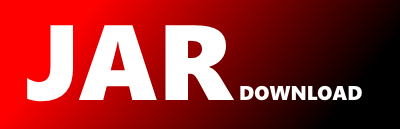
io.fabric8.kubernetes.api.model.ManagedFieldsEntryFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import java.lang.StringBuffer;
import java.lang.Deprecated;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
import java.lang.Boolean;
public class ManagedFieldsEntryFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements ManagedFieldsEntryFluent{
private String apiVersion;
private String fieldsType;
private FieldsV1Builder fieldsV1;
private String manager;
private String operation;
private String time;
public ManagedFieldsEntryFluentImpl(){
}
public ManagedFieldsEntryFluentImpl(ManagedFieldsEntry instance){
this.withApiVersion(instance.getApiVersion());
this.withFieldsType(instance.getFieldsType());
this.withFieldsV1(instance.getFieldsV1());
this.withManager(instance.getManager());
this.withOperation(instance.getOperation());
this.withTime(instance.getTime());
}
public String getApiVersion(){
return this.apiVersion;
}
public A withApiVersion(String apiVersion){
this.apiVersion=apiVersion; return (A) this;
}
public Boolean hasApiVersion(){
return this.apiVersion != null;
}
public A withNewApiVersion(String arg1){
return (A)withApiVersion(new String(arg1));
}
public A withNewApiVersion(StringBuilder arg1){
return (A)withApiVersion(new String(arg1));
}
public A withNewApiVersion(StringBuffer arg1){
return (A)withApiVersion(new String(arg1));
}
public String getFieldsType(){
return this.fieldsType;
}
public A withFieldsType(String fieldsType){
this.fieldsType=fieldsType; return (A) this;
}
public Boolean hasFieldsType(){
return this.fieldsType != null;
}
public A withNewFieldsType(String arg1){
return (A)withFieldsType(new String(arg1));
}
public A withNewFieldsType(StringBuilder arg1){
return (A)withFieldsType(new String(arg1));
}
public A withNewFieldsType(StringBuffer arg1){
return (A)withFieldsType(new String(arg1));
}
/**
* This method has been deprecated, please use method buildFieldsV1 instead.
* @return The buildable object.
*/
@Deprecated public FieldsV1 getFieldsV1(){
return this.fieldsV1!=null?this.fieldsV1.build():null;
}
public FieldsV1 buildFieldsV1(){
return this.fieldsV1!=null?this.fieldsV1.build():null;
}
public A withFieldsV1(FieldsV1 fieldsV1){
_visitables.get("fieldsV1").remove(this.fieldsV1);
if (fieldsV1!=null){ this.fieldsV1= new FieldsV1Builder(fieldsV1); _visitables.get("fieldsV1").add(this.fieldsV1);} return (A) this;
}
public Boolean hasFieldsV1(){
return this.fieldsV1 != null;
}
public ManagedFieldsEntryFluent.FieldsV1Nested withNewFieldsV1(){
return new FieldsV1NestedImpl();
}
public ManagedFieldsEntryFluent.FieldsV1Nested withNewFieldsV1Like(FieldsV1 item){
return new FieldsV1NestedImpl(item);
}
public ManagedFieldsEntryFluent.FieldsV1Nested editFieldsV1(){
return withNewFieldsV1Like(getFieldsV1());
}
public ManagedFieldsEntryFluent.FieldsV1Nested editOrNewFieldsV1(){
return withNewFieldsV1Like(getFieldsV1() != null ? getFieldsV1(): new FieldsV1Builder().build());
}
public ManagedFieldsEntryFluent.FieldsV1Nested editOrNewFieldsV1Like(FieldsV1 item){
return withNewFieldsV1Like(getFieldsV1() != null ? getFieldsV1(): item);
}
public String getManager(){
return this.manager;
}
public A withManager(String manager){
this.manager=manager; return (A) this;
}
public Boolean hasManager(){
return this.manager != null;
}
public A withNewManager(String arg1){
return (A)withManager(new String(arg1));
}
public A withNewManager(StringBuilder arg1){
return (A)withManager(new String(arg1));
}
public A withNewManager(StringBuffer arg1){
return (A)withManager(new String(arg1));
}
public String getOperation(){
return this.operation;
}
public A withOperation(String operation){
this.operation=operation; return (A) this;
}
public Boolean hasOperation(){
return this.operation != null;
}
public A withNewOperation(String arg1){
return (A)withOperation(new String(arg1));
}
public A withNewOperation(StringBuilder arg1){
return (A)withOperation(new String(arg1));
}
public A withNewOperation(StringBuffer arg1){
return (A)withOperation(new String(arg1));
}
public String getTime(){
return this.time;
}
public A withTime(String time){
this.time=time; return (A) this;
}
public Boolean hasTime(){
return this.time != null;
}
public A withNewTime(String arg1){
return (A)withTime(new String(arg1));
}
public A withNewTime(StringBuilder arg1){
return (A)withTime(new String(arg1));
}
public A withNewTime(StringBuffer arg1){
return (A)withTime(new String(arg1));
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ManagedFieldsEntryFluentImpl that = (ManagedFieldsEntryFluentImpl) o;
if (apiVersion != null ? !apiVersion.equals(that.apiVersion) :that.apiVersion != null) return false;
if (fieldsType != null ? !fieldsType.equals(that.fieldsType) :that.fieldsType != null) return false;
if (fieldsV1 != null ? !fieldsV1.equals(that.fieldsV1) :that.fieldsV1 != null) return false;
if (manager != null ? !manager.equals(that.manager) :that.manager != null) return false;
if (operation != null ? !operation.equals(that.operation) :that.operation != null) return false;
if (time != null ? !time.equals(that.time) :that.time != null) return false;
return true;
}
public class FieldsV1NestedImpl extends FieldsV1FluentImpl> implements ManagedFieldsEntryFluent.FieldsV1Nested,io.fabric8.kubernetes.api.builder.Nested{
private final FieldsV1Builder builder;
FieldsV1NestedImpl(FieldsV1 item){
this.builder = new FieldsV1Builder(this, item);
}
FieldsV1NestedImpl(){
this.builder = new FieldsV1Builder(this);
}
public N and(){
return (N) ManagedFieldsEntryFluentImpl.this.withFieldsV1(builder.build());
}
public N endFieldsV1(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy