersistentVolumeClaimList());
fluent.withPersistentVolumeList(instance.getPersistentVolumeList());
fluent.withPodList(instance.getPodList());
fluent.withPodTemplateList(instance.getPodTemplateList());
fluent.withQuantity(instance.getQuantity());
fluent.withReplicationControllerList(instance.getReplicationControllerList());
fluent.withResourceQuota(instance.getResourceQuota());
fluent.withResourceQuotaList(instance.getResourceQuotaList());
fluent.withRootPaths(instance.getRootPaths());
fluent.withSecret(instance.getSecret());
fluent.withSecretList(instance.getSecretList());
fluent.withServiceAccount(instance.getServiceAccount());
fluent.withServiceAccountList(instance.getServiceAccountList());
fluent.withServiceList(instance.getServiceList());
fluent.withStatus(instance.getStatus());
fluent.withTime(instance.getTime());
fluent.withToleration(instance.getToleration());
fluent.withTopologySelectorTerm(instance.getTopologySelectorTerm());
fluent.withTypeMeta(instance.getTypeMeta());
fluent.withUpdateOptions(instance.getUpdateOptions());
fluent.withWatchEvent(instance.getWatchEvent());
this.validationEnabled = validationEnabled;
}
public KubeSchemaBuilder(KubeSchema instance){
this(instance,true);
}
public KubeSchemaBuilder(KubeSchema instance,Boolean validationEnabled){
this.fluent = this;
this.withAPIGroup(instance.getAPIGroup());
this.withAPIGroupList(instance.getAPIGroupList());
this.withBaseKubernetesList(instance.getBaseKubernetesList());
this.withBinding(instance.getBinding());
this.withComponentStatus(instance.getComponentStatus());
this.withComponentStatusList(instance.getComponentStatusList());
this.withConfig(instance.getConfig());
this.withConfigMap(instance.getConfigMap());
this.withConfigMapList(instance.getConfigMapList());
this.withContainerStatus(instance.getContainerStatus());
this.withCreateOptions(instance.getCreateOptions());
this.withDeleteOptions(instance.getDeleteOptions());
this.withEndpointPort(instance.getEndpointPort());
this.withEndpoints(instance.getEndpoints());
this.withEndpointsList(instance.getEndpointsList());
this.withEnvVar(instance.getEnvVar());
this.withEventSource(instance.getEventSource());
this.withGetOptions(instance.getGetOptions());
this.withGroupVersionKind(instance.getGroupVersionKind());
this.withGroupVersionResource(instance.getGroupVersionResource());
this.withInfo(instance.getInfo());
this.withLimitRangeList(instance.getLimitRangeList());
this.withListOptions(instance.getListOptions());
this.withMicroTime(instance.getMicroTime());
this.withNamespace(instance.getNamespace());
this.withNamespaceList(instance.getNamespaceList());
this.withNode(instance.getNode());
this.withNodeList(instance.getNodeList());
this.withObjectMeta(instance.getObjectMeta());
this.withPatch(instance.getPatch());
this.withPatchOptions(instance.getPatchOptions());
this.withPersistentVolume(instance.getPersistentVolume());
this.withPersistentVolumeClaim(instance.getPersistentVolumeClaim());
this.withPersistentVolumeClaimList(instance.getPersistentVolumeClaimList());
this.withPersistentVolumeList(instance.getPersistentVolumeList());
this.withPodList(instance.getPodList());
this.withPodTemplateList(instance.getPodTemplateList());
this.withQuantity(instance.getQuantity());
this.withReplicationControllerList(instance.getReplicationControllerList());
this.withResourceQuota(instance.getResourceQuota());
this.withResourceQuotaList(instance.getResourceQuotaList());
this.withRootPaths(instance.getRootPaths());
this.withSecret(instance.getSecret());
this.withSecretList(instance.getSecretList());
this.withServiceAccount(instance.getServiceAccount());
this.withServiceAccountList(instance.getServiceAccountList());
this.withServiceList(instance.getServiceList());
this.withStatus(instance.getStatus());
this.withTime(instance.getTime());
this.withToleration(instance.getToleration());
this.withTopologySelectorTerm(instance.getTopologySelectorTerm());
this.withTypeMeta(instance.getTypeMeta());
this.withUpdateOptions(instance.getUpdateOptions());
this.withWatchEvent(instance.getWatchEvent());
this.validationEnabled = validationEnabled;
}
public KubeSchema build(){
KubeSchema buildable = new KubeSchema(fluent.getAPIGroup(),fluent.getAPIGroupList(),fluent.getBaseKubernetesList(),fluent.getBinding(),fluent.getComponentStatus(),fluent.getComponentStatusList(),fluent.getConfig(),fluent.getConfigMap(),fluent.getConfigMapList(),fluent.getContainerStatus(),fluent.getCreateOptions(),fluent.getDeleteOptions(),fluent.getEndpointPort(),fluent.getEndpoints(),fluent.getEndpointsList(),fluent.getEnvVar(),fluent.getEventSource(),fluent.getGetOptions(),fluent.getGroupVersionKind(),fluent.getGroupVersionResource(),fluent.getInfo(),fluent.getLimitRangeList(),fluent.getListOptions(),fluent.getMicroTime(),fluent.getNamespace(),fluent.getNamespaceList(),fluent.getNode(),fluent.getNodeList(),fluent.getObjectMeta(),fluent.getPatch(),fluent.getPatchOptions(),fluent.getPersistentVolume(),fluent.getPersistentVolumeClaim(),fluent.getPersistentVolumeClaimList(),fluent.getPersistentVolumeList(),fluent.getPodList(),fluent.getPodTemplateList(),fluent.getQuantity(),fluent.getReplicationControllerList(),fluent.getResourceQuota(),fluent.getResourceQuotaList(),fluent.getRootPaths(),fluent.getSecret(),fluent.getSecretList(),fluent.getServiceAccount(),fluent.getServiceAccountList(),fluent.getServiceList(),fluent.getStatus(),fluent.getTime(),fluent.getToleration(),fluent.getTopologySelectorTerm(),fluent.getTypeMeta(),fluent.getUpdateOptions(),fluent.getWatchEvent());
return buildable;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
KubeSchemaBuilder that = (KubeSchemaBuilder) o;
if (fluent != null &&fluent != this ? !fluent.equals(that.fluent) :that.fluent != null &&fluent != this ) return false;
if (validationEnabled != null ? !validationEnabled.equals(that.validationEnabled) :that.validationEnabled != null) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy