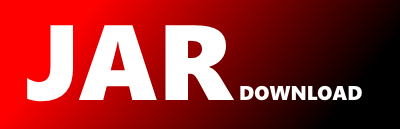
io.fabric8.kubernetes.api.model.NodeConfigStatusFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import java.lang.StringBuffer;
import java.lang.Deprecated;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
import java.lang.Boolean;
public class NodeConfigStatusFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements NodeConfigStatusFluent{
private NodeConfigSourceBuilder active;
private NodeConfigSourceBuilder assigned;
private String error;
private NodeConfigSourceBuilder lastKnownGood;
public NodeConfigStatusFluentImpl(){
}
public NodeConfigStatusFluentImpl(NodeConfigStatus instance){
this.withActive(instance.getActive());
this.withAssigned(instance.getAssigned());
this.withError(instance.getError());
this.withLastKnownGood(instance.getLastKnownGood());
}
/**
* This method has been deprecated, please use method buildActive instead.
* @return The buildable object.
*/
@Deprecated public NodeConfigSource getActive(){
return this.active!=null?this.active.build():null;
}
public NodeConfigSource buildActive(){
return this.active!=null?this.active.build():null;
}
public A withActive(NodeConfigSource active){
_visitables.get("active").remove(this.active);
if (active!=null){ this.active= new NodeConfigSourceBuilder(active); _visitables.get("active").add(this.active);} return (A) this;
}
public Boolean hasActive(){
return this.active != null;
}
public NodeConfigStatusFluent.ActiveNested withNewActive(){
return new ActiveNestedImpl();
}
public NodeConfigStatusFluent.ActiveNested withNewActiveLike(NodeConfigSource item){
return new ActiveNestedImpl(item);
}
public NodeConfigStatusFluent.ActiveNested editActive(){
return withNewActiveLike(getActive());
}
public NodeConfigStatusFluent.ActiveNested editOrNewActive(){
return withNewActiveLike(getActive() != null ? getActive(): new NodeConfigSourceBuilder().build());
}
public NodeConfigStatusFluent.ActiveNested editOrNewActiveLike(NodeConfigSource item){
return withNewActiveLike(getActive() != null ? getActive(): item);
}
/**
* This method has been deprecated, please use method buildAssigned instead.
* @return The buildable object.
*/
@Deprecated public NodeConfigSource getAssigned(){
return this.assigned!=null?this.assigned.build():null;
}
public NodeConfigSource buildAssigned(){
return this.assigned!=null?this.assigned.build():null;
}
public A withAssigned(NodeConfigSource assigned){
_visitables.get("assigned").remove(this.assigned);
if (assigned!=null){ this.assigned= new NodeConfigSourceBuilder(assigned); _visitables.get("assigned").add(this.assigned);} return (A) this;
}
public Boolean hasAssigned(){
return this.assigned != null;
}
public NodeConfigStatusFluent.AssignedNested withNewAssigned(){
return new AssignedNestedImpl();
}
public NodeConfigStatusFluent.AssignedNested withNewAssignedLike(NodeConfigSource item){
return new AssignedNestedImpl(item);
}
public NodeConfigStatusFluent.AssignedNested editAssigned(){
return withNewAssignedLike(getAssigned());
}
public NodeConfigStatusFluent.AssignedNested editOrNewAssigned(){
return withNewAssignedLike(getAssigned() != null ? getAssigned(): new NodeConfigSourceBuilder().build());
}
public NodeConfigStatusFluent.AssignedNested editOrNewAssignedLike(NodeConfigSource item){
return withNewAssignedLike(getAssigned() != null ? getAssigned(): item);
}
public String getError(){
return this.error;
}
public A withError(String error){
this.error=error; return (A) this;
}
public Boolean hasError(){
return this.error != null;
}
public A withNewError(String arg1){
return (A)withError(new String(arg1));
}
public A withNewError(StringBuilder arg1){
return (A)withError(new String(arg1));
}
public A withNewError(StringBuffer arg1){
return (A)withError(new String(arg1));
}
/**
* This method has been deprecated, please use method buildLastKnownGood instead.
* @return The buildable object.
*/
@Deprecated public NodeConfigSource getLastKnownGood(){
return this.lastKnownGood!=null?this.lastKnownGood.build():null;
}
public NodeConfigSource buildLastKnownGood(){
return this.lastKnownGood!=null?this.lastKnownGood.build():null;
}
public A withLastKnownGood(NodeConfigSource lastKnownGood){
_visitables.get("lastKnownGood").remove(this.lastKnownGood);
if (lastKnownGood!=null){ this.lastKnownGood= new NodeConfigSourceBuilder(lastKnownGood); _visitables.get("lastKnownGood").add(this.lastKnownGood);} return (A) this;
}
public Boolean hasLastKnownGood(){
return this.lastKnownGood != null;
}
public NodeConfigStatusFluent.LastKnownGoodNested withNewLastKnownGood(){
return new LastKnownGoodNestedImpl();
}
public NodeConfigStatusFluent.LastKnownGoodNested withNewLastKnownGoodLike(NodeConfigSource item){
return new LastKnownGoodNestedImpl(item);
}
public NodeConfigStatusFluent.LastKnownGoodNested editLastKnownGood(){
return withNewLastKnownGoodLike(getLastKnownGood());
}
public NodeConfigStatusFluent.LastKnownGoodNested editOrNewLastKnownGood(){
return withNewLastKnownGoodLike(getLastKnownGood() != null ? getLastKnownGood(): new NodeConfigSourceBuilder().build());
}
public NodeConfigStatusFluent.LastKnownGoodNested editOrNewLastKnownGoodLike(NodeConfigSource item){
return withNewLastKnownGoodLike(getLastKnownGood() != null ? getLastKnownGood(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
NodeConfigStatusFluentImpl that = (NodeConfigStatusFluentImpl) o;
if (active != null ? !active.equals(that.active) :that.active != null) return false;
if (assigned != null ? !assigned.equals(that.assigned) :that.assigned != null) return false;
if (error != null ? !error.equals(that.error) :that.error != null) return false;
if (lastKnownGood != null ? !lastKnownGood.equals(that.lastKnownGood) :that.lastKnownGood != null) return false;
return true;
}
public class ActiveNestedImpl extends NodeConfigSourceFluentImpl> implements NodeConfigStatusFluent.ActiveNested,io.fabric8.kubernetes.api.builder.Nested{
private final NodeConfigSourceBuilder builder;
ActiveNestedImpl(NodeConfigSource item){
this.builder = new NodeConfigSourceBuilder(this, item);
}
ActiveNestedImpl(){
this.builder = new NodeConfigSourceBuilder(this);
}
public N and(){
return (N) NodeConfigStatusFluentImpl.this.withActive(builder.build());
}
public N endActive(){
return and();
}
}
public class AssignedNestedImpl extends NodeConfigSourceFluentImpl> implements NodeConfigStatusFluent.AssignedNested,io.fabric8.kubernetes.api.builder.Nested{
private final NodeConfigSourceBuilder builder;
AssignedNestedImpl(NodeConfigSource item){
this.builder = new NodeConfigSourceBuilder(this, item);
}
AssignedNestedImpl(){
this.builder = new NodeConfigSourceBuilder(this);
}
public N and(){
return (N) NodeConfigStatusFluentImpl.this.withAssigned(builder.build());
}
public N endAssigned(){
return and();
}
}
public class LastKnownGoodNestedImpl extends NodeConfigSourceFluentImpl> implements NodeConfigStatusFluent.LastKnownGoodNested,io.fabric8.kubernetes.api.builder.Nested{
private final NodeConfigSourceBuilder builder;
LastKnownGoodNestedImpl(NodeConfigSource item){
this.builder = new NodeConfigSourceBuilder(this, item);
}
LastKnownGoodNestedImpl(){
this.builder = new NodeConfigSourceBuilder(this);
}
public N and(){
return (N) NodeConfigStatusFluentImpl.this.withLastKnownGood(builder.build());
}
public N endLastKnownGood(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy